Which of the following is a class in Java
Which of the following is a class in Java
I'm happy to help! However, please note that I'll be responding in English as per your request.
A class in Java is the fundamental building block of object-oriented programming (OOP). It represents a blueprint or a template for creating objects that share common attributes and methods. In other words, a class defines the characteristics and behavior of an object, including its properties and methods.
In Java, a class is declared using the class
keyword followed by the name of the class. For example:
public class Person {
private String name;
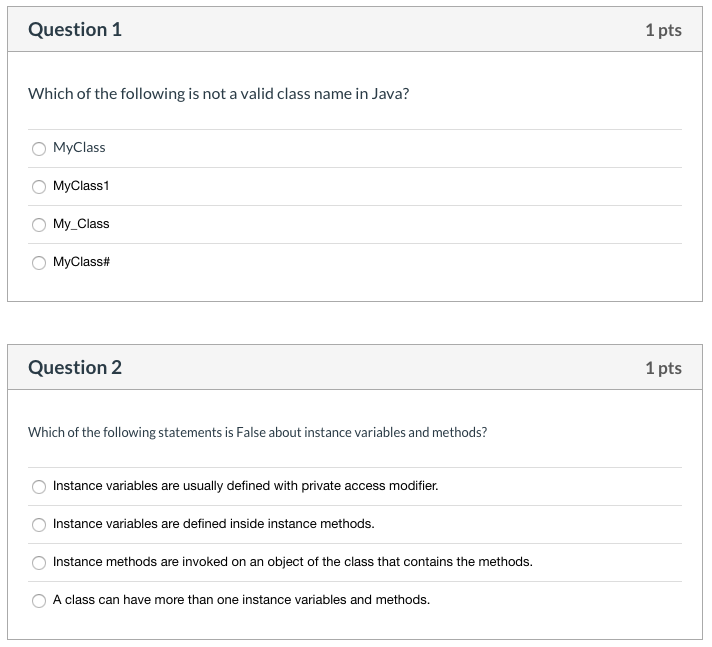
private int age;
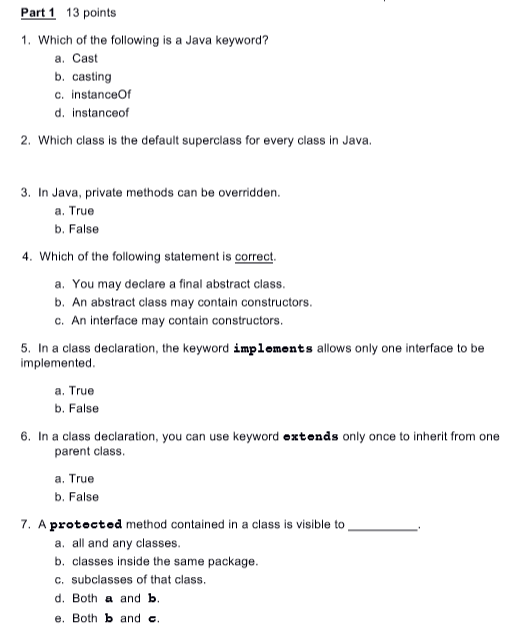
public Person(String name, int age) {
this.name = name;
this.age = age;
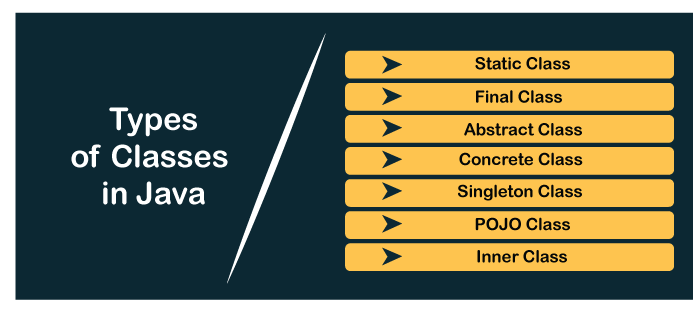
}
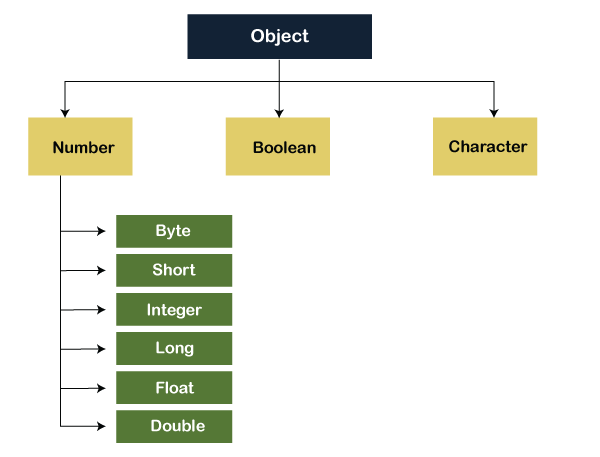
public void printInfo() {
System.out.println("Name: " + name);
System.out.println("Age: " + age);
}
}
In the above example, Person
is a class that has two private properties (attributes): name
and age
. It also has a constructor method (public Person(String name, int age)
), which initializes an object of the Person
class. Furthermore, it has a method called printInfo()
, which prints out the value of the name
and age
properties when called.
Java classes can be classified into three main categories:
Basic Classes: These are the fundamental building blocks of Java programming. They represent objects with attributes (data) and methods (functions). Examples includePerson
, Car
, BankAccount
, etc. Container Classes: These classes are used to store and manipulate collections of objects or primitive data types. Examples include ArrayList
, HashMap
, Vector
, etc. Specialized Classes: These classes are designed to perform specific tasks or operations. Examples include Thread
, TimerTask
, Socket
, etc.
In addition to these categories, Java classes can also be distinguished based on their scope:
Public Class: A public class is accessible from anywhere in the program and can be instantiated using its fully qualified name. Private Class: A private class is only accessible within its own package or file. Protected Class: A protected class is accessible within its own package, but not outside it.In conclusion, a Java class is a fundamental concept that defines an object's characteristics and behavior. It represents the blueprint for creating objects that share common attributes and methods. Understanding classes is crucial to mastering object-oriented programming in Java.
