How many types of scopes are there in Java?
How many types of scopes are there in Java?
I'd be happy to respond in English for this question.
In Java, a scope refers to the region of the code where a variable is accessible. The type of scope in Java can be classified into several categories:
Package Scope: A package-private variable or method is only accessible within its declaring class and any classes that inherit from it within the same package. This is denoted by the default (no modifier) access specifier.public class Myclass {
int x; // has package scope
public static void main(String[] args) {
Myclass my = new Myclass();
System.out.println(my.x); // ok, because it's in the same package
}
}
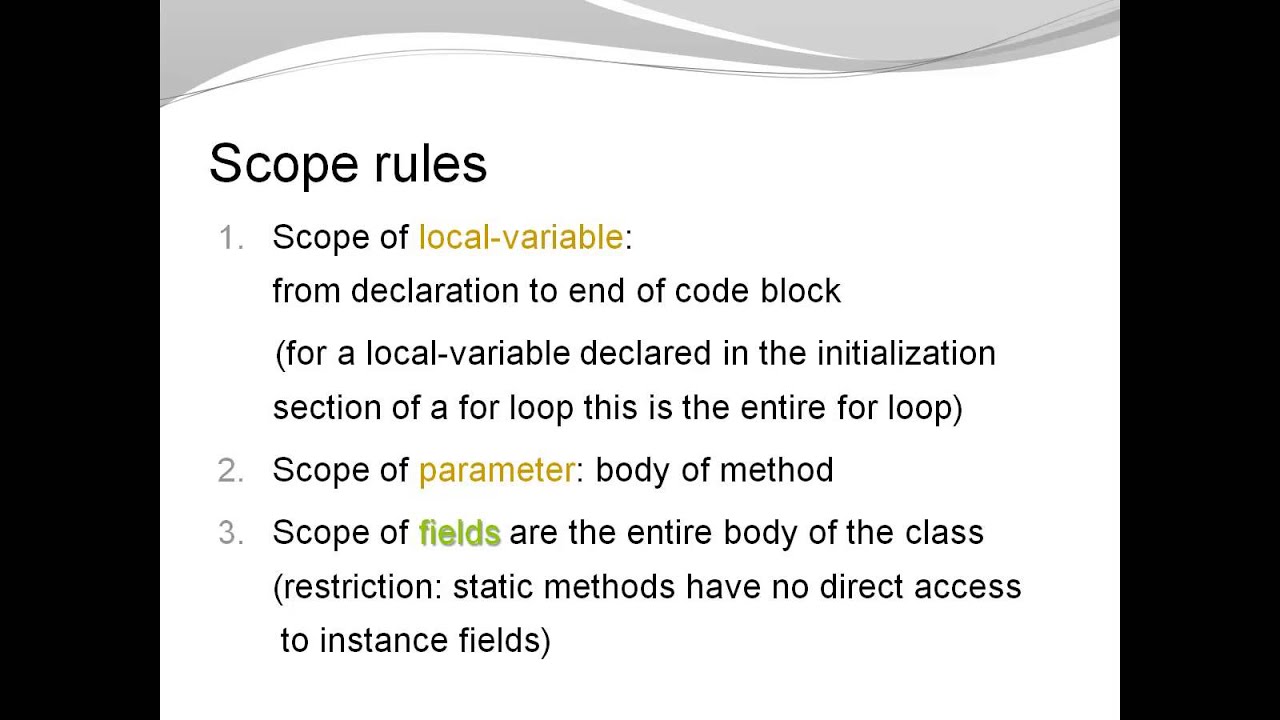
Public Scope: A public variable or method can be accessed from any part of the program, whether within the same class or outside.
public class MyClass {
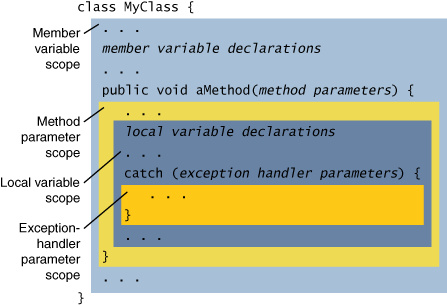
public int x; // has public scope
public static void main(String[] args) {
MyClass my = new MyClass();
System.out.println(my.x); // ok, because it's public
}
}
Private Scope: A private variable or method can only be accessed within the same class.
public class MyClass {
private int x; // has private scope
public void display() {
System.out.println(x);
}
public static void main(String[] args) {
MyClass my = new MyClass();
System.out.println(my.x); // error, because it's private
}
}
Protected Scope: A protected variable or method can be accessed within the same class and its subclasses.
public class MyClass {
protected int x; // has protected scope
public void display() {
System.out.println(x);
}
public static void main(String[] args) {
MyClass my = new MyClass();
System.out.println(my.x); // ok, because it's in the same package
}
}
public class MySubClass extends MyClass {
public static void main(String[] args) {
MySubClass submy = new MySubClass();
System.out.println(submy.x); // ok, because it's protected and it's a subclass
}
}
In summary, the four types of scope in Java are package-private, public, private, and protected. Understanding these scopes is crucial for designing classes, methods, and variables that work together effectively.
Please let me know if you have any further questions or concerns!
How to check the scope of a variable in Java?
I'd be happy to help!
In Java, you can use several methods to determine the scope of a variable:
Using thestatic
keyword: If you declare a variable using the static
keyword, it belongs to the class itself and is accessible within its entire scope. This means that if you have multiple instances of the same class, they will all share the same static variables.
Example:
public class MyStaticClass {
public static int myStaticVariable = 0;
public void printStatic() {
System.out.println(myStaticVariable); // prints 0
}
}
Using a block-level scope: Variables declared within a block (such as an if
statement or a loop) are only accessible within that specific block and its inner blocks.
Example:
public class MyBlockClass {
public void myMethod() {
if (true) {
int myLocalVariable = 5; // accessible here
System.out.println(myLocalVariable); // prints 5
}
}
}
Using a method-level scope: Variables declared within a method are only accessible within that specific method and its inner blocks.
Example:
public class MyMethodClass {
public void myMethod() {
int myLocalVariable = 0; // accessible here
System.out.println(myLocalVariable); // prints 0
}
}
Using a lambda scope: Variables declared within a lambda expression are only accessible within that specific lambda and its inner blocks.
Example:
public class MyLambdaClass {
public void myMethod() {
IntFunction intFunc = () -> {
int myLocalVariable = 5; // accessible here
System.out.println(myLocalVariable); // prints 5
};
}
}
Using a scope of an anonymous inner class: Variables declared within an anonymous inner class are only accessible within that specific anonymous inner class and its inner blocks.
Example:
public class MyAnonymousClass {
public void myMethod() {
new Runnable() {
@Override
public void run() {
int myLocalVariable = 0; // accessible here
System.out.println(myLocalVariable); // prints 0
}
};
}
}
In summary, Java has several ways to define the scope of a variable:
static
variables have class-level scope and are shared across all instances of the same class. Block-level variables have block-level scope and are only accessible within that specific block and its inner blocks. Method-level variables have method-level scope and are only accessible within that specific method and its inner blocks. Lambda variables have lambda scope and are only accessible within that specific lambda and its inner blocks. Anonymous inner class variables have the scope of their parent class, which is the anonymous inner class itself.
I hope this helps!