forEach lambda Java
forEach lambda Java
I'm happy to respond in English!
The forEach
method is a part of the Java Stream API, which was introduced in Java 8. It's used to perform an operation on each element in a stream.
A lambda expression (not lambda Java) is a shorthand way of writing a function that can be passed as an argument to certain methods, such as forEach
. The syntax for a lambda expression is (parameters) -> { body }
.
Here's the general syntax:
(parameters) -> {
// code to execute
}
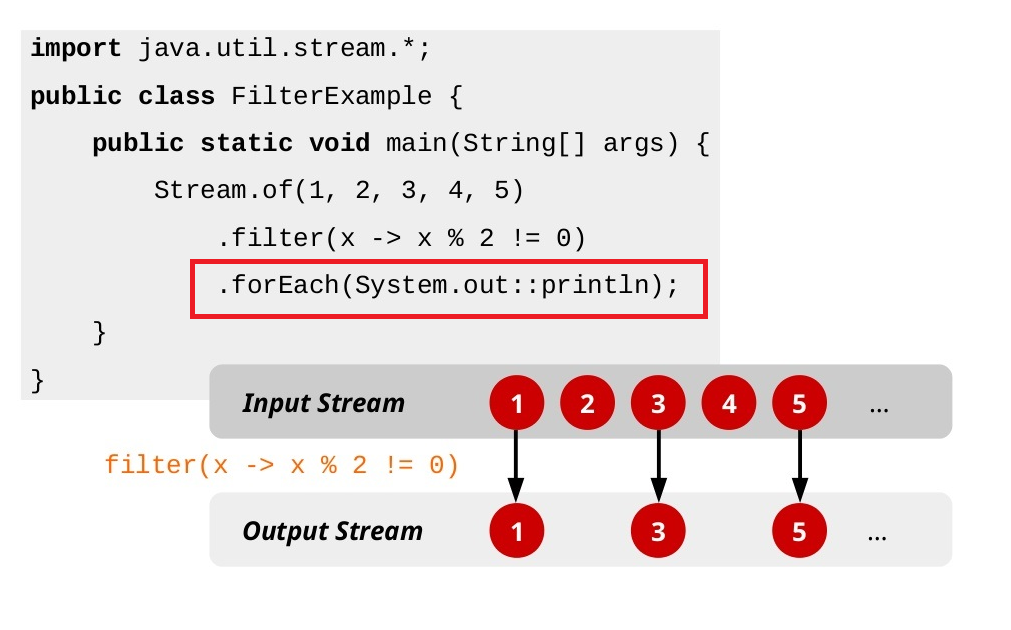
The parameters are the variables that will be passed when the lambda is executed. The body is the code that will be executed on each element in the stream.
Let's say we have a list of integers and we want to square each number using forEach
:
List numbers = Arrays.asList(1, 2, 3, 4, 5);
numbers.forEach(n -> n * n);
In this example, n
is the parameter that will be passed for each element in the list. The body of the lambda expression is n * n
, which squares each number.
Here's a more complex example where we use forEach
to print out the elements of a list:
List names = Arrays.asList("John", "Mary", "Jane");
names.forEach(name -> System.out.println(name));
In this case, name
is the parameter that will be passed for each element in the list. The body of the lambda expression is System.out.println(name)
, which prints out each name on a new line.
Using forEach
with lambda expressions can make your code more concise and readable. It's a powerful tool to have in your Java toolkit!
Additional details:
When usingforEach
, you can't return anything, as it's designed for side effects only. If an exception is thrown during the iteration, it will be propagated to the caller of forEach
. You can also use forEach
with other types of streams, such as IntStream
or DoubleStream
.
That's a brief overview of forEach
and lambda expressions in Java!
For loop in Java 8 for list
I'm happy to help you with your question!
In Java 8, the for
loop can be used to iterate over a list of objects. There are several ways to do this, depending on what you want to achieve.
Using an Enhanced For Loop
The first way is to use an enhanced for loop, which was introduced in Java 5. This type of loop allows you to iterate over a collection (such as a list) without having to use an explicit index variable.
