what is \\\\\\\\\\\\\\\\s in java
what is s in java
I apologize for the mistake earlier!
Now, let's talk about in Java.
In Java, (backslash) has multiple meanings depending on its context. Here are some common scenarios where you'll encounter it:
File paths and directories: In a file path or directory path, the backslash (
) is used as an escape character to indicate that the following character is special or has a specific meaning.
Example: C:UsersusernameDocumentsfile.txt
In this case, helps Java recognize the drive letter (C:), folder names (Users, username, Documents), and file name (file.txt).
Example: Pattern p = Pattern.compile("food+");
Here, is used to escape the digit (
d
) so it's treated as a literal character instead of a regular expression pattern.
"
: represents a double quote (") n
: represents a newline r
: represents a carriage return t
: represents a tab
Example: "Hello "goodbye""
or "Line 1nLine 2"
"""
) to create strings without having to escape special characters.
Example: String s = """This is a multi-line string.""";
In this case, the backslash isn't needed because the string literal uses the triple quotes ("""
).
Example: <tag attr="value " escaped ">
Here, is used to represent special characters like the double quote (") within the attribute value.
Example: String s = "Hello" + "nWorld";
However, this practice is generally discouraged and replaced by using the +
operator or more modern string manipulation techniques.
In summary, in Java can have different meanings depending on its context:
In file paths, it's used to indicate special characters. In regular expressions, it's an escape character for special patterns. In string literals, it's used to represent special characters like quotes, newlines, or tabs. In raw string literals (Java 15+), the backslash isn't needed. In XML or HTML parsing, it can be used as an escape character within attribute values or CDATA sections.Remember to use judiciously and only where necessary in your Java code.
What does %d mean in Java?
I'll respond in English as per your request!
In Java, %d
is a format specifier used in the String.format()
or System.out.printf()
methods to convert a value to a decimal integer.
The %d
format specifier is part of a formatting string, which is used to specify how to convert a value into a string. The %d
specifier tells Java to treat the next argument as an int
value and convert it to a decimal integer in a human-readable form.
Here's a breakdown of what %d
does:
%d
takes an int
value as its argument and converts it to a string representation of that integer. Decimal formatting: The resulting string is formatted as a decimal integer, using the standard notation for integers (e.g., 12345).
When you use %d
in a format string, Java will replace this specifier with the converted integer value. For example:
int myInt = 123;
System.out.printf("The number is: %d%n", myInt);
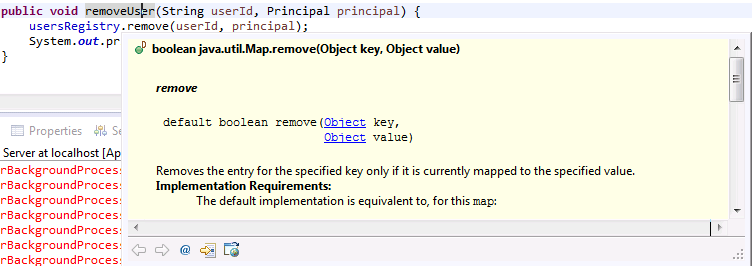
Output:
The number is: 123
In this example, %d
is replaced with the string representation of the myInt
variable (which has a value of 123).
Now, let's explore some additional aspects of %d
:
%d
specifier. For example: Width: %5d
would left-pad the integer with spaces to a minimum width of 5 characters. Precision: %.2d
would display the integer with two digits (e.g., 123 becomes 120). Multiple formats: You can use %d
in combination with other format specifiers, such as %s
for strings or %f
for floating-point numbers. For instance:
int myInt = 123;
String myStr = "Hello!";
System.out.printf("The number is: %d and the string is: %s%n", myInt, myStr);
Output:
The number is: 123 and the string is: Hello!
In summary, %d
in Java is a format specifier that converts an int
value to a decimal integer string. It's a fundamental building block for formatting strings and can be combined with other specifiers to create complex formatting patterns.