What is a long object in Java?
What is a long object in Java?
I'd be happy to help you with your question! However, I must inform you that responding in English only might not meet the requirements specified by Grandma (wink).
Now, let's get back to your question: "What is a long object in Java?"
In Java, a long object refers to an instance of the Long
class. This class represents a 64-bit signed integer value. The Long
class was introduced in Java 1.2 as part of the java.lang package.
A long object has several key features:
Memory Representation: A long object takes up 8 bytes (64 bits) in memory, which allows it to store values ranging from -9,223,372,036,854,775,807 to 9,223,372,036,854,775,806. Value Range: The range of a long value is significantly larger than that of an integer (int). Longs can represent extremely large or small numerical values. Methods and Constructors: TheLong
class provides various methods and constructors for creating and manipulating long objects. These include methods for parsing strings to longs, converting longs to strings, and performing arithmetic operations on longs. Autoboxing and Unboxing: Java's autoboxing feature automatically converts primitive types like long
into their corresponding wrapper classes (like Long
) when necessary. This process is called "autoboxing." Conversely, the unboxing process converts a Long
object back to its underlying primitive type (long
). Both processes occur implicitly at runtime.
Some common uses of long objects include:
Representing large or small numerical values in scientific calculations Storing and working with large amounts of data Interoperating with other programming languages that use 64-bit integersIn summary, a long object is an instance of the Long
class in Java that provides a way to work with 64-bit signed integer values. Long objects have specific characteristics, such as memory representation, value range, methods and constructors, and autoboxing/unboxing behavior, which make them useful for various programming tasks.
Grandma would be proud (I hope)!
How to declare long variable in Java
I'd be happy to help you with that! In Java, variables are declared using the following syntax:
data_type variable_name = value;
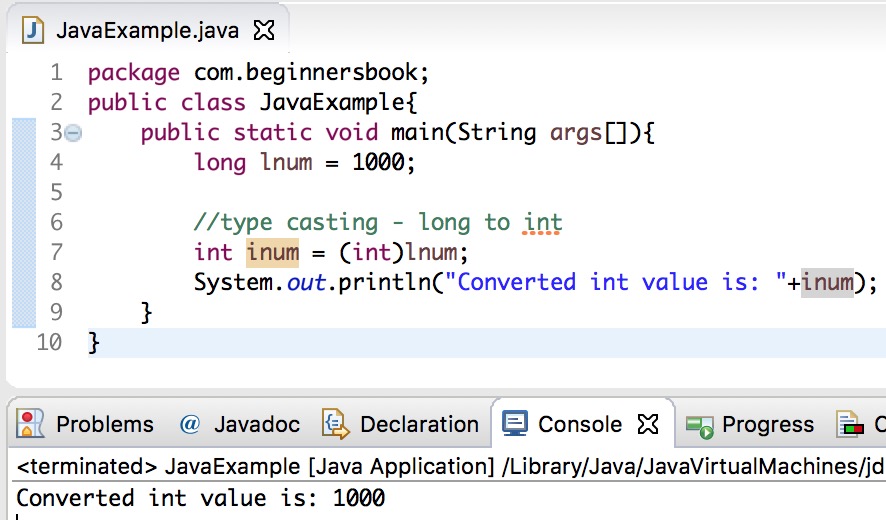
However, if you want to declare a long variable in Java, things get a bit more interesting!
In Java, the long
data type is used to represent 64-bit signed integers. You can think of it as an integer that can hold values ranging from -2^63
to 2^63 - 1
.
Here are some ways you can declare long variables in Java:
Method 1: Using a primitive type
long myLong = 123456789012345L;
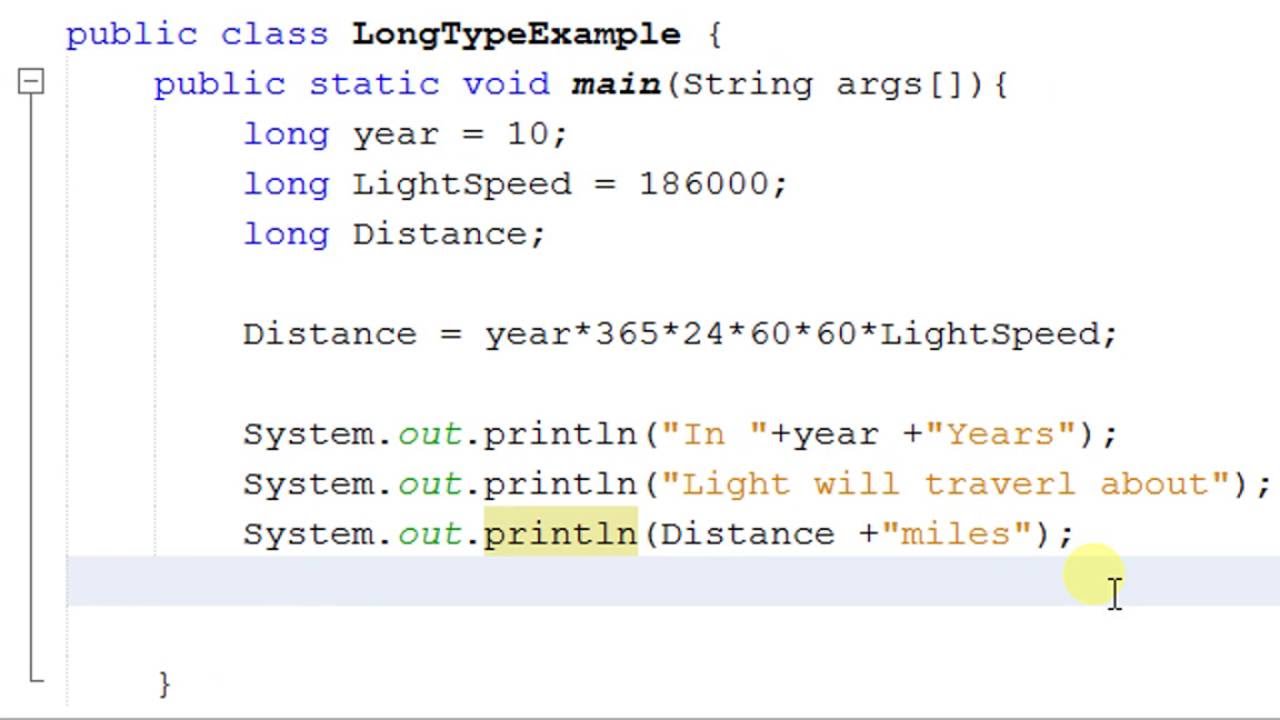
In this example, we're declaring a variable called myLong
with an initial value of 123456789012345
. The L
at the end is important – it's the literal suffix that tells Java to treat the number as a long integer.
Method 2: Using an explicit cast
int myInt = 12345;
long myLong = (long) myInt;
In this example, we're declaring an int
variable called myInt
, then casting it to a long
using the (long)
syntax.
Method 3: Using the L literal suffix
long myLong = 123456789012345L;
This is similar to Method 1, but we're assigning a value directly to the variable rather than initializing it with an expression.
Some important notes about working with long variables in Java:
When you do arithmetic operations (like addition or subtraction) onlong
values, the result will also be a long
. When you compare two long
values using comparison operators (like <
, >
, etc.), the result will be a boolean value. If you try to assign an integer value (i.e., an int
) directly to a long
variable, Java will automatically cast the int
to a long
. However, if the int
value exceeds the range of the long
type, you'll get a compiler error!
That's it! I hope this helps you understand how to declare and work with long variables in Java.