List strings = Arrays.asList("Hello", "World", "Java");
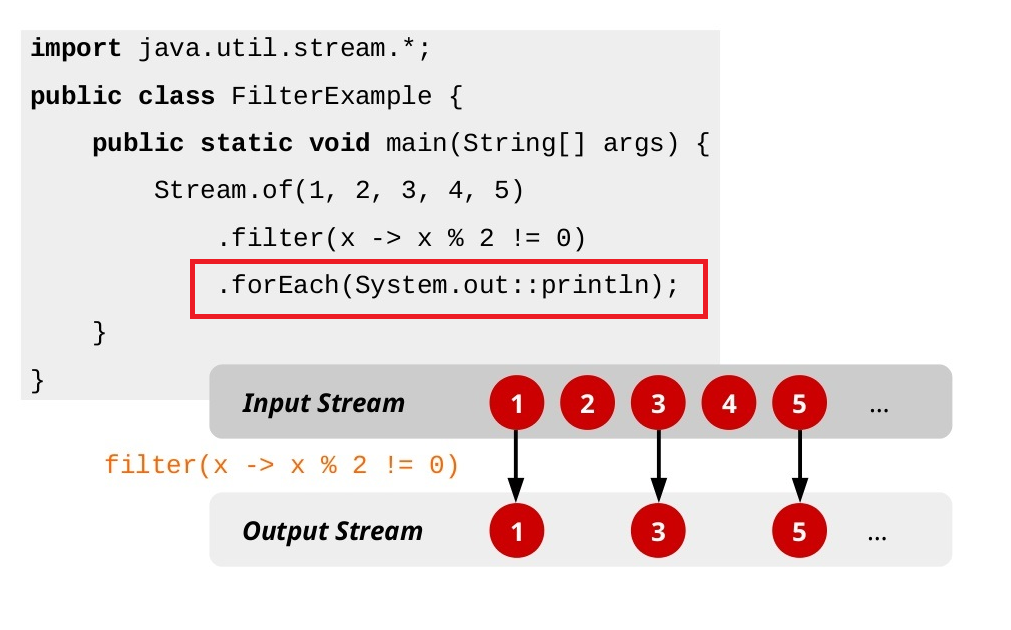
for (String s : strings) {
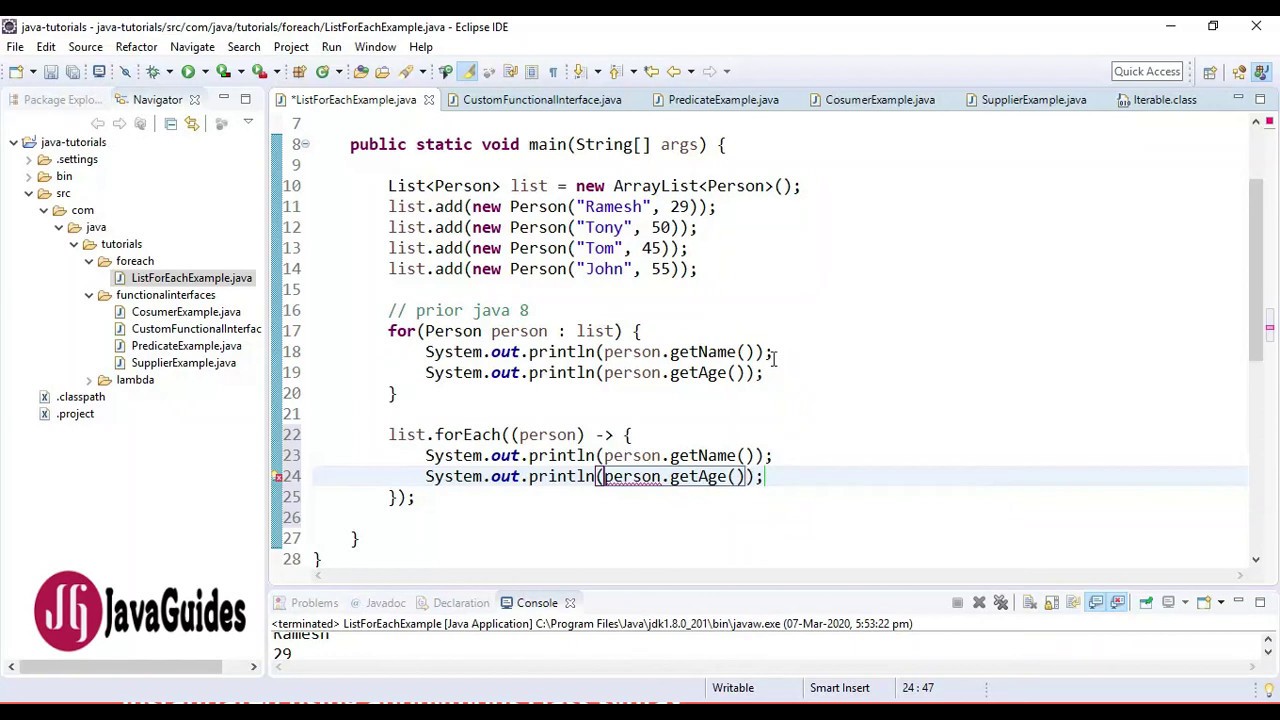
System.out.println(s);
}
In this example, the for
loop iterates over each element in the strings
list, assigning each element to the variable s
. The code inside the loop is executed once for each element.
Using an Iterator
Another way to iterate over a list in Java 8 is to use an iterator. You can create an instance of Iterator
and then call its next()
method repeatedly until there are no more elements.
List strings = Arrays.asList("Hello", "World", "Java");
Iterator it = strings.iterator();
while (it.hasNext()) {
String s = it.next();
System.out.println(s);
}
In this example, the for
loop iterates over each element in the strings
list using an iterator. The code inside the loop is executed once for each element.
Using Java 8's Stream API
Java 8 introduced a new way to iterate over collections called the Stream API. This API allows you to create a stream from a collection and then process it using various operations.
List strings = Arrays.asList("Hello", "World", "Java");
strings.stream()
.forEach(s -> System.out.println(s));
In this example, we create a stream from the strings
list and then use the forEach()
method to print each element.
Using Java 8's Lambda Expressions
Finally, Java 8 allows you to write lambda expressions inside your for loop. This can make your code more concise and expressive.
List strings = Arrays.asList("Hello", "World", "Java");
for (String s : strings) {
System.out.println(() -> System.out.println(s));
}
In this example, we use a lambda expression to print each element in the strings
list.
Conclusion
In Java 8, you have several options for iterating over a list of objects. You can use an enhanced for loop, an iterator, the Stream API, or even write a lambda expression inside your for loop. Each option has its own strengths and weaknesses, so choose the one that best fits your needs.
I hope this helps! Let me know if you have any further questions.