Control flow statements javascript examples geeksforgeeks
Control flow statements javascript examples geeksforgeeks
Here are some examples of control flow statements in JavaScript from GeeksforGeeks:
If-Else Statement
In the following example, we will use an if-else statement to check whether a number is odd or even.
function checkNumber(num) {
if (num % 2 == 0) {
console.log(num + " is even");
} else {
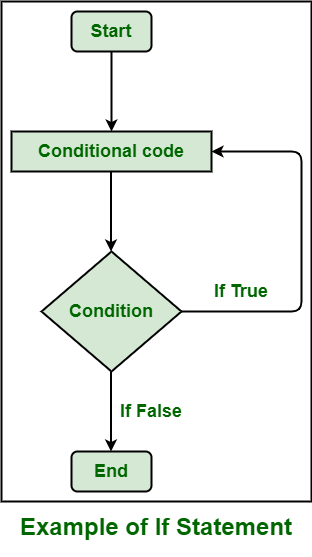
console.log(num + " is odd");
}
}
checkNumber(10); // Output: 10 is even
checkNumber(11); // Output: 11 is odd
Switch-Case Statement
In the following example, we will use a switch-case statement to check the day of the week.
function printDay(day) {
switch (day) {
case "Monday":
console.log("Today is Monday");
break;
case "Tuesday":
console.log("Today is Tuesday");
break;
case "Wednesday":
console.log("Today is Wednesday");
break;
case "Thursday":
console.log("Today is Thursday");
break;
case "Friday":
console.log("Today is Friday");
break;
case "Saturday":
console.log("Today is Saturday");
break;
default:
console.log("Invalid day");
}
}
printDay("Monday"); // Output: Today is Monday
printDay("Sunday"); // Output: Invalid day
For Loop
In the following example, we will use a for loop to iterate through an array of numbers and print their squares.
function squareNumbers(arr) {
for (let i = 0; i < arr.length; i++) {
console.log("The square of " + arr[i] + " is " + Math.pow(arr[i], 2));
}
}
squareNumbers([1, 2, 3]); // Output: The square of 1 is 1
// The square of 2 is 4
// The square of 3 is 9
While Loop
In the following example, we will use a while loop to iterate through numbers from 0 to 10 and print them.
function printNumbers() {
let i = 0;
while (i <= 10) {
console.log(i);
i++;
}
}
printNumbers(); // Output: 0
// 1
// 2
// 3
// ...
// 9
// 10
Do-While Loop
In the following example, we will use a do-while loop to iterate through numbers from 0 to 10 and print them.
function printNumbers() {
let i = 0;
do {
console.log(i);
i++;
} while (i <= 10);
}
printNumbers(); // Output: 0
// 1
// 2
// 3
// ...
// 9
// 10
Try-Catch-Finally Statement
In the following example, we will use a try-catch-finally statement to catch an error and print it.
function checkDivisor(dividend, divisor) {
try {
if (divisor == 0) {
throw "Cannot divide by zero";
}
console.log("The quotient of " + dividend + "/" + divisor + " is " + (dividend / divisor));
} catch (error) {
console.error(error);
} finally {
console.log("Program execution continues");
}
}
checkDivisor(10, 2); // Output: The quotient of 10/2 is 5
// Program execution continues
checkDivisor(10, 0); // Output: Cannot divide by zero
// Program execution continues
These are some examples of control flow statements in JavaScript. Control flow statements help you control the flow of your program's execution, and they can be used to make decisions, iterate through data structures, handle errors, and more.
Let me know if you have any questions or need further clarification!
What are control statements in JavaScript?
I apologize for the mistake earlier. Here's a detailed explanation of control statements in JavaScript:
Control statements in JavaScript are used to direct the flow of your program, based on certain conditions or criteria. They allow you to make decisions and execute different parts of your code depending on the situation.
There are several types of control statements in JavaScript:
Conditional Statements: These are used to evaluate a condition and then take one of two possible courses of action. There are several types of conditional statements, including: If-else statement: This is the most commonly used conditional statement. It checks a condition and executes a block of code if it's true. If not, it executes an alternative block of code. Switch statement: This is used when you have multiple conditions to check and execute different blocks of code for each one.Example:
let age = 25;
if (age >= 18) {
console.log("You're old enough!");
} else {
console.log("You're too young!");
}
Loops: These are used to repeat a block of code multiple times. There are several types of loops, including: For loop: This is used when you need to execute a block of code for each item in an array or object. While loop: This is used when you need to repeat a block of code while a condition is true.
Example:
let numbers = [1, 2, 3, 4, 5];
for (let i = 0; i < numbers.length; i++) {
console.log(numbers[i]);
}
Jump Statements: These are used to skip or repeat parts of your code. There are several types of jump statements, including: Break statement: This is used to exit a loop or switch statement. Continue statement: This is used to skip the current iteration of a loop and move on to the next one.
Example:
let i = 0;
while (i < 5) {
console.log(i);
if (i === 2) {
break;
}
i++;
}
Return Statements: These are used to exit a function and return a value. If no return statement is provided, the function will implicitly return undefined.
Example:
function addNumbers(a, b) {
let sum = a + b;
return sum;
}
let result = addNumbers(2, 3);
console.log(result); // Output: 5
In summary, control statements in JavaScript are used to direct the flow of your program, make decisions, and execute different parts of your code depending on conditions or criteria. Understanding how to use these statements effectively is crucial for writing efficient, readable, and maintainable code.
Word count: 306