Builder pattern example Java 8
Builder pattern example Java 8
Here's an example of using the builder pattern in Java 8:
// A simple Person class with a constructor that takes name and age as parameters.
class Person {
private String name;
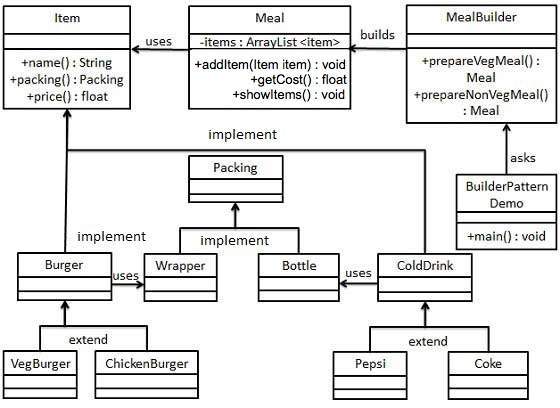
private int age;
public static class Builder {
private String name;
private int age = 0;
// Constructor that takes the required parameter (name).
public Builder(String name) {
this.name = name;
}
// Method to set the age.
public Builder age(int age) {
this.age = age;
return this; // This allows us to chain method calls.
}
// Method to get the built Person object.
public Person build() {
return new Person(this);
}
}
private Person(Builder builder) {
name = builder.name;
age = builder.age;
}
@Override
public String toString() {
return "Person{" +
"name='" + name + ''' +
", age=" + age +
'}';
}
}
public class BuilderPatternExample {
public static void main(String[] args) {
// Create a Person with the builder pattern.
Person person = new Person.Builder("John")
.age(30)
.build();
System.out.println(person.toString());
}
}
In this example, we have a Person
class that has a constructor with two parameters: name
and age
. We also have an inner class called Builder
which is used to build the Person
object. This builder pattern allows us to create complex objects step by step.
We can create a new person object like this:
person = new Person.Builder("John")
.age(30)
.build();
This way, we have more control over how our Person
objects are constructed and it makes the code easier to read and maintain.
Abstract builder pattern Java
I'd be happy to explain the Builder design pattern in Java!
What is the Builder Pattern?
The Builder pattern is a creational design pattern that allows you to construct complex objects step-by-step, separating the construction and representation of the object's internal state. This pattern helps solve the problem of building complex objects when their construction requires multiple steps or dependencies.
Problem Statement:
Suppose we're building a Java class, Car
, that represents a vehicle with properties like color
, model
, year
, and engineType
. We want to create different types of cars (e.g., sports car, family sedan) with varying characteristics. Using traditional constructors or setters would lead to code duplication and make the object's construction more complicated.
Solution:
We'll use the Builder pattern to build a Car
object in a step-by-step manner, allowing us to construct different types of cars without modifying the original class.
public class Car {
private String color;
private String model;
private int year;
private EngineType engineType;
public Car(Builder builder) {
this.color = builder.getColor();
this.model = builder.getModel();
this.year = builder.getYear();
this.engineType = builder.getEngineType();
}
public static class Builder {
private String color;
private String model;
private int year;
private EngineType engineType;
public Builder(String color) {
this.color = color;
}
public Builder model(String model) {
this.model = model;
return this;
}
public Builder year(int year) {
this.year = year;
return this;
}
public Builder engineType(EngineType engineType) {
this.engineType = engineType;
return this;
}
public Car build() {
return new Car(this);
}
}
}
How it Works:
We create aBuilder
class that contains the necessary data members (attributes) to construct a Car
object. The Builder
class provides methods (model
, year
, engineType
) for setting each attribute step-by-step. Each method returns this
, allowing us to chain multiple calls and build the car in a fluent manner. We create a constructor in the Car
class that takes a Builder
object as its parameter. This constructor sets all the attributes from the Builder
. To build a car, we create an instance of the Builder
, set each attribute step-by-step, and then call the build()
method to construct the final Car
object.
Benefits:
The Builder pattern offers several advantages:
Separation of Concerns: The construction and representation of theCar
object are decoupled. Flexibility: We can create different types of cars without modifying the original class. Readability: The code is more readable, as each step of the construction process is clear and concise.
By using the Builder pattern in Java, you can efficiently construct complex objects while maintaining a clean, modular design.