com.auth0 java-jwt maven
com.auth0 java-jwt maven
I'd be happy to help you with your question!
To integrate Java JWT (JSON Web Token) authentication into your Maven-based project using Auth0, you can follow these steps:
Step 1: Add Dependencies
In your pom.xml
file, add the following dependencies:
com.auth0
java-jwt
3.10.0
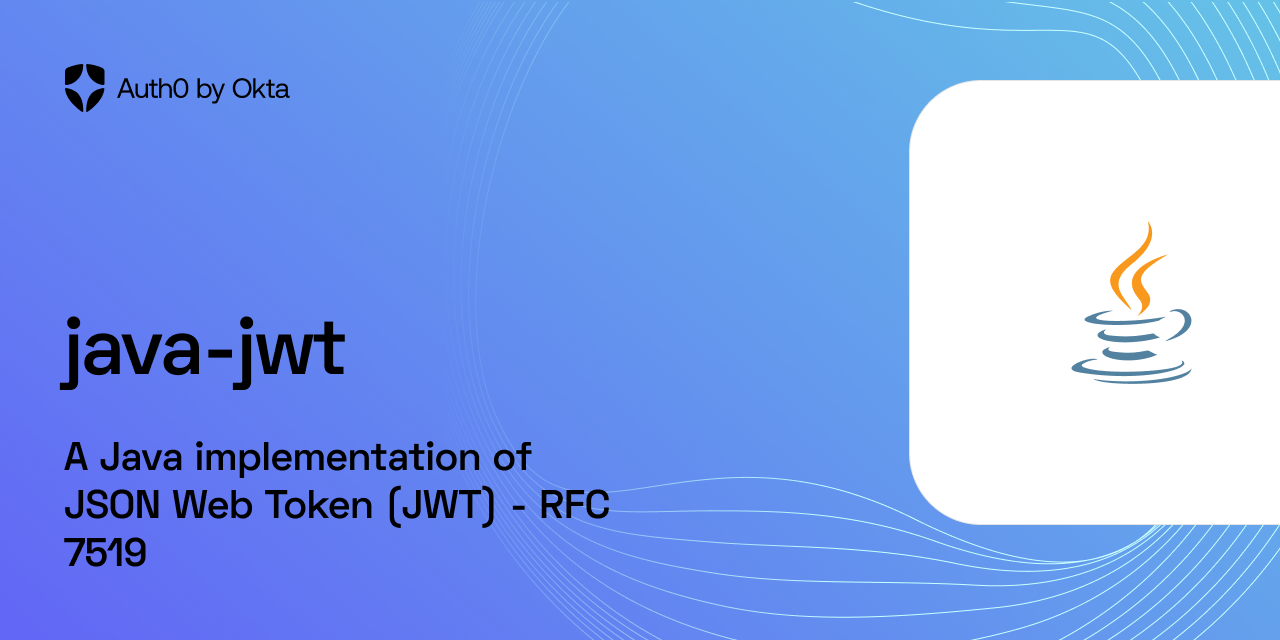
Step 2: Configure Auth0
Create a new file named auth0.properties
in your project's root directory, with the following content:
client.id=YOUR_CLIENT_ID
client.secret=YOUR_CLIENT_SECRET
domain=YOUR_AUTH0_DOMAIN
audience=YOUR_AUDIENCE
Replace YOUR_CLIENT_ID
, YOUR_CLIENT_SECRET
, YOUR_AUTH0_DOMAIN
, and YOUR_AUDIENCE
with your actual Auth0 credentials.
