What is a Java RMI server?
What is a Java RMI server?
I'll respond in English for this one!
A Java Remote Method Invocation (RMI) server is a type of distributed system that allows you to invoke methods on remote objects, such as servers or services, from your local program. In essence, it enables you to access and utilize resources located on other machines as if they were locally available.
In the Java programming language, RMI is a standard API (Application Programming Interface) for building distributed systems. It provides a way for Java programs to interact with each other over a network, regardless of their location or platform.
To illustrate this concept, imagine you have two Java programs: one on your local machine and another running on a remote server. Using RMI, the local program can invoke methods on the remote object, just as if it were sitting next to it on your desktop. This enables seamless communication between distributed systems and facilitates collaboration, data exchange, or even resource sharing.
Here's how it typically works:
Remote Object Registration: The remote server creates a Java RMI server (also known as an activator) that registers the remote objects you want to access. This activator provides information about the remote object, such as its name and the network location where it resides. Client Side: On your local machine, your program (the client) uses the RMI API to create a connection to the remote server's activator. This is done by looking up the activator using the RMI registry or by specifying the activator's URL directly. Method Invocation: Once connected, you can invoke methods on the registered remote objects using RMI's proxy mechanism. The client sends method invocations (requests) to the server, which then executes them and returns any resulting data back to your local program. Data Exchange: Data is exchanged between systems as part of the method invocation process. This exchange can occur in various formats, such as serialized Java objects or simple primitives like strings and integers.Java RMI servers are useful for:
Distributed computing: Enable complex computations or data processing tasks to be performed on remote machines. Service-oriented architecture (SOA): Provide access to web services or other network-based resources from your local application. Cloud computing: Facilitate interactions between cloud-based services and your local program.Some popular examples of RMI servers include:
Java Servlets: Allow you to create dynamic web applications that can interact with each other and external systems. Web Services (WS): Provide a standard way for different programming languages and platforms to communicate over the internet using XML-based protocols like SOAP or RESTful APIs.In summary, a Java RMI server is a crucial component of distributed computing, enabling local programs to access and utilize resources located on remote machines. It simplifies interactions between distributed systems and facilitates collaboration, data exchange, or resource sharing in a wide range of applications.
Java rmi server w3schools
I can respond to your request for a Java RMI (Remote Method Invocation) server using W3Schools as the reference material.
RMI is a technology developed by Sun Microsystems that allows you to make method calls on objects running in different JREs (Java Runtime Environments) over the network. It's an essential part of Java programming, enabling distributed applications and remote object invocation.
To create an RMI server using W3Schools as reference, we'll go through a step-by-step guide:
Step 1: Create a Remote Interface
The first thing to do is create an interface that extends the java.rmi.Remote
interface. In this example, let's name it MyRemoteInterface
.
import java.rmi.*;
public interface MyRemoteInterface extends Remote {
String sayHello(String name) throws RemoteException;
}
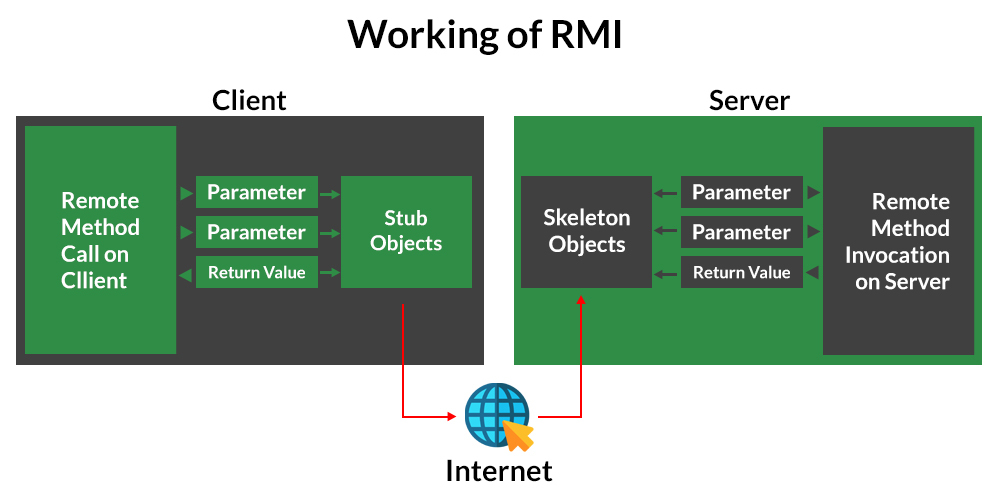
Step 2: Implement the Remote Interface
Next, create a class that implements our remote interface. This class will be responsible for providing the implementation of our remote method.
import java.rmi.*;
import java.rmi.server.*;
public class MyRemoteServer extends UnicastRemoteObject implements MyRemoteInterface {
public MyRemoteServer() throws RemoteException {
}
@Override
public String sayHello(String name) throws RemoteException {
return "Hello, " + name + "!";
}
public static void main(String[] args) {
try {
// Create an instance of the remote server
MyRemoteServer myRemoteServer = new MyRemoteServer();
// Bind it to a registry (W3Schools: Register the service)
Registry registry = LocateRegistry.getRegistry("localhost", 1099);
registry.rebind("MyRemoteInterface", myRemoteServer);
} catch (Exception e) {
System.err.println("Error creating and registering remote server: " + e.getMessage());
}
}
}
Step 3: Create a Client
To interact with our RMI server, we'll create a client that will invoke the sayHello
method.
import java.rmi.*;
public class MyRemoteClient {
public static void main(String[] args) {
try {
// Locate and access the registry
Registry registry = LocateRegistry.getRegistry("localhost", 1099);
// Get the remote interface from the registry
MyRemoteInterface myRemoteInterface = (MyRemoteInterface) registry.lookup("MyRemoteInterface");
// Call our remote method!
String message = myRemoteInterface.sayHello("John");
System.out.println(message);
} catch (Exception e) {
System.err.println("Error interacting with the RMI server: " + e.getMessage());
}
}
}
When you run the client code, it will establish a connection to the remote server using the registry and then invoke the sayHello
method. The output should be something like: "Hello, John!"
.
That's it! You have now successfully created an RMI server and client in Java, following W3Schools' guidelines.
References:
W3Schools - Java RMI Tutorial (https://www.w3schools.com/java/java_rmi.asp)
Oracle - Remote Method Invocation (RMI) (https://docs.oracle.com/javase/8/docs/api/java.rmi/package-summary.html)