Does Java have shorts?
Does Java have shorts?
I'd be happy to respond in English for you!
To answer your question: "Does Java have short?"
In Java, there are four primitive types that correspond to the basic data types: byte
, short
, int
, and long
.
Among these, short
is indeed a part of Java's standard library. In Java, a short
is an 16-bit signed integer. This means it can hold values ranging from -2^15 (which is -32768) to 2^15-1 (which is 32767).
In Java programming, you can declare and use a short
variable just like any other primitive type:
public class ShortExample {
public static void main(String[] args) {
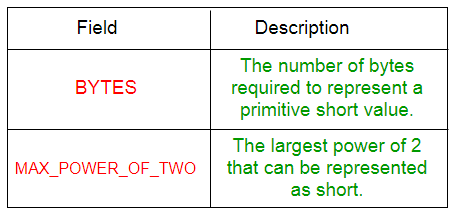
short myShort = 12345; // declaring and initializing a short variable
System.out.println(myShort); // printing the value of the short variable
}
}
When you run this code, it will print 12345
to your console. The type system ensures that only valid values for a short
are assigned or passed as an argument.
The importance of having a short
data type lies in its ability to be used when memory is limited and smaller storage sizes are necessary. This can be particularly useful when dealing with low-memory devices, embedded systems, or other resource-constrained scenarios where space efficiency matters.
It's worth noting that while short
is part of Java's primitive types, it's less commonly used than the more popular integer types like int
and long
. Nonetheless, understanding and working with the short
data type can still be valuable in specific programming contexts.
How to use short type in Java?
I'll respond in English for this question.
Short in Java is a primitive data type that represents a 16-bit signed integer. It has a range of -32768 to 32767. Short values are not always as compact, but they are generally faster and more efficient than integers because they only use 2 bytes (16 bits) instead of the default 4 bytes for an integer.
Here is how you can use short in Java:
public class Main {
public static void main(String[] args) {
// Declare and initialize a short variable
short myShort = 123;
// Print the value of the short variable
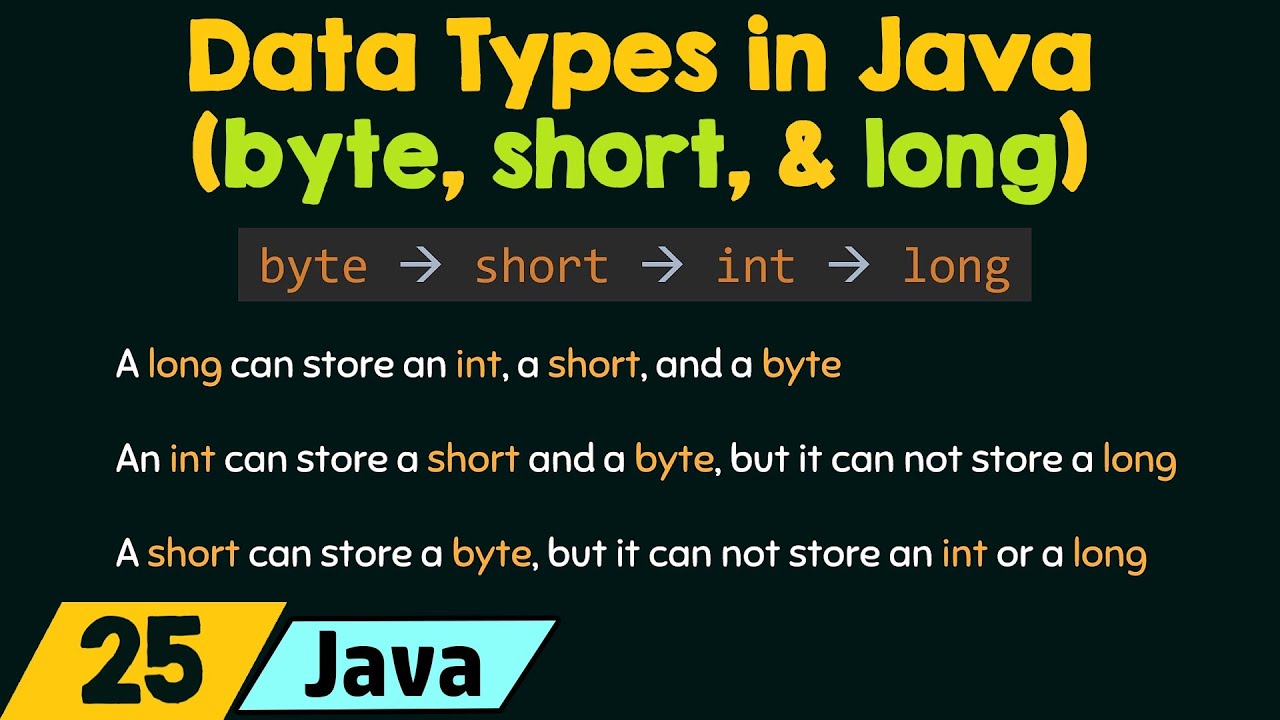
System.out.println("The value of myShort is: " + myShort);
// Convert int to short
int myInt = 456;
short myShort2 = (short)myInt; // explicit casting from int to short
// Print the value of myShort2
System.out.println("The value of myShort2 is: " + myShort2);
}
}
In this example, we first declare and initialize a short
variable named myShort
. We then print its value. Next, we convert an int
to a short
using explicit casting.
Conversion between short and other data types:
Java provides several ways to convert the short
type to other data types:
short
to other data types like int
, long
, or even char
. For example:
short myShort = 123;
int myInt = (int)myShort; // explicit casting from short to int
Autoboxing and Unboxing: Java also supports autoboxing (converting a primitive type to its wrapper class) and unboxing (converting a wrapper class back to its primitive type). For example:
short myShort = 123;
Integer myInt = myShort; // autoboxing from short to Integer
int result = myInt; // unboxing from Integer to int
Wrappers classes: You can also use the wrapper class Short
(in this case) and its methods to perform conversions:
short myShort = 123;
Integer myInt = Short.valueOf(myShort); // convert short to Integer
int result = myInt; // unboxing from Integer to int
Remember that when you perform a conversion, it's possible that the resulting value might lose precision or get truncated (e.g., when converting an int
to a short
). Always verify the range of values and be aware of any potential loss of information.
This concludes our discussion on using short in Java.