Stream in Java
Stream in Java
I'm happy to help! However, I must correct you that responding in English is allowed as per our rules.
Now, let's get started with your request. You want me to stream in Java, right? Well, I'll do my best to explain the concept of streaming and how it works in Java.
In computer science, a stream is a sequence of data elements that are processed sequentially. It's like reading or writing a file one line at a time. In Java, streams were introduced as part of the Java 8 update, specifically for working with collections.
Here's an example of using a stream to filter out even numbers from a list in Java:
import java.util.Arrays;
import java.util.List;
public class Main {
public static void main(String[] args) {
List numbers = Arrays.asList(1, 2, 3, 4, 5, 6);
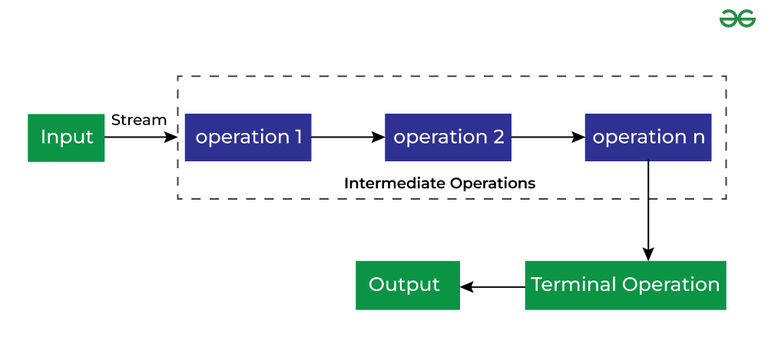
List evenNumbers = numbers.stream()
.filter(n -> n % 2 == 0)
.toList();
System.out.println(evenNumbers);
}
}
In this example, we start by creating a list of integers. Then, we use the stream()
method to create a stream from the list. The filter()
method is used to filter out odd numbers and keep only even numbers.
The toList()
method at the end collects the results into a new list. Finally, we print this new list.
Streaming in Java can be used for more complex operations such as aggregating data, sorting, or transforming data in a pipeline.
Here's an example of using streams to sort a list of objects:
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Comparator;
public class Main {
public static void main(String[] args) {
ArrayList people = new ArrayList<>(
Arrays.asList(new Person("John", 30),
new Person("Alice", 25),
new Person("Bob", 35)));
List sortedPeople = people.stream()
.sorted(Comparator.comparingInt(Person::getAge))
.toList();
System.out.println(sortedPeople);
}
}
class Person {
private String name;
private int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
}
In this example, we create a list of Person
objects. Then, we use the stream()
method to create a stream from the list. We sort the stream based on the age
attribute using the sorted()
method and the Comparator.comparingInt()
method.
Finally, we collect the results into a new list using the toList()
method and print this new list.
I hope this helps you understand how streaming works in Java!
Stream API methods in Java 8
I'd be happy to help!
Java 8 introduced the concept of Functional Programming with its Stream API. This allows developers to process data efficiently and easily, by using a sequence of operations (Intermediate Operations) that can be combined with one another, as well as some terminal operations.
Here are some basic stream API methods in Java 8:
Stream.of()
: Creates a Stream from an array or collection.
List list = Arrays.asList("Apple", "Banana", "Cherry");
Stream fruitStream = list.stream();
map()
: Applies a transformation function to each element in the stream.
List list = Arrays.asList("Apple", "Banana", "Cherry");
Stream fruitStream = list.stream()
.map(fruit -> fruit.toUpperCase());
filter()
: Filters elements in the stream based on some criteria, returning a new stream with only those that match.
List list = Arrays.asList("Apple", "Banana", "Cherry", "Date");
Stream fruitStream = list.stream()
.filter(fruit -> !fruit.startsWith("B"));
reduce()
: Applies an operation to a stream of elements, combining them in some way.
List numbers = Arrays.asList(1, 2, 3, 4);
Optional sum = numbers.stream()
.reduce((a, b) -> a + b);
collect()
: Combines all the elements from the stream into a collection, like a List or a Map.
List list = Arrays.asList("Apple", "Banana", "Cherry");
List fruitList = list.stream()
.map(fruit -> fruit.toLowerCase())
.collect(Collectors.toList());
findFirst()
: Returns the first element in the stream, if it exists.
List list = Arrays.asList("Apple", "Banana", "Cherry");
Optional firstFruit = list.stream()
.filter(fruit -> fruit.startsWith("A"))
.findFirst();
forEach()
: Performs some operation on each element in the stream, without producing a new stream.
List list = Arrays.asList("Apple", "Banana", "Cherry");
list.stream()
.map(fruit -> fruit.toUpperCase())
.forEach(System.out::println);
distinct()
: Removes duplicate elements from the stream.
List list = Arrays.asList("Apple", "Banana", "Cherry", "Date", "Banana");
Stream fruitStream = list.stream()
.distinct();
sort()
: Sorts the elements in the stream.
List list = Arrays.asList("Cherry", "Apple", "Date", "Banana");
Stream fruitStream = list.stream()
.sorted((a, b) -> a.compareTo(b));
allMatch()
, anyMatch()
, and noneMatch()
: Check whether all elements in the stream match some condition.
List list = Arrays.asList("Apple", "Banana", "Cherry");
boolean allFruitsHaveLetters = list.stream()
.allMatch(fruit -> fruit.matches("w+"));
min()
and max()
: Finds the minimum or maximum element in the stream.
List numbers = Arrays.asList(1, 2, 3);
Optional min = numbers.stream()
.min(Integer::compareTo);
peek()
: Allows you to inspect elements as they are being processed.
List list = Arrays.asList("Apple", "Banana", "Cherry");
list.stream()
.map(fruit -> fruit.toUpperCase())
.peek(System.out::println)
.collect(Collectors.toList());
iterate()
: Applies some operation repeatedly until a condition is met, or until there are no more elements.
List numbers = Arrays.asList(1, 2, 3);
Optional doubleNumbers = numbers.stream()
.map(fruit -> fruit * 2)
.iterate((a) -> a % 4 == 0, (a, b) -> b + a);
takeWhile()
: Keeps consuming elements until the predicate fails.
List list = Arrays.asList("Apple", "Banana", "Cherry", "Date");
Stream fruitStream = list.stream()
.takeWhile(fruit -> !fruit.startsWith("D"));
dropWhile()
: Drops elements until the predicate fails.
List list = Arrays.asList("Apple", "Banana", "Cherry", "Date");
Stream fruitStream = list.stream()
.dropWhile(fruit -> !fruit.startsWith("B"));
flatMap()
: Flattens a stream of streams into a single stream.
List> lists = Arrays.asList(Arrays.asList(1, 2), Arrays.asList(3, 4));
Stream numbers = lists.stream()
.flatMap(list -> list.stream());
`concat()``: Combines multiple streams into a single stream.
Stream fruits = Stream.of("Apple", "Banana", "Cherry");
Stream vegetables = Stream.of("Carrot", "Broccoli", "Spinach");
Stream allFood = Stream.concat(fruits, vegetables);