Step 3: Initialize Auth0
Create a new Java class, for example, Auth0Service.java
, to initialize the Auth0 client:
import com.auth0.jwt.JWT;
import com.auth0.jwt.JWTCreator;
import com.auth0.jwt.JwtBuilder;
import com.auth0.jwt.algorithms.HmacSha256;
import com.auth0.jwt.exceptions.TokenExpiredException;
public class Auth0Service {
private String clientId;
private String clientSecret;
private String domain;
private String audience;
public Auth0Service() {
try {
Properties props = new Properties();
props.load(new FileInputStream("auth0.properties"));
clientId = props.getProperty("client.id");
clientSecret = props.getProperty("client.secret");
domain = props.getProperty("domain");
audience = props.getProperty("audience");
} catch (IOException e) {
// Handle error
}
}
public String generateToken(String username, long expiresAt) {
JWTCreator.Builder builder = JWT.create()
.withClaim("sub", username)
.withExpiresAt(expiresAt);
return builder.sign(new HmacSha256(clientId, clientSecret));
}
}
This class loads the auth0.properties
file and initializes the Auth0 client using the client ID, secret, domain, and audience.
Step 4: Use Auth0 in Your Application
Now that you have your Auth0 service initialized, you can use it to generate and verify JWT tokens in your application. For example:
public class MyApplication {
public void handleRequest(HttpExchange exchange) {
String token = exchange.getRequestHeaders().getFirst("Authorization");
if (token != null && !token.isEmpty()) {
try {
String username = JWT.decode(token)
.getClaimValue("sub").asString();
// Verify the user's identity
} catch (TokenExpiredException e) {
// Handle token expiration
}
} else {
// The request is not authenticated, handle accordingly
}
}
}
In this example, we're using the Auth0Service
to verify a JWT token sent in an HTTP request header.
That's it! With these steps, you should now have a basic understanding of how to integrate Java JWT authentication into your Maven-based project using Auth0.
java-jwt maven
I'm happy to help you with your question about using Java JWT and Maven!
What is JSON Web Token (JWT)?
JSON Web Token (JWT) is a compact, URL-safe means of representing claims to be transferred between two parties. The tokens are digitally signed and contain identifying information about the user, such as their username or role. JWTs can be used to authenticate users, authorize access to specific resources, or validate the integrity of data.
Why use JSON Web Token (JWT) with Java?
JSON Web Tokens can be used in conjunction with Java to provide secure authentication and authorization mechanisms for your web applications. In a Java-based application, you can generate JWTs using the Java JWT library and then verify them on each subsequent request.
Getting Started with JSON Web Token (JWT) in Java
To get started with JSON Web Token (JWT) in Java, you'll need to add the following dependencies to your Maven project:
io.jsonwebtoken
jsonwebtoken
0.9.1
joda-time
joda-time
2.3
Here's an example of how you might use the JWT library to generate a token:
import io.jsonwebtoken.JwtBuilder;
import io.jsonwebtoken.Jwts;
public class Main {
public static void main(String[] args) throws Exception {
// Create claims for the token
JwtBuilder builder = Jwts.builder()
.setSubject("John Doe")
.setExpiration(Instant.now().plusSeconds(3600));
// Generate the token
String compactToken = builder.compact();
System.out.println(compactToken);
}
}
In this example, we're creating a JWT that contains a subject (the username "John Doe"), and an expiration time (1 hour from now).
Verifying JSON Web Tokens
To verify a JSON Web Token in Java, you can use the following code:
import io.jsonwebtoken.Jwts;
import io.jsonwebtoken.ExpiredJwtException;
public class Main {
public static void main(String[] args) throws Exception {
// Verify the token
try {
JwsHeader header = Jwts.parser()
.setVerificationKey("your-secret-key")
.parseClaimsJws(compactToken);
String username = header.getSubject();
System.out.println(username);
} catch (ExpiredJwtException e) {
System.out.println("Token has expired");
} catch (SecurityException | MalformedJwtException e) {
System.out.println("Invalid token");
}
}
}
In this example, we're verifying the JWT by parsing it and then checking its subject claim. If the token is valid and hasn't expired, we can access the username.
That's a basic overview of using JSON Web Tokens (JWTs) in Java with Maven! I hope this helps you get started with your project.
Please let me know if you have any further questions or concerns!