Mockito version for java 17 github
Mockito version for java 17 github
I'd be happy to help you with that.
Mockito Version for Java 17 on GitHub
Mockito is a popular testing framework for Java that allows you to isolate and test individual units of your code, such as classes or methods, in isolation from the rest of your application. With Mockito, you can create mock objects that simulate the behavior of real objects, making it easier to write unit tests that are robust, reliable, and efficient.
What is Mockito?
Mockito is a Java-based library that provides a way to create mock objects that mimic the behavior of real objects. A mock object is an artificial substitute for a real object that you use in your test code to simulate the behavior of that object. Mockito allows you to create mock objects programmatically, which means you can define how they behave without having to write custom code.
How Does Mockito Work?
To use Mockito, you need to add it as a dependency to your Java project. Then, you can use the Mockito API to create mock objects and define their behavior. Here's a high-level overview of the process:
Create a mock object: Use theMockito.mock()
method to create a mock object for the class or interface you want to test. Define the behavior of the mock object: Use the various Mockito methods, such as when()
, thenReturn()
, and thenThrow()
, to define how the mock object should behave when called with certain inputs or under specific conditions.
Key Features of Mockito
Mockito has many features that make it a powerful tool for testing Java code. Some of its key features include:
Easy mocking: Mockito allows you to create mocks quickly and easily, without having to write custom code. Flexible behavior definition: You can define the behavior of your mock objects using a variety of methods, such aswhen()
, thenReturn()
, and thenThrow()
. Powerful mocking APIs: Mockito provides a range of APIs for creating and configuring mocks, including support for mocking final classes and interfaces. Support for Java 17: As you mentioned, Mockito supports Java 17 and is compatible with other popular testing frameworks like JUnit and TestNG.
Getting Started with Mockito on GitHub
If you're interested in getting started with Mockito, I recommend checking out the official Mockito GitHub repository. The repository contains a range of examples, tutorials, and documentation to help you get started with using Mockito in your Java projects.
In particular, you can check out the following resources:
Mockito Getting Started Guide: This guide provides an introduction to Mockito and its key features. Mockito Example Code: This section of the repository contains example code that demonstrates how to use Mockito in different scenarios. Mockito API Documentation: This documentation provides detailed information about the various Mockito APIs and methods.I hope this helps you get started with using Mockito for Java 17 on GitHub! Let me know if you have any further questions.
Mockito Java 17 maven dependency
I'd be happy to help you with that!
Mockito Maven Dependency for Java 17
To use Mockito in your Java project, you'll need to add the necessary dependencies to your pom.xml
file (if you're using Maven) or your build script (if you're using Gradle). Here are the steps:
Step 1: Add the Mockito dependency
Add the following dependency to your pom.xml
file:
org.mockito
mockito-core
5.2.0
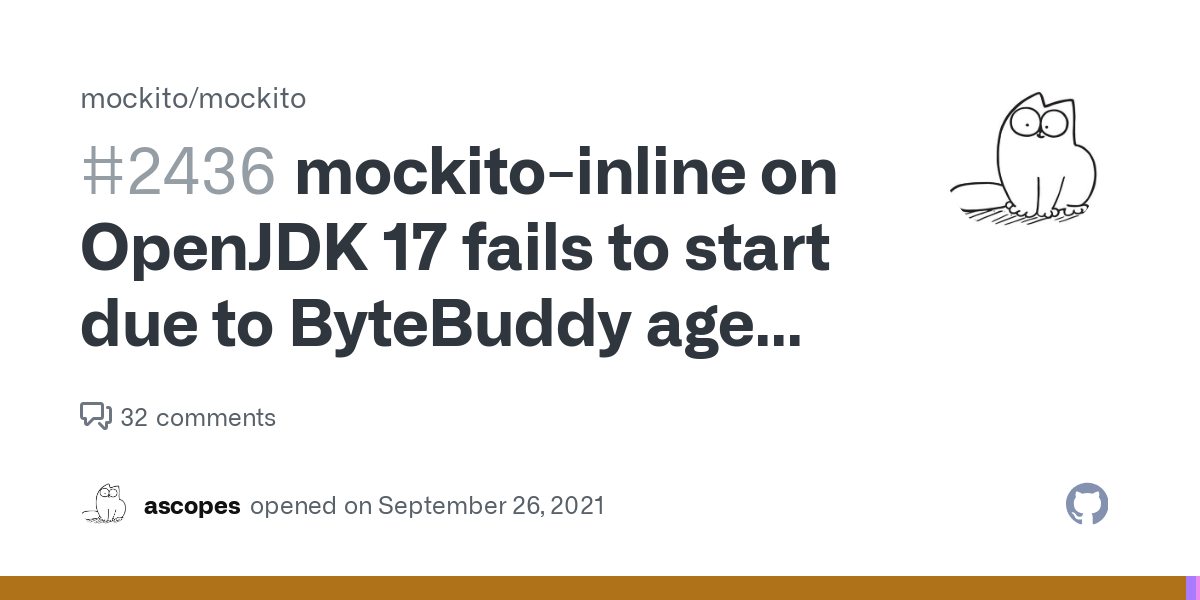
test
This will include the core Mockito library in your project.
Step 2: Add the Java 17 dependency (if needed)
If you're using Java 17, you'll also need to add the following dependency:
jakarta.xml.bind
jakarta.xml.bind-api
3.0.0
test
This is because Java 17 requires the jakarta.xml.bind
library for XML serialization.
Step 3: Configure your build script (if using Gradle)
If you're using Gradle, add the following dependencies to your build.gradle
file:
dependencies {
testImplementation 'org.mockito:mockito-core:5.2.0'
testImplementation 'jakarta.xml.bind:jakarta.xml.bind-api:3.0.0'
}
Step 4: Verify your dependencies
Once you've added the dependencies, verify that they're correctly included in your project by running mvn dependency:list
(for Maven) or ./gradlew dependencies
(for Gradle). This will show you a list of all the dependencies included in your project.
With these steps complete, you should now have the necessary Mockito dependency for Java 17 set up in your project. Happy testing!