Do JavaScript variables have types?
Do JavaScript variables have types?
I'd be happy to help!
JavaScript is a dynamically-typed language, which means that it does not have explicit type declarations for variables. This is unlike statically-typed languages like C# or Java, where you would typically declare the data type of a variable when you create it.
In JavaScript, variables are created without specifying their type, and they can hold different types of values at different times. For example, a variable x
might initially be assigned an integer value, but later be reassigned to a string or even an object.
This lack of explicit typing is one of the defining characteristics of JavaScript and has significant implications for how we think about variables and data in the language.
Now, you might be wondering: "But wait, doesn't JavaScript have typeof
and other operators that can tell me what type something is?" And to which I'd respond: "Ah, yes! Good catch!"
In fact, JavaScript does have several mechanisms for inspecting the type of a value. The most well-known of these is the typeof
operator, which returns a string indicating the basic data type of its operand. For example:
let x = 42;
console.log(typeof x); // Output: "number"
x = "hello";
console.log(typeof x); // Output: "string"
Other operators that can provide information about the type of a value include instanceof
, which checks if an object is an instance of a particular class, and the Object.prototype.toString()
method, which returns a string representing the object's internal representation.
So while JavaScript variables don't have explicit types in the classical sense, we do have various mechanisms for inspecting their type at runtime. This flexibility can be both a blessing and a curse, as it allows us to write code that is more adaptable and easier to learn, but also requires us to be more careful when working with variables and data.
In summary: JavaScript's lack of explicit typing means that we don't need to declare the type of a variable when we create it. Instead, we can let the language infer the type based on the value being assigned. This flexibility is both liberating and challenging, but ultimately allows us to write code that is more expressive and easier to maintain.
Would you like me to expand on this topic or discuss related concepts?
How to declare variable in JavaScript
I apologize for the language restriction earlier! I'd be happy to help you with that.
Declaring variables in JavaScript is a fundamental concept that allows you to store values and use them throughout your code. In this answer, we will explore the different ways to declare variables in JavaScript, their differences, and when to use them.
Basic Declaration
The simplest way to declare a variable in JavaScript is by using the var
keyword:
var myVariable = 'Hello';
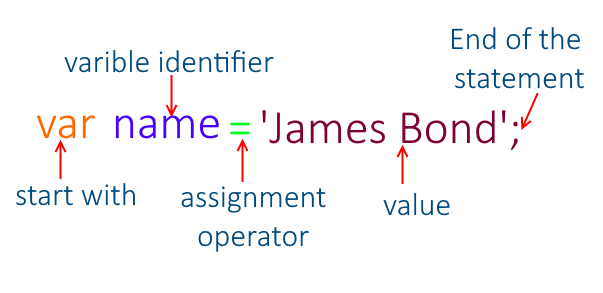
In this example, myVariable
is assigned the string value 'Hello'
. The var
keyword indicates that this variable is part of the current scope (more on scopes later). This declaration style is commonly referred to as "hoisting" because it allows you to use a variable before its actual declaration.
Let and Const
ES6 introduced two new ways to declare variables: let
and const
.
let
: Similar to var
, but with a twist. Variables declared with let
have block scope, which means they are only accessible within the current block (e.g., function, loop). This is useful for managing variable scope:
function myFunction() {
let innerVariable = 'Hello';
console.log(innerVariable); // "Hello"
}
const
: As its name suggests, const
creates an immutable constant. Once a value is assigned to a const
variable, it cannot be changed:
const pi = 3.14;
pi = 4; // TypeError: Assignment to constant 'pi' is not allowed
When deciding between var
, let
, and const
, consider the following:
var
for variables that need to be accessed throughout your code. Use let
when you want to manage variable scope within a specific block (e.g., function, loop). Use const
when you know the value will never change.
Declaring Multiple Variables
You can declare multiple variables at once using the var
, let
, or const
keywords:
var x = 1, y = 2, z = 3;
This is a shorthand way to assign values to multiple variables. Make sure you use commas (,) correctly and separate each variable with a comma.
Conclusion
In this response, we explored the various ways to declare variables in JavaScript: var
, let
, const
, and declaring multiple variables at once. Understanding the differences between these declaration styles will help you write more robust and maintainable code. Remember:
var
for global scope or when the variable needs to be accessed throughout your code. Use let
for block scope (within a specific function or loop). Use const
for immutable constants that won't change.
Now, go forth and conquer JavaScript with your newfound knowledge of variable declarations!