Exception handling in java with examples w3schools geeksforgeek
Exception handling in java with examples w3schools geeksforgeek
I'm happy to help you with that!
Exception handling is an essential part of programming, and Java has a robust mechanism for it. In this response, I'll cover the basics of exception handling in Java using examples from W3Schools and GeeksforGeek.
What are Exceptions?
In Java, an exception is an object that represents an error or abnormal condition. When an exception occurs, the normal flow of program execution is interrupted, and the program jumps to the catch block (if one exists) to handle the exception.
Types of Exceptions
Java has two types of exceptions:
Try-Catch Blocks
The try-catch block is used to handle exceptions in Java. The general syntax is:
try {
// code that may throw an exception
} catch (ExceptionType e) {
// code to handle the exception
}
Let's take a simple example from W3Schools:
public class Main {
public static void main(String[] args) {
try {
int x = Integer.parseInt("abc");
} catch (NumberFormatException e) {
System.out.println("Invalid number format.");
}
}
}
In this example, we're trying to parse a string "abc" as an integer. Since "abc" is not a valid integer, the program throws a NumberFormatException
. The catch block catches this exception and prints an error message.
Multiple Catch Blocks
You can have multiple catch blocks for different types of exceptions:
public class Main {
public static void main(String[] args) {
try {
int x = Integer.parseInt("abc");
} catch (ArithmeticException e) {
System.out.println("Arithmetic exception occurred.");
} catch (NumberFormatException e) {
System.out.println("Invalid number format.");
}
}
}
In this example, we're catching both ArithmeticException
and NumberFormatException
. The order of the catch blocks is important - Java will check each block in order until it finds one that matches the exception type.
Finally Block
The finally block is executed regardless of whether an exception was thrown or not. It's commonly used to close resources, such as streams or connections:
public class Main {
public static void main(String[] args) {
try {
int x = Integer.parseInt("abc");
} catch (NumberFormatException e) {
System.out.println("Invalid number format.");
} finally {
System.out.println("Finally block executed.");
}
}
}
In this example, the finally block is executed even though an exception was thrown.
Throwing Custom Exceptions
You can also create your own custom exceptions by extending the Exception
class:
public class MyException extends Exception {
public MyException(String message) {
super(message);
}
}
Let's take an example from GeeksforGeek:
class MyException extends Exception {
MyException(String s) {
super(s);
}
}
public class Main {
public static void main(String args[]) {
try {
throw new MyException("This is my custom exception.");
} catch (MyException ex) {
System.out.println(ex.getMessage());
}
}
}
In this example, we're throwing a custom MyException
and catching it in the catch block.
That's it for now! I hope this helps you understand exception handling in Java better. Remember to always handle exceptions robustly to ensure your program remains stable and reliable.
Throws keyword in Java
I'd be happy to help you with that!
Here's an example of how you can use the throws
keyword in Java:
public class Calculator {
public int divide(int numerator, int denominator) throws ArithmeticException {
if (denominator == 0) {
throw new ArithmeticException("Cannot divide by zero!");
}
return numerator / denominator;
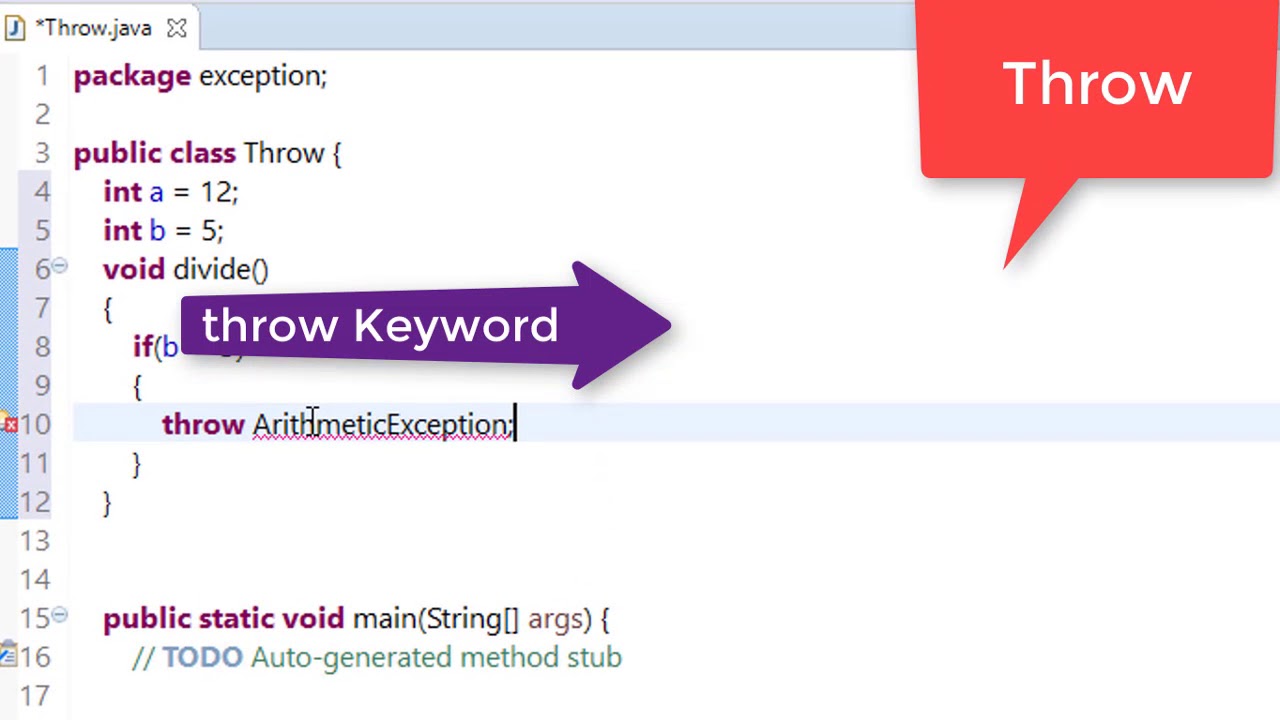
}
public static void main(String[] args) {
Calculator calculator = new Calculator();
try {
System.out.println(calculator.divide(10, 2));
} catch (ArithmeticException e) {
System.out.println(e.getMessage());
}
}
}
In this example, the divide
method is declared to throw an ArithmeticException
if the denominator is zero. The main
method calls the divide
method and handles the exception using a try-catch
block.
When you run this program, it will print:
5
Cannot divide by zero!
The first line prints the result of the division (5), and the second line prints the error message if an exception is thrown.
The throws
keyword is used to specify the types of exceptions that a method can throw. In Java, you must declare the exceptions that a method can throw before the method declaration using the throws
keyword.
For example:
public void foo() throws IOException {
// code here
}
In this example, the foo
method is declared to throw an IOException
.
When you use the throws
keyword, you must handle the exception in your method. You can do this by using a try-catch
block:
public void foo() throws IOException {
try {
// code here
} catch (IOException e) {
// error handling here
}
}
If you don't handle an exception, the program will crash when it's thrown. So, it's important to use the throws
keyword and handle exceptions in your code.
I hope this helps! Let me know if you have any questions or need further clarification.