How to send data from server to client in socket programming in Java?
How to send data from server to client in socket programming in Java?
I'm happy to help!
In Java socket programming, sending data from the server to the client involves several steps:
Creating a Socket: First, create a socket on both the server and the client. The server creates a server socket (ServerSocket), while the client creates a client socket (Socket). The server socket listens for incoming connections, whereas the client socket connects to the server.// Server-side:
ServerSocket serverSocket = new ServerSocket(8000);
Socket clientSocket = serverSocket.accept();
// Client-side:
Socket clientSocket = new Socket("localhost", 8000);
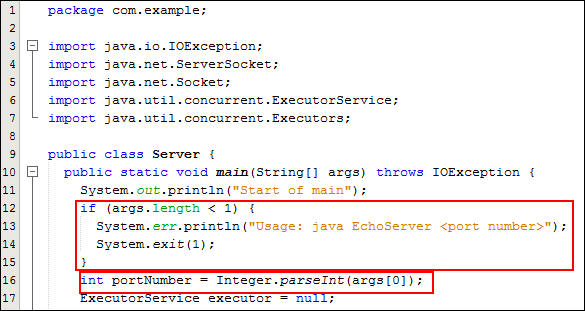
// Server-side:
InputStream in = clientSocket.getInputStream();
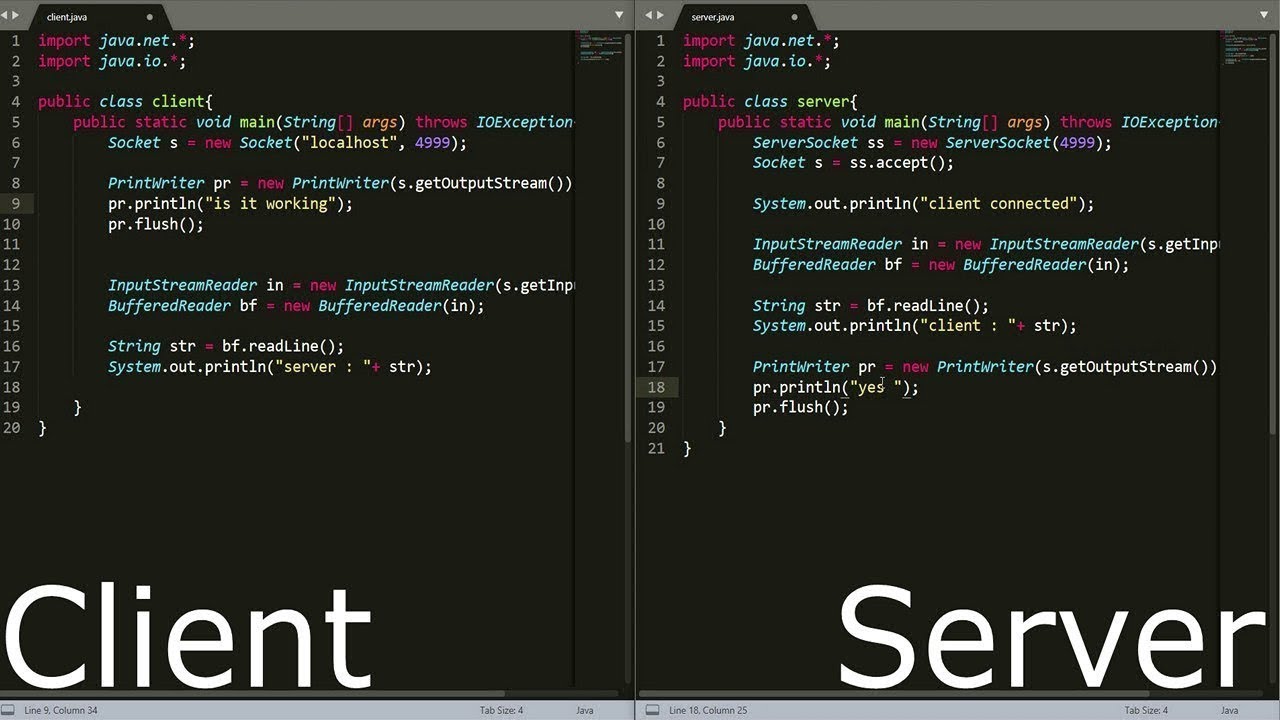
OutputStream out = clientSocket.getOutputStream();
// Client-side:
InputStream in = clientSocket.getInputStream();
OutputStream out = clientSocket.getOutputStream();
Sending Data: On the server side, write the data to be sent into the output stream using a DataOutputStream
. On the client side, read the data from the input stream using a BufferedReader
.
