How to write multiple if-else conditions in Java?
How to write multiple if-else conditions in Java?
I'd be happy to explain how to write multiple if-else
conditions in Java!
In Java, you can use the traditional if-else
statement to check for multiple conditions and execute different blocks of code accordingly. Here's a step-by-step guide on how to do it:
if
statement:
int age = 25;
if (age >= 18) {
System.out.println("You are an adult.");
}
In this case, the code inside the if
block will only be executed if the condition age >= 18
is true.
if
, else if
, and else
statements to check for different conditions:
int score = 90;
if (score > 95) {
System.out.println("You got an A!");
} else if (score >= 85) {
System.out.println("You got a B.");
} else if (score >= 75) {
System.out.println("You got a C.");
} else {
System.out.println("You need to try harder.");
}
In this example, the code will check for the condition score > 95
first. If it's true, it will print "You got an A!". If not, it will move on to the next condition score >= 85
, and so on. The else
statement is executed only if none of the conditions are met.
int grade = 'A';
if (grade == 'A' || grade == 'B') {
if (student.isHonorsStudent()) {
System.out.println("You're an honors student!");
} else {
System.out.println("You're a regular student.");
}
} else if (grade == 'C' || grade == 'D' || grade == 'F') {
System.out.println("You need to retake the course.");
}
In this example, the code checks for the condition grade == 'A' || grade == 'B'
. If true, it then checks if the student is an honors student. If not, it prints a different message. The subsequent conditions are only checked if the previous one is false.
int temperature = 25;
if (temperature > 20) {
if (isRainy()) {
System.out.println("It's a rainy day!");
} else {
System.out.println("The weather is nice.");
}
} else {
System.out.println("The temperature is too low.");
}
In this example, the code first checks for the condition temperature > 20
. If true, it then evaluates whether it's a rainy day. If not, it prints a different message.
Remember to use proper indentation and formatting to make your code readable! In Java, you can also use switch
statements to handle multiple conditions, but that's a topic for another time.
That's it! I hope this helps you write more efficient and readable code in Java.
What is the difference between nested if and ifs in Java?
I'm happy to help you with your query!
In Java, both "nested ifs" and "ifs" are used to control the flow of a program by making decisions based on certain conditions. However, the key difference between them lies in their structure and functionality.
If Statements (Single Level):
A single-level if statement is a standalone statement that checks a condition and executes a block of code if the condition is true. The basic syntax for an if statement in Java is:
if (condition) {
// code to execute if condition is true
}
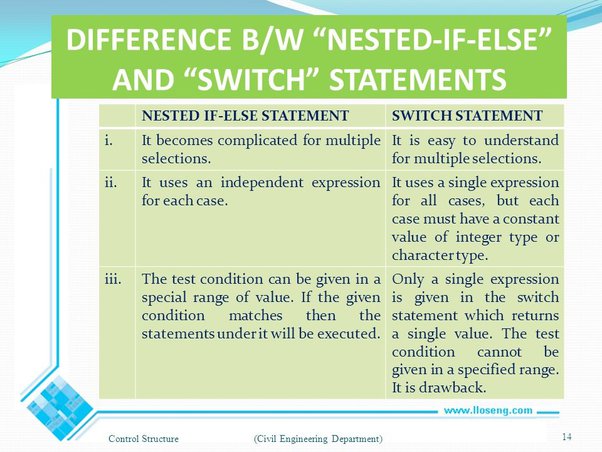
Here, the condition is evaluated, and if it's true, the code inside the curly braces is executed. If the condition is false, the code is skipped.
Nested If Statements:
A nested if statement, also known as an "if-else-if" or "chained if," is when one if statement is enclosed within another if statement (or multiple levels of if statements). This allows you to check multiple conditions and execute different blocks of code based on the results. The basic syntax for a nested if statement in Java is:
if (condition1) {
if (condition2) {
// code to execute if condition1 and condition2 are true
} else if (condition3) {
// code to execute if condition1 is true but condition2 is false
}
}
In this example, we have two levels of if statements. The outer if statement checks condition1
, and if it's true, the inner if statement checks condition2
. If condition2
is also true, the code inside the inner curly braces is executed.
Key Differences:
The main differences between single-level if statements and nested if statements are:
Depth of decision-making: Nested if statements allow for more complex decision-making by evaluating multiple conditions in a hierarchical manner. Single-level if statements only evaluate a single condition. Code execution: In a single-level if statement, the code is executed if the condition is true. In a nested if statement, the code is executed based on the result of the outermost if statement and then possibly again for inner if statements. Readability and maintainability: Nested if statements can make your code more difficult to read and maintain due to their complexity. Single-level if statements are generally easier to understand and debug.When to Use Each:
Single-level if statements are suitable when you need to check a single condition and execute different blocks of code based on the result.
Nested if statements are useful when you need to evaluate multiple conditions in a hierarchical manner, such as when processing user input or handling errors. They can also be used to implement complex logic with multiple "if-else" scenarios.
In conclusion, while both single-level if statements and nested if statements serve similar purposes, the key difference lies in their structure and functionality. Single-level if statements are simpler and more straightforward, whereas nested if statements allow for more complex decision-making and code execution based on multiple conditions.