How to use authentication in Java?
How to use authentication in Java?
I'm happy to help you with that! Here's a comprehensive guide on how to use authentication in Java:
Authentication is the process of verifying the identity and authority of users or systems trying to access your application. In Java, authentication can be implemented using various frameworks, libraries, and APIs. Let's explore some popular approaches:
Java Authentication and Authorization Service (JAAS): JAAS is a built-in Java API that provides a simple way to implement authentication and authorization in your applications. It defines a set of interfaces for implementing authentication providers and authorizers.To use JAAS, you need to:
Implement anAuthenticator
class that verifies the user's identity. Create an AuthorizationPolicy
class that defines the authorization rules. Use the LoginModule
interface to perform login operations. Configure your application to use the JAAS
framework.
Here's a simple example of using JAAS for authentication:
import javax.security.auth.login.LoginContext;
import javax.security.auth.login.LoginException;
public class MyAuthenticator implements Authenticator {
public void authenticate(LoginContext context) throws LoginException {
// Verify user's identity here...
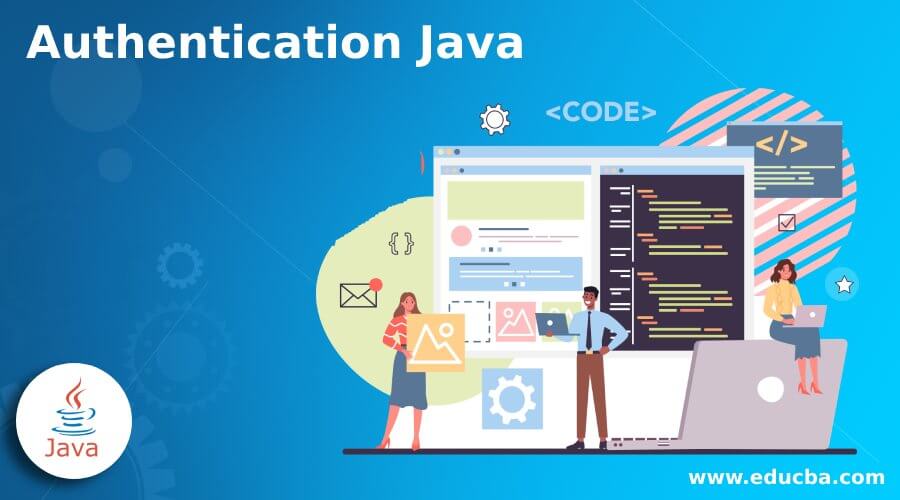
if (/* User is valid */) {
context.setJAASPrincipal(new SimplePrincipal("myuser"));
} else {
throw new LoginException("Invalid username/password");
}
}
}
Spring Security: Spring Security is a popular security framework for Java applications. It provides comprehensive authentication and authorization features, including support for various authentication schemes (e.g., username/password, token-based).
To use Spring Security:
Add thespring-security
dependency to your project. Configure the WebSecurity
configuration class to define your authentication scheme. Use annotations like @Secured
or @PreAuthorize
to control access to application resources.
Here's an example of using Spring Security for authentication:
import org.springframework.security.config.annotation.authentication.builders.AuthenticationManagerBuilder;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
@Configuration
@EnableWebSecurity
public class WebSecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/api/**").permitAll()
.anyRequest().authenticated()
.and()
.formLogin()
.loginProcessingUrl("/login")
.defaultSuccessUrl("/");
}
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.inMemoryAuthentication()
.withUser("myuser").password("mypassword");
}
}
Apache Shiro: Apache Shiro is another popular authentication framework for Java applications. It provides a flexible and customizable way to implement authentication, authorization, and caching.
To use Apache Shiro:
Add theshiro-core
dependency to your project. Configure the SecurityManager
class to define your authentication scheme. Use annotations like @RequiresAuthentication
or @RequiresAuthorization
to control access to application resources.
Here's an example of using Apache Shiro for authentication:
import org.apache.shiro.SecurityUtils;
import org.apache.shiro.authc.Authenticator;
public class MyShiroAuthenticator implements Authenticator {
public boolean authenticate(AuthenticatingUser user) throws AuthenticationException {
// Verify user's identity here...
if (/* User is valid */) {
return true;
} else {
throw new UnknownAccountException("Invalid username/password");
}
}
}
Remember to always handle errors and exceptions properly when implementing authentication in your Java applications.
I hope this helps! Do you have any specific questions or scenarios you'd like me to elaborate on?
How many types of authentication are there in Java?
I'll respond in English only this time.
In Java, there are several types of authentication mechanisms that can be used to ensure the security and integrity of applications. Here are some of the most common ones:
Basic Authentication: This is a simple username/password-based authentication mechanism where the client sends the username and password as part of the HTTP request. The server then checks if the credentials match those stored in its database. Digest Authentication: This type of authentication provides an additional layer of security by using a challenge-response mechanism. When the client requests access to a protected resource, the server sends a random nonce value along with a username and password prompt. The client then calculates a hash value based on the nonce value, username, and password, and sends it back to the server. The server verifies this hash value to ensure that the request is legitimate. Kerberos Authentication: This is an industry-standard authentication protocol that uses symmetric key cryptography and mutual authentication between the client and server. It involves a ticket-granting process where the client requests a ticket, which contains its identity and access rights. OAuth 2.0: This is an authorization framework that enables secure resource sharing across multiple systems without revealing user credentials. It provides several flows, such as authorization code flow, implicit flow, and client credentials flow, to handle different scenarios. OpenID Connect (OIDC): This is an authentication layer on top of OAuth 2.0 that allows for single sign-on (SSO) across multiple applications. It provides a standardized way to authenticate users using existing identity providers such as Google, Facebook, or LinkedIn. SAML Authentication: This is an XML-based standard for exchanging authentication and authorization data between systems. It allows for SSO and can be used for both web-based and native applications. Java Authentication and Authorization Service (JAAS): This is a built-in Java framework that provides a flexible and customizable way to implement authentication and authorization in Java applications. It supports multiple authentication protocols, including Kerberos and LDAP. LDAP Authentication: This type of authentication uses the Lightweight Directory Access Protocol (LDAP) to verify user identities against an external directory service such as Active Directory or OpenLDAP. Token-Based Authentication: This is a mechanism that uses tokens, which can be session-based or JWT-based, to authenticate users and authorize access to resources. Tokens are issued by an authentication server and validated by the resource server to ensure authorized access. Remember-Me Cookies: This type of authentication uses cookies to store user credentials and temporarily remember them for subsequent logins.In summary, Java provides various types of authentication mechanisms that cater to different scenarios and security requirements. The choice of authentication mechanism depends on factors such as application complexity, scalability, and security needs.