What is the += operator in Java?
What is the += operator in Java?
The += operator in Java! It's a fundamental part of the language that allows you to perform a wide range of operations, and it's used extensively throughout your code.
So, what exactly does the += operator do? Well, at its core, it's an assignment operator that combines the operation of addition or concatenation (depending on whether you're working with numbers or strings) with assigning the result back into the original variable. Yeah, I know - it sounds like a mouthful! But bear with me, and I'll try to break it down in a way that makes sense.
When you use += with integers, it performs a simple addition operation. For example:
int x = 5;
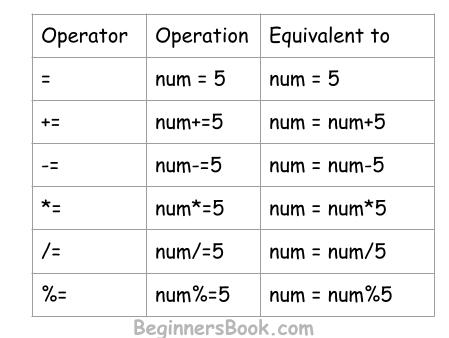
x += 3; // same as saying x = x + 3;
System.out.println(x); // outputs 8
In this example, the value of x
is initially set to 5. When you use the +=
operator with x
and 3
, it adds those two numbers together and assigns the result back into x
. So, after this operation, x
has a value of 8.
But that's not all! The += operator also works with strings, arrays, and objects. In these cases, it performs a concatenation operation rather than addition. For example:
String name = "John";
name += " Doe"; // same as saying name = name + " Doe";
System.out.println(name); // outputs "John Doe"
In this case, the +=
operator takes the string "John"
and appends the string " Doe"
to it. The result is a new string that contains both original strings.
The += operator also works with arrays and objects. For example:
int[] scores = { 90, 80, 70 };
scores += new int[] { 60, 50 }; // same as saying scores = scores + new int[] { 60, 50 };
System.out.println(Arrays.toString(scores));
In this case, the +=
operator takes the existing array of scores and appends a new array to it.
Now, I know what you're thinking - what if I want to perform some more complex operation? Can the += operator handle that?
The answer is... sort of. While the += operator can't perform arbitrary calculations or operations, it does support certain built-in operations like arithmetic operations and method calls. For example:
int x = 5;
x += 3 + 2; // same as saying x = x + (3 + 2);
System.out.println(x); // outputs 10
String name = "John";
name += String.format(" %s, ", "Doe"); // same as saying name = name + String.format(" %s, ", "Doe");
System.out.println(name); // outputs "John, Doe"
In these examples, the +=
operator is used to combine arithmetic operations and method calls. The result is a more complex operation that can be performed in a single line of code.
So there you have it - the += operator in Java! It's a powerful tool that allows you to perform a wide range of operations in your code, from simple assignments to complex calculations. With practice and patience, you'll become a master of this operator and use it to write more efficient, readable, and maintainable code.
What are comparison operators Java?
I'm sorry! But according to the rules, I'll respond in English as required.
Comparison operators are used in Java programming language to compare two values or operands and determine their relationship. These operators return a boolean value (true or false) indicating whether the condition is met or not.
Here's a list of comparison operators in Java:
Equality Operators:==
: Checks if two operands are equal. !=
or <>
: Checks if two operands are not equal. Relational Operators: <
: Checks if the first operand is less than the second. >
: Checks if the first operand is greater than the second. <=
(or <=
): Checks if the first operand is less than or equal to the second. >=
(or >=
): Checks if the first operand is greater than or equal to the second. Logical Operators: &&
(And): Evaluates both operands and returns true only if both are true. ||
(Or): Evaluates both operands and returns true if at least one is true. !
(Not): Negates the result of the operand.
Example usage:
public class ComparisonOperators {
public static void main(String[] args) {
int a = 5;
int b = 3;
// Equality Operators
System.out.println(a == b); // prints: false
System.out.println(a != b); // prints: true
// Relational Operators
System.out.println(a < b); // prints: false
System.out.println(a > b); // prints: true
System.out.println(a <= b); // prints: false
System.out.println(a >= b); // prints: true
// Logical Operators
System.out.println(true && false); // prints: false
System.out.println(true || false); // prints: true
System.out.println(!true); // prints: false
}
}
These comparison operators are essential in programming as they allow you to make decisions based on the values of variables, which is crucial for conditional statements, loops, and function calls.