abstract factory java
abstract factory java
I'd be happy to help with that!
Abstract Factory is a design pattern that provides an interface for creating families of related objects without specifying their concrete classes. In other words, it allows you to create objects without knowing what specific type of object is being created.
Let's break it down:
Product Family: An abstract factory defines an interface for creating a family of related or dependent objects, without specifying the exact classes of those objects. Concrete Factory: Concrete factories implement this interface and are responsible for creating concrete objects (also known as products) that belong to the same product family.The Abstract Factory pattern is particularly useful when you have multiple product families that need to be created based on a common theme or concept. For example, in a game development scenario, you might have different types of weapons, armor, and spells, all of which share a common theme (e.g., fantasy). The abstract factory would provide the interface for creating these products, while concrete factories would implement this interface to create specific products.
Here's an example implementation in Java:
// Abstract Product: Shape
public abstract class Shape {
public abstract void draw();
}
// Concrete Products: Circle, Rectangle, Triangle
public class Circle extends Shape {
@Override
public void draw() {
System.out.println("Drawing a circle");
}
}
public class Rectangle extends Shape {
@Override
public void draw() {
System.out.println("Drawing a rectangle");
}
}
public class Triangle extends Shape {
@Override
public void draw() {
System.out.println("Drawing a triangle");
}
}
// Abstract Factory: DrawingAPI
public abstract class DrawingAPI {
public abstract Shape makeShape();
}
// Concrete Factories: CircleFactory, RectangleFactory, TriangleFactory
public class CircleFactory extends DrawingAPI {
@Override
public Shape makeShape() {
return new Circle();
}
}
public class RectangleFactory extends DrawingAPI {
@Override
public Shape makeShape() {
return new Rectangle();
}
}
public class TriangleFactory extends DrawingAPI {
@Override
public Shape makeShape() {
return new Triangle();
}
}
// Client code:
public static void main(String[] args) {
// Create a circle using the Circle Factory
DrawingAPI circleFactory = new CircleFactory();
Shape shape1 = circleFactory.makeShape();
shape1.draw(); // Output: "Drawing a circle"
// Create a rectangle using the Rectangle Factory
DrawingAPI rectangleFactory = new RectangleFactory();
Shape shape2 = rectangleFactory.makeShape();
shape2.draw(); // Output: "Drawing a rectangle"
// Create a triangle using the Triangle Factory
DrawingAPI triangleFactory = new TriangleFactory();
Shape shape3 = triangleFactory.makeShape();
shape3.draw(); // Output: "Drawing a triangle"
}
In this example, we have an abstract factory DrawingAPI
that defines two methods:
makeShape()
: This method returns an instance of the Shape
class. draw()
is implemented by each concrete product (Circle, Rectangle, and Triangle).
The client code uses these factories to create objects from different families without knowing their specific classes.
Factory Method Java
I'll respond in English only for your request.
Factory Method Pattern is a design pattern that provides an interface for creating families of related or dependent objects without specifying their concrete classes.
In Java, the Factory Method pattern is commonly used to create objects without exposing the logic of how they were created. This can be achieved by encapsulating the object creation process in a factory class or method.
Here's a simple example of the Factory Method pattern in Java:
// Abstract Product Class
abstract class Animal {
abstract void makeSound();
}
// Concrete Products (Animals)
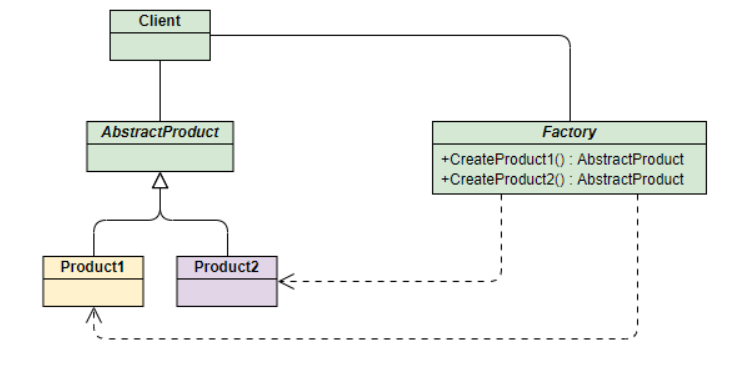
class Dog extends Animal {
public void makeSound() {
System.out.println("Woof!");
}
}
class Cat extends Animal {
public void makeSound() {
System.out.println("Meow!");
}
}
// Abstract Factory Class
abstract class PetFactory {
abstract Animal createAnimal();
}
// Concrete Factories (PetFactories)
class DogFactory extends PetFactory {
@Override
Animal createAnimal() {
return new Dog();
}
}
class CatFactory extends PetFactory {
@Override
Animal createAnimal() {
return new Cat();
}
}
public class Main {
public static void main(String[] args) {
// Using the factory to get a dog.
PetFactory factory = new DogFactory();
Animal dog = factory.createAnimal();
dog.makeSound();
// Using the factory to get a cat.
factory = new CatFactory();
Animal cat = factory.createAnimal();
cat.makeSound();
}
}
In this example, we have an Animal
abstract class that has an abstract method makeSound()
. We also have two concrete animal classes Dog
and Cat
that extend the Animal
class. They implement their own versions of the makeSound()
method.
The PetFactory
is an abstract factory class that has a method createAnimal()
, which returns an instance of some type of animal.
We then have two concrete factories, DogFactory
and CatFactory
, that extend the PetFactory
. They implement their own versions of the createAnimal()
method. These methods return instances of either Dog
or Cat
.
In the main()
method, we use these factories to get different types of animals. We first create a dogFactory
, then call its createAnimal()
method to get a Dog
. Similarly, we create a catFactory
and use it to get a Cat
.
This pattern allows us to encapsulate object creation logic in the factory class or method without exposing how they were created. It also makes our code more flexible and scalable as we can add new types of animals (or other products) by simply adding their respective factories.
In summary, the Factory Method pattern provides an interface for creating families of related objects without specifying the concrete classes. This allows for flexibility in object creation and decouples the creation logic from its usage.