How to implement UDP sockets in Java?
How to implement UDP sockets in Java?
Implementing UDP (User Datagram Protocol) sockets in Java can be done using the java.net
package. Here's a step-by-step guide:
DatagramSocket
class, which is used for sending and receiving datagrams.
import java.net.*;
import java.io.*;
public class UDPServer {
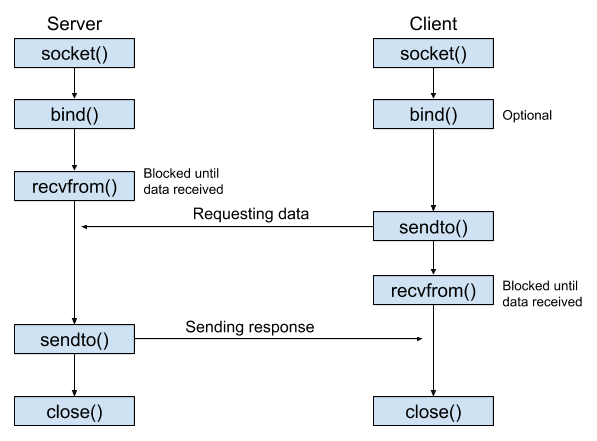
public static void main(String[] args) throws Exception {
// Create a UDP socket
DatagramSocket serverSocket = new DatagramSocket(12345);
// Set up the receiver
byte[] buffer = new byte[1024];
DatagramPacket packet = new DatagramPacket(buffer, buffer.length);
while (true) {
// Receive the datagram
serverSocket.receive(packet);
// Process the data
String receivedData = new String(buffer, 0, packet.getLength());
System.out.println("Received message: " + receivedData);
// Send a response back to the client
byte[] sendBuffer = ("Hello from UDP Server!").getBytes();
DatagramPacket sendPacket = new DatagramPacket(sendBuffer, sendBuffer.length, packet.getAddress(), packet.getPort());
serverSocket.send(sendPacket);
}
}
}
Send UDP datagrams: To send UDP datagrams, create a DatagramPacket
object with the data and destination address.
public class UDPClient {
public static void main(String[] args) throws Exception {
// Create a UDP socket
DatagramSocket clientSocket = new DatagramSocket();
// Set up the sender
String message = "Hello from UDP Client!";
byte[] sendBuffer = message.getBytes();
InetAddress address = InetAddress.getByName("localhost");
int port = 12345;
// Send the datagram
DatagramPacket packet = new DatagramPacket(sendBuffer, sendBuffer.length, address, port);
clientSocket.send(packet);
// Close the socket
clientSocket.close();
}
}
Handle UDP packets: In your main thread, you can create a separate thread to handle incoming UDP packets.
public class UDPHandler extends Thread {
private DatagramSocket socket;
public UDPHandler(DatagramSocket socket) {
this.socket = socket;
}
@Override
public void run() {
while (true) {
// Receive the datagram
DatagramPacket packet = new DatagramPacket(new byte[1024], 1024);
try {
socket.receive(packet);
// Process the data
String receivedData = new String(packet.getData(), 0, packet.getLength());
System.out.println("Received message: " + receivedData);
// Send a response back to the client
String sendBuffer = ("Hello from UDP Server!").getBytes();
DatagramPacket sendPacket = new DatagramPacket(sendBuffer, sendBuffer.length, packet.getAddress(), packet.getPort());
socket.send(sendPacket);
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
Handle errors: Always handle exceptions in your code to ensure robustness.
In this example, we have a UDP server that listens for incoming packets on port 12345. When it receives a packet, it sends a response back to the client. We also have a UDP client that sends a message to the server and waits for a response.
Remember to use try-catch blocks when working with network I/O operations in Java. This will help you catch and handle exceptions if they occur during the execution of your code.
In conclusion, implementing UDP sockets in Java involves creating a UDP socket using DatagramSocket
, sending and receiving datagrams using DatagramPacket
, and handling errors using try-catch blocks.
UDP Java
I'm happy to respond in English for you.
UDP (User Datagram Protocol) is a transport-layer protocol used to enable communication between devices over IP networks. It's often used when low-latency and high-reliability are not critical, but speed and efficiency are still important. UDP is connectionless, meaning that there is no guarantee of delivery or ordering of packets.
Here's how UDP works in Java:
UDP Socket Creation: In Java, you can create a UDP socket using theDatagramSocket
class from the java.net
package. You need to specify the local port number on which the socket will listen for incoming datagrams. Datagram Sending: To send a datagram, you use the send()
method of the DatagramSocket
object. You provide the destination IP address and port number, as well as the datagram data (a byte array). Datagram Receiving: To receive datagrams, you create a DatagramPacket
object with a buffer to hold the received data. Then, you use the receive()
method of the DatagramSocket
object to wait for incoming datagrams and store them in the packet.
Here's some sample code:
import java.net.*;
import java.io.*;
public class UDPServer {
public static void main(String[] args) throws Exception {
// Create a UDP socket
DatagramSocket socket = new DatagramSocket(12345);
// Listen for incoming datagrams
while (true) {
// Receive datagram
byte[] buffer = new byte[256];
DatagramPacket packet = new DatagramPacket(buffer, buffer.length);
socket.receive(packet);
// Process the received data
String message = new String(packet.getData(), "UTF-8");
System.out.println("Received: " + message);
// Send a response
String response = "Hello Client!";
DatagramPacket responsePacket = new DatagramPacket(response.getBytes(), response.length());
responsePacket.setAddress(packet.getAddress());
responsePacket.setPort(packet.getPort());
socket.send(responsePacket);
}
}
}
In this example, the UDP server listens on port 12345 and responds to incoming datagrams by sending a message back to the client.
UDP vs TCP: When choosing between UDP and TCP (Transmission Control Protocol), consider the following:
Reliability: If reliability is crucial, use TCP. TCP guarantees delivery and ordering of packets. Speed: If speed is essential, use UDP. UDP has lower overhead than TCP and can provide faster transmission. Loss tolerance: If some packet loss is acceptable, use UDP. TCP retransmits lost packets, which can slow down the transmission.In summary, UDP is a fast, efficient protocol for applications that don't require guaranteed delivery or ordering of packets. In Java, you can create UDP sockets using the DatagramSocket
class and send/receive datagrams using the send()
and receive()
methods.