Please let me know if you'd like me to elaborate on any of these points or clarify any specific concepts related to Java classes!
Can there be 2 classes in Java?
I'd be happy to help!
Yes, absolutely! In Java, it is possible to have multiple classes with different purposes and responsibilities. In fact, this is one of the key features of object-oriented programming (OOP) that Java supports.
In Java, a class is essentially a blueprint for creating objects that contain data (attributes or fields) and behavior (methods). When you create a class, you can define its attributes, methods, and constructors to specify how it should behave. Multiple classes can be defined in the same Java program, each with its own unique characteristics.
Here are some examples of why having multiple classes might be beneficial:
Separation of Concerns: In larger programs, it's often helpful to divide the code into smaller, more manageable pieces that focus on specific tasks or responsibilities. Each class can handle a distinct aspect of the program, making it easier to maintain and update. Reusability: Classes can be designed to work together seamlessly, allowing you to create reusable code that can be easily integrated into other parts of your program. This promotes code reuse and reduces duplication. Abstraction: By defining multiple classes, you can abstract away complex logic or dependencies, making it easier for other developers (or yourself) to understand the code at a higher level.To illustrate this concept, let's consider an example: suppose you're building a simple game that involves player characters, enemies, and items. You might define three separate classes:
Player: This class could represent the player character, with attributes like health points, attack strength, and special abilities. Enemy: The Enemy class would define characteristics for each type of enemy in the game (e.g., strength, agility, or magical resistance). Item: Finally, the Item class could be used to create different types of items that players can collect (e.g., health potions, shields, or weapons).Each class would have its own methods and constructors for handling specific aspects of the game. For instance, the Player class might have a method for leveling up, while the Enemy class could have a method for determining when it's time to attack.
By using multiple classes in Java, you can create complex programs with clear, organized code that's easy to understand and maintain. So go ahead and give it a try – you never know what amazing creations you might bring to life!