What are the different types of java with examples
What are the different types of java with examples
I'd be happy to help! Here's a comprehensive overview of the different types of Java, along with examples:
1. Java Standard Edition (JSE)
Java Standard Edition is the most widely used and well-known type of Java. It is used for developing platform-independent applications, including web applications, Android apps, desktop applications, and more.
Example: A simple "Hello World" program using JSE:
public class HelloWorld {
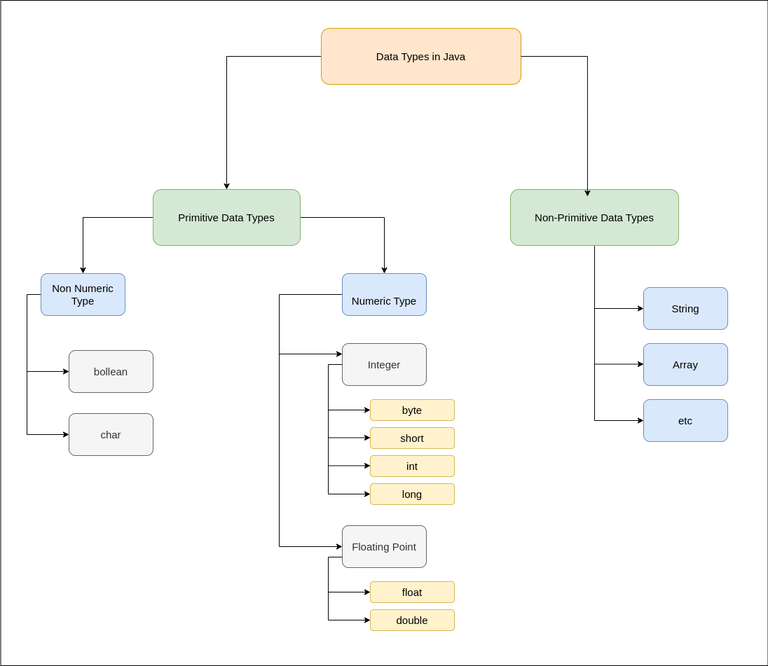
public static void main(String[] args) {
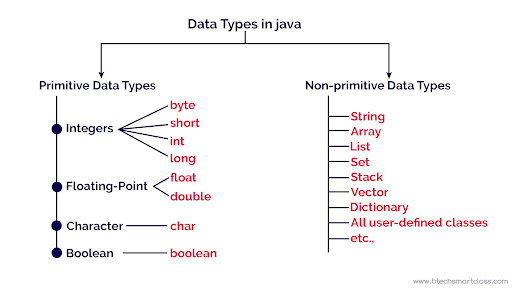
System.out.println("Hello World!");
}
}
2. Java Micro Edition (JME)
Java Micro Edition is designed for developing mobile applications, such as games, utilities, and more. It is optimized for small footprint and low-memory devices.
Example: A simple JME game:
import javax.microedition.lcdui.*;
import java.io.*;
public class SnakeGame extends MIDlet {
private GameCanvas canvas;
private Snake snake;
public SnakeGame() {
canvas = new GameCanvas();
snake = new Snake(canvas);
}
public void startApp() {
Display.getDisplay(this).setCurrent(canvas);
}
}
3. Java Enterprise Edition (JEE)
Java Enterprise Edition is designed for developing large-scale, distributed applications, including web services, enterprise systems, and more.
Example: A simple JEE web application:
import javax.servlet.*;
import java.io.*;
public class HelloServlet extends HttpServlet {
public void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
response.setContentType("text/plain");
PrintWriter out = response.getWriter();
out.println("Hello from Java!");
}
}
4. Java ME Embedded (JMEED)
Java ME Embedded is designed for developing embedded systems, including those used in appliances, vehicles, and more.
Example: A simple JMEED application controlling a robotic arm:
import com.intel.jmeed.*;
public class RoboticArmController extends JMEApplication {
private Motor motor;
private Servo servo;
public RoboticArmController() {
motor = new Motor();
servo = new Servo();
}
public void startApp() {
motor.setSpeed(50);
servo.setPosition(30);
}
}
5. Java Card Edition (JCE)
Java Card Edition is designed for developing smart card applications, including those used in identity verification, secure transactions, and more.
Example: A simple JCE applet verifying a user's identity:
import javacard.framework.*;
public class IdentityVerifier implements Applet {
private byte[] id;
public void installApplet(APDU apdu) {
id = new byte[16];
// read ID from smart card
}
public void processAPDU(APDU apdu) {
if (verifyID(id)) {
System.out.println("User identity verified!");
} else {
System.out.println("Invalid user identity!");
}
}
}
These are just a few examples of the different types of Java, each with its own unique characteristics and use cases. I hope this helps!
5 types of Java
I apologize for the misunderstanding earlier!
Java is a popular programming language known for its platform independence and vast ecosystem of libraries, frameworks, and tools. There are several subcategories or flavors of Java, each with its unique characteristics, strengths, and use cases. Here are five types of Java:
Standard Edition (SE) Java: This is the most widely used type of Java, also known as "Java SE." It includes the fundamental libraries, APIs, and tools for developing platform-independent applications. Standard Edition Java provides support for features like networking, GUI programming, multithreading, and file I/O. It's suitable for developing a wide range of applications, from games to business software. Enterprise Edition (EE) Java: As its name suggests, Enterprise Edition Java is designed specifically for large-scale enterprise environments. It builds upon the Standard Edition and adds more features for distributed systems, including Java Server Pages (JSP), Servlets, and JavaServer Faces (JSF). This edition also includes support for advanced database technologies like JDBC and JPA. It's ideal for developing complex business applications with multiple tiers. Micro Edition (ME) Java: Micro Edition Java is designed for resource-constrained devices, such as embedded systems, smart cards, and mobile phones. It's optimized for small memory footprints, low power consumption, and fast execution speeds. This edition provides a lightweight JVM (Java Virtual Machine) and simplified APIs to simplify development. It's perfect for developing applications that require high performance, low latency, or limited resources.Each type of Java has its unique characteristics and use cases. By choosing the right flavor of Java, developers can create innovative solutions that meet specific needs and constraints.