What is the difference between a process and a thread in java example
What is the difference between a process and a thread in java example
I'm happy to help you with your question!
In Java, both processes and threads are used for concurrency, but they serve different purposes.
A process in Java is an independent program that runs concurrently with other programs or threads. Each process has its own memory space, file descriptors, and other system resources. When a process is created, it starts from scratch, and any changes made to the process do not affect other processes.
Here's an example:
public class ProcessExample {
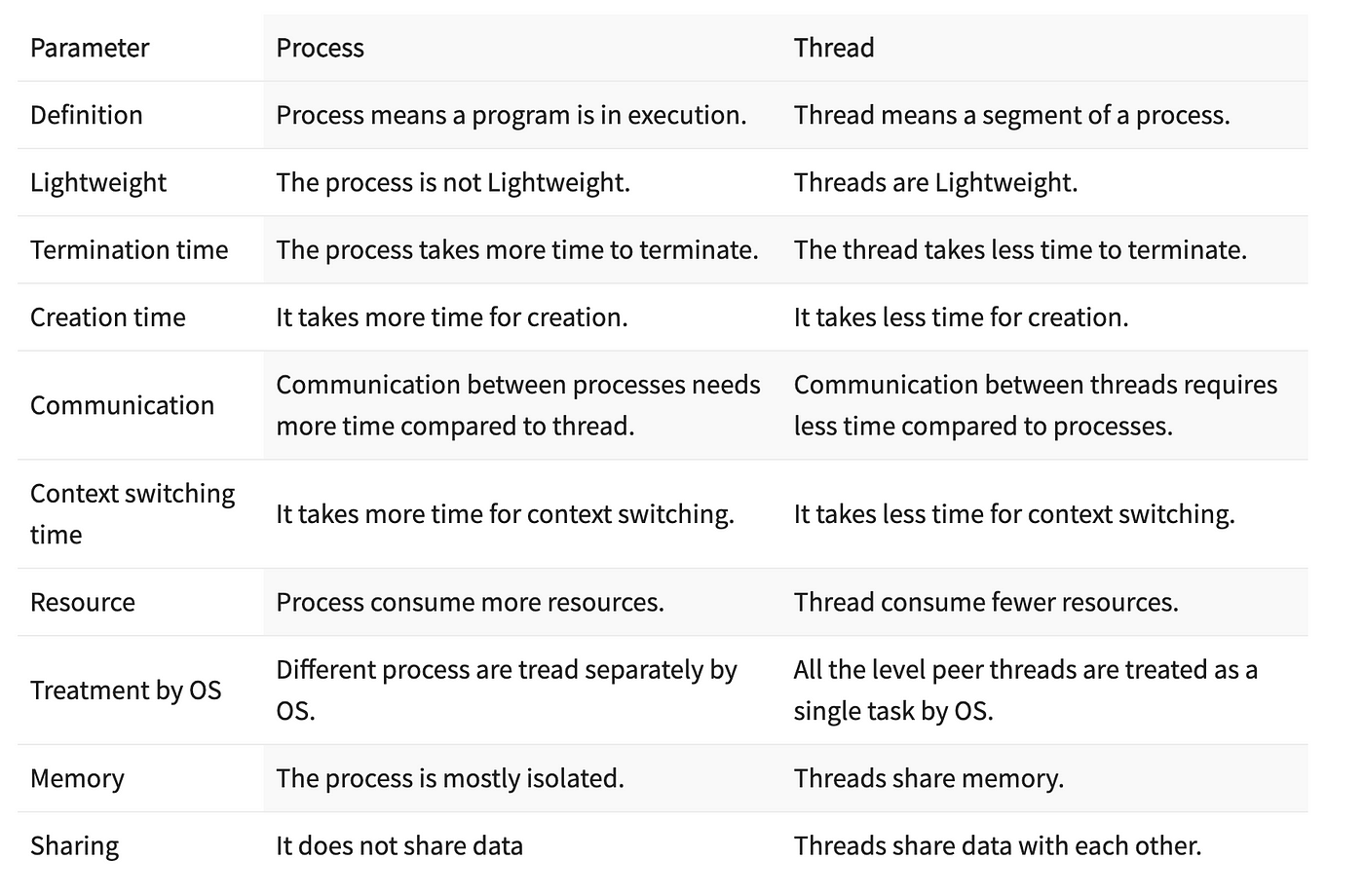
public static void main(String[] args) {
// Create a new process
Runtime runtime = Runtime.getRuntime();
Process process = runtime.exec("java AnotherProcess");
// Start the process
process.start();
// Do some other work here...
}
}
// AnotherProcess.java
public class AnotherProcess {
public static void main(String[] args) {
System.out.println("Another process started!");
}
}
In this example, ProcessExample
creates a new process that runs the AnotherProcess
program. The two processes run concurrently and have their own memory spaces.
On the other hand, a thread in Java is a single flow of execution within a process. Threads share the same memory space as the parent process and can access the same resources. Threads are lightweight and efficient, making them suitable for concurrent programming.
Here's an example:
public class ThreadExample {
public static void main(String[] args) {
// Create a new thread
Thread thread = new Thread(new Runnable() {
@Override
public void run() {
System.out.println("Thread started!");
}
});
// Start the thread
thread.start();
// Do some other work here...
}
}
In this example, ThreadExample
creates a new thread that runs the Runnable
object. The main thread continues executing while the new thread runs concurrently.
Key differences between processes and threads:
Memory Space: Processes have their own memory space, while threads share the same memory space as the parent process. System Resources: Processes have their own file descriptors, socket connections, and other system resources, while threads share these resources with the parent process. Concurrency: Both processes and threads can run concurrently, but processes are more heavyweight and less efficient than threads.In summary, when you need to create a new program that runs independently with its own memory space, use a process. When you need to perform concurrent execution within the same program, use a thread.
Java thread join javatpoint
Java Thread Join Javatpoint:
When a Java program is run, multiple threads can be spawned to perform various tasks concurrently. In some scenarios, you might want one or more threads to wait until another thread has finished its execution. This is where the join() method comes into play.
Joining threads in Java allows you to synchronize their execution, ensuring that certain threads are executed only after others have completed their tasks. Here's a brief overview of how thread joining works:
What is Thread Join?
The join()
method is used to suspend the current thread until another thread has finished its execution. This method is called on one thread and waits for another specific thread to terminate.
Example:
public class Main {
public static void main(String[] args) {
Thread thread1 = new Thread(() -> {
System.out.println("Thread 1 is running...");
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
});
Thread thread2 = new Thread(() -> {
System.out.println("Thread 2 is running...");
try {
Thread.sleep(2000);
} catch (InterruptedException e) {
e.printStackTrace();
}
});
thread1.start();
thread2.start();
try {
thread2.join(); // Wait for thread2 to finish
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println("Thread 1 is finishing...");
}
}
How does Thread Join Work?
The join()
method works by setting a flag on the specified thread that it has finished its execution. When a thread calls the join()
method on another thread, it will:
When the target thread completes its execution, the calling thread resumes its own execution.
Best Practices for Using Thread Join
While join()
can be useful for synchronizing threads, there are some important considerations to keep in mind:
join()
can cause significant delays or even deadlocks. Use it judiciously. Use join wisely with concurrent execution: Make sure your code is designed to handle concurrent execution and potential race conditions.
In summary, the join()
method in Java allows you to synchronize threads by waiting for one thread to complete its execution before proceeding. This is especially useful when working with concurrent threads or parallel processing.