// Server-side:
DataOutputStream dos = new DataOutputStream(out);
dos.writeUTF("Hello Client!");
dos.close();
// Client-side:
BufferedReader reader = new BufferedReader(new InputStreamReader(in));
String message = reader.readLine();
reader.close();
Closing Sockets: Finally, close the sockets to free up system resources:
clientSocket.close();
serverSocket.close();
Here is a complete example of sending data from server to client in Java socket programming:
import java.io.*;
import java.net.*;
public class SocketServer {
public static void main(String[] args) throws IOException {
ServerSocket serverSocket = new ServerSocket(8000);
System.out.println("Server started. Listening for incoming connections...");
Socket clientSocket = serverSocket.accept();
System.out.println("Connection established with " + clientSocket.getInetAddress());
BufferedReader reader = new BufferedReader(new InputStreamReader(clientSocket.getInputStream()));
DataOutputStream dos = new DataOutputStream(clientSocket.getOutputStream());
String message = "Hello Client!";
dos.writeUTF(message);
dos.close();
System.out.println("Sent message: " + message);
reader.close();
clientSocket.close();
}
}
import java.io.*;
import java.net.*;
public class SocketClient {
public static void main(String[] args) throws IOException {
Socket clientSocket = new Socket("localhost", 8000);
System.out.println("Connected to server");
BufferedReader reader = new BufferedReader(new InputStreamReader(clientSocket.getInputStream()));
String message = reader.readLine();
System.out.println("Received message: " + message);
reader.close();
clientSocket.close();
}
}
This example demonstrates a simple text-based communication between the server and client. The server sends a predefined message to the client, which reads and prints it.
Remember that this is just a basic example of socket programming in Java, and you may need to add error handling, implement more advanced protocols, or use additional libraries depending on your specific requirements.
Socket programming in Java PDF
Here is a comprehensive guide to socket programming in Java in the form of a PDF:
Socket Programming in Java: A Comprehensive Guide
Introduction
Socket programming is an essential part of network communication, allowing programs to communicate with each other over a network. This tutorial provides a step-by-step guide on how to create socket programming in Java.
What are Sockets?
Sockets are endpoints for communication between two devices (computer, phone, etc.) in a network. They provide a way for different processes (programs) to communicate with each other. A socket is like an end point where the program can read from or write to.
Types of Sockets
There are two types of sockets:
Stream-oriented Socket: This type of socket allows data to be sent and received as streams of bytes. Datagram-oriented Socket (also known as UDP Socket): This type of socket sends and receives datagrams, which are packets of data with a maximum size.Creating Sockets in Java
To create sockets in Java, we use the java.net
package, specifically the Socket
, ServerSocket
, DatagramPacket
, and DatagramSocket
classes.
Client Side (Sender)
Import necessary packages:import java.net.*;
Create a new socket: Socket client = new Socket("serverHost", portNumber);
Send data over the socket: client.getOutputStream().write(data);
Close the socket: client.close();
Server Side (Receiver)
Import necessary packages:import java.net.*;
Create a new server socket: ServerSocket server = new ServerSocket(portNumber);
Listen for incoming connections: Socket client = server.accept();
Receive data from the client: byte[] receivedData = new byte[1024]; client.getInputStream().read(receivedData);
Close the socket: client.close();
Datagram Sockets (UDP)
Import necessary packages:import java.net.*;
Create a new datagram socket: DatagramSocket dgramSock = new DatagramSocket();
Send data over the socket: dgramSock.send(new DatagramPacket(data, length));
Receive data from the client: DatagramPacket receivedData = new DatagramPacket(receivedData, receivedData.length);
Close the socket: dgramSock.close();
Best Practices
Always close sockets after use to prevent resource leaks. Handle exceptions and errors properly to ensure robustness. Use thread pools for concurrent processing.Conclusion
In this comprehensive guide, we have learned how to create socket programming in Java using stream-oriented and datagram-oriented sockets. By following the best practices and handling exceptions, you can create reliable and efficient network communication programs in Java.
PDF: Socket Programming in Java
Please note that this is a basic guide and you may want to consider advanced topics such as socket options, non-blocking sockets, and multiplexing.