Which map is synchronized in Java?
Which map is synchronized in Java?
I'm happy to help you with your question!
In Java, there are several maps that can be synchronized. A synchronized map is a map that allows multiple threads to access and modify its contents concurrently without causing issues such as data corruption or race conditions.
Here are some of the most commonly used synchronized maps in Java:
ConcurrentHashMap: This is one of the most popular synchronized maps in Java. It's designed for high-performance, concurrent access, and is highly scalable. ConcurrentHashMap uses a combination of locks and fine-grained locking to provide excellent concurrency and performance. It's implemented as a hash table with buckets that can be accessed concurrently.To use ConcurrentHashMap, you would typically create an instance like this:
ConcurrentHashMap myMap = new ConcurrentHashMap<>();
CopyOnWriteArrayList: This is another synchronized map that's often used in Java applications. Unlike ConcurrentHashMap, CopyOnWriteArrayList is designed for concurrent access and modification of a list (not just a map). It provides excellent performance and concurrency by using a copy-on-write approach.
To use CopyOnWriteArrayList, you would typically create an instance like this:
CopyOnWriteArrayList myArrayList = new CopyOnWriteArrayList<>();
LinkedHashMap: This is another synchronized map in Java that provides fast fail-fast iterators and allows for efficient insertion and deletion of entries at a specific position. LinkedHashMap uses a combination of locks and fine-grained locking to provide excellent concurrency and performance.
To use LinkedHashMap, you would typically create an instance like this:
LinkedHashMap myMap = new LinkedHashMap<>();
In general, when deciding which synchronized map to use in your Java application, consider the following factors:
Concurrency requirements: If you need high-performance, concurrent access and modification of a map, ConcurrentHashMap might be the best choice. Thread-safety requirements: If you only need to ensure thread-safety for your map, LinkedHashMap or CopyOnWriteArrayList could be suitable options. Scalability requirements: If you expect your application to handle a large number of requests concurrently, ConcurrentHashMap is likely a better choice due to its excellent scalability.In summary, Java provides several synchronized maps that can help you achieve high-performance, concurrency, and thread-safety in your applications. The choice between ConcurrentHashMap, LinkedHashMap, or CopyOnWriteArrayList depends on your specific requirements and use case.
Which is synchronized in Java?
I'd be happy to explain the synchronization mechanism in Java!
In Java, synchronization refers to the process of controlling access to a shared resource or data structure by multiple threads. Synchronization ensures that only one thread can execute a critical section of code at a time, preventing conflicts and ensuring data integrity.
There are several ways to achieve synchronization in Java:
synchronized
is executed by only one thread at a time. This is done using the synchronize
keyword. When a thread calls a synchronized method on an object, it must acquire the lock on that object before executing the method.
public class MyClass {
public synchronized void doSomething() {
// code here
}
}
Synchronized Blocks: A block of code can be declared as synchronized
using a block statement. This ensures that only one thread can execute the code within the block at a time.
public class MyClass {
public void doSomething() {
synchronized (this) {
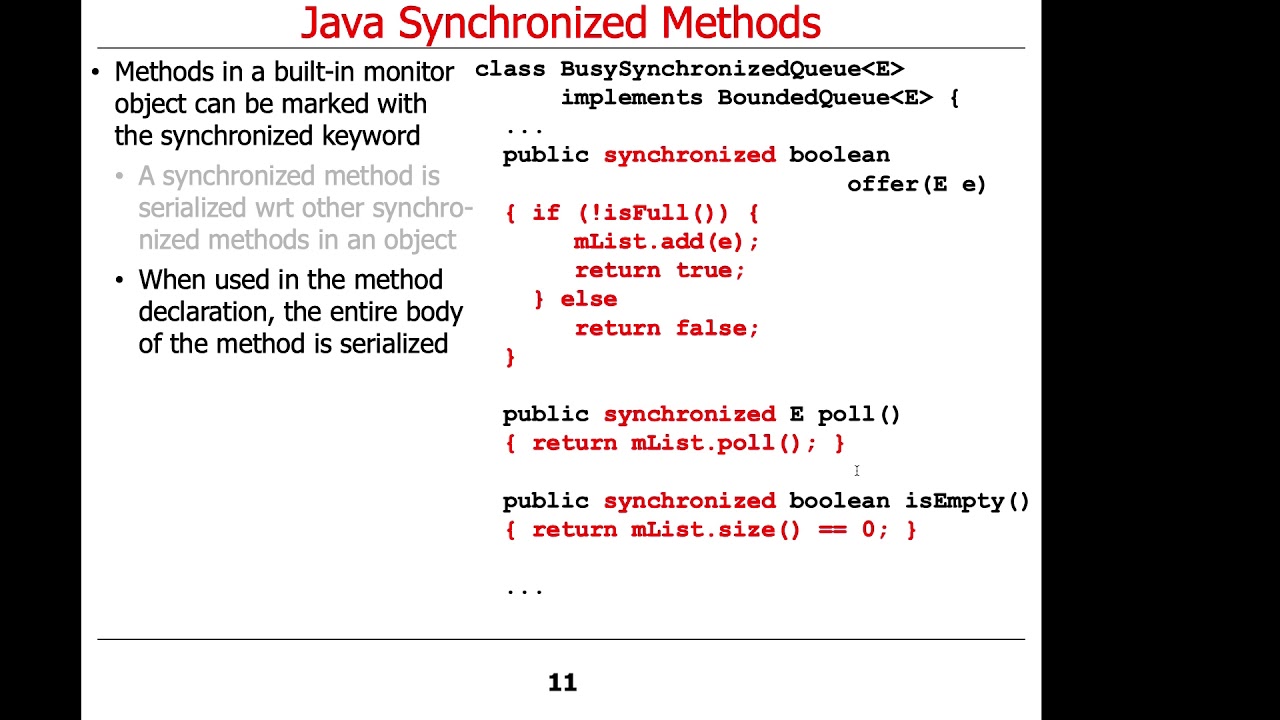
// code here
}
}
}
Lock Objects: Java 5 introduced the ReentrantLock
class, which allows developers to create custom locks for synchronization purposes. Locks can be used to synchronize access to shared resources or data structures.
public class MyClass {
private final ReentrantLock lock = new ReentrantLock();
public void doSomething() {
lock.lock();
try {
// code here
} finally {
lock.unlock();
}
}
}
Atomic Variables: Java 5 also introduced atomic variables, which provide a way to update shared data structures in a thread-safe manner.
public class MyClass {
private AtomicInteger counter = new AtomicInteger(0);
public void doSomething() {
while (true) {
int i = counter.get();
if (i < 10) {
counter.incrementAndGet();
// code here
} else {
break;
}
}
}
}
Volatile Variables: Java provides a way to make variables volatile, which ensures that changes made by one thread are visible to other threads.
public class MyClass {
private volatile int counter = 0;
public void doSomething() {
// code here
}
}
In summary, Java provides several ways to achieve synchronization, including synchronized methods and blocks, lock objects, atomic variables, and volatile variables. Synchronization is crucial in multithreaded programming to ensure data integrity and prevent conflicts between threads.
Would you like me to elaborate on any of these topics or provide more examples?