serializable interface in java
serializable interface in java
I'd be happy to help!
What is Serializable Interface?
In Java, Serializable
is an interface that can be implemented by any class whose instances need to be converted into a byte stream. It's a built-in interface provided by the Java API that allows an object to be serialized (converted into a byte stream) and deserialized (reconstructed from the byte stream).
How Does Serialization Work?
When an object is serialized, its state is captured and written to a byte stream in a compact binary format. The process involves the following steps:
Marking: The object's state is marked as needing to be serialized. Writing: The object's state is written to the output stream (such as a file or network connection). Reading: The serialized object is read from the input stream.Key Features of Serializable Interface
Here are some important aspects of the Serializable
interface:
Serializable
doesn't need to provide any methods. WriteObject() and ReadObject() Methods: While not strictly required, these methods can be used by subclasses to control how their objects are serialized or deserialized. Transient Fields: An object's transient fields (those marked with the transient
keyword) will not be written during serialization.
Benefits of Using Serializable Interface
Implementing Serializable
provides several benefits:
Common Use Cases
The Serializable
interface is often used in scenarios where:
Best Practices
When implementing Serializable
:
Conclusion
In summary, the Serializable
interface in Java provides a convenient way to serialize and deserialize objects. By implementing this interface, developers can create classes that can be easily converted into byte streams for persistent storage or data transfer. With minimal effort, they can enjoy the benefits of serialization without having to write custom serialization code.
Hope this explanation helps!
How to make fields Serializable in Java?
To make a field in Java serializable, you can follow these steps:
Implement the Serializable Interface: TheSerializable
interface is defined in the Java standard library and is used to indicate that an object's state (i.e., its fields) should be written out when the object is serialized, so it can later be reconstructed from the serialization stream.
In your class, you need to implement this interface. You do this by implementing the writeObject
and readObject
methods in your class. These methods are used by Java's serialization mechanism to save and restore an object's state.
Here is a simple example of how to use these methods:
public class MySerializableClass implements Serializable {
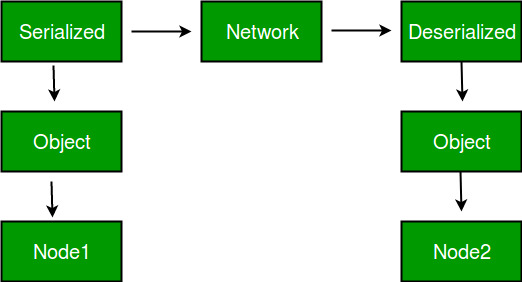
private String name;
private int age;
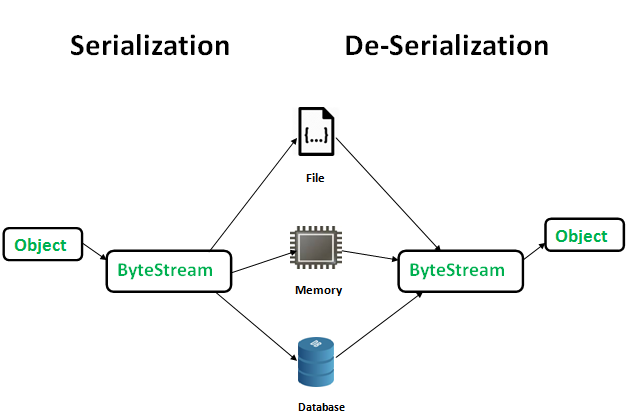
// Constructor, getters and setters...
private void writeObject(ObjectOutputStream out) throws IOException {
out.defaultWriteObject();
}
private void readObject(ObjectInputStream in) throws IOException, ClassNotFoundException {
in.defaultReadObject();
}
}
In this example, writeObject
and readObject
methods are empty because the default serialization mechanism of Java is sufficient for your class.
transient
keyword before these fields to indicate that they should be ignored during serialization and deserialization.
Here is an example:
public class MySerializableClass implements Serializable {
private transient String id;
private String name;
// Constructor, getters and setters...
}
In this example, the id
field will not be serialized because it's declared as transient
.
Here is an example using Externalizable
interface:
public class MySerializableClass implements Externalizable {
private String name;
private int age;
// Constructor, getters and setters...
public void writeExternal(ObjectOutput out) throws IOException {
out.writeObject(name);
out.writeInt(age);
}
public void readExternal(ObjectInput in) throws IOException, ClassNotFoundException {
name = (String) in.readObject();
age = in.readInt();
}
}
In this example, writeExternal
and readExternal
methods are used to customize the serialization and deserialization process.
Serializable
or Externalizable
interfaces.
Here is an example:
public class MySerializableClass {
private String name;
private int age;
// Constructor, getters and setters...
}
In this example, Java will automatically serialize and deserialize your object when you use the writeObject
and readObject
methods of a Stream
or OutputStream
.
Note that the Serializable
interface does not provide any guarantees about the serialization format. It's up to the class implementing this interface to decide how its state should be serialized.
Remember to declare all fields in your class as either transient
(if you don't want them serialized) or implement the necessary methods in your class if it implements Serializable
or Externalizable
.