Nested if Java best practices
Nested if Java best practices
I'm happy to respond in English!
Nested If Statements in Java: Best Practices
When it comes to writing code, Java developers often face the challenge of deciding when and how to use nested if statements. In this response, I'll provide you with some best practices for working with nested if statements in Java.
Avoid Deep Nesting
One common mistake is creating too many levels of nesting, which can make your code difficult to read and understand. As a general rule, try to limit the depth of your nested if statements to three levels or less.
Here's an example of how this might look:
if (condition1) {
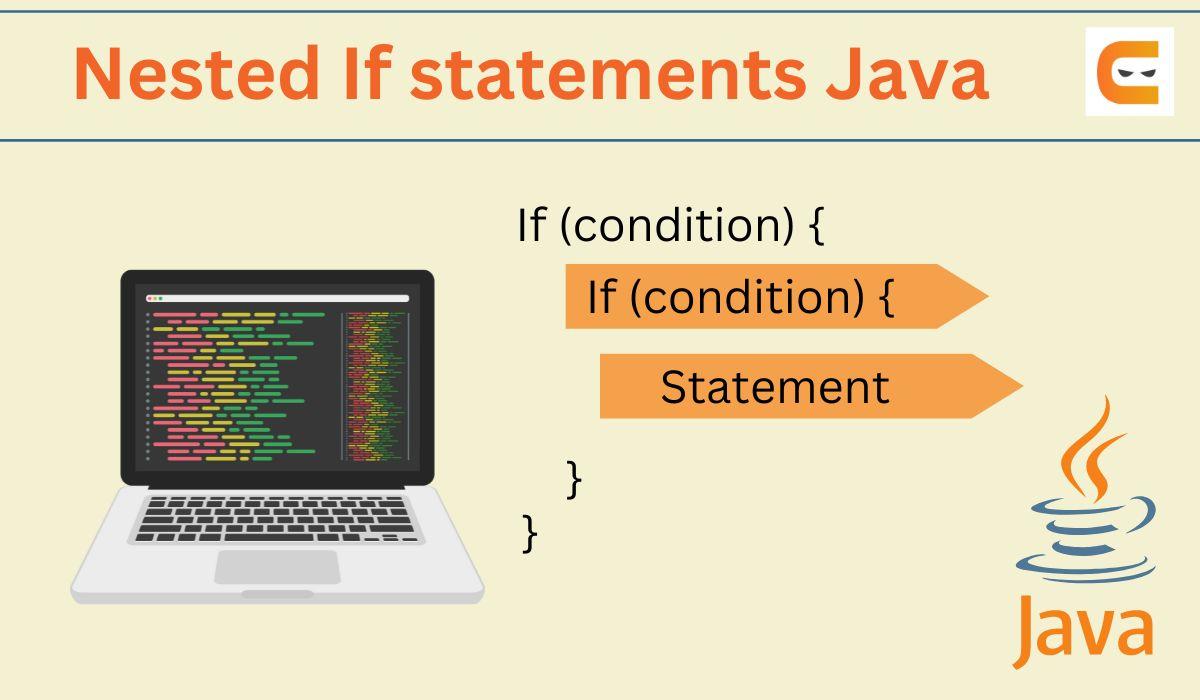
if (condition2) {
if (condition3) {
// Code to execute when all conditions are true
}
}
}
This can be difficult to follow, so it's essential to use whitespace effectively and provide clear comments.
Use Logical Operators
Nested if statements often involve using logical operators (e.g., &&, ||, !) to combine conditional statements. This can lead to complex code that's difficult to maintain. Instead, consider using variables to store the results of intermediate conditions and then combining them using logical operators.
Here's an example:
boolean condition1 = ...;
boolean condition2 = ...;
boolean condition3 = ...;
if (condition1 && condition2 || !condition3) {
// Code to execute when all conditions are true or one condition is false
}
Use Early Returns
When working with nested if statements, it's often helpful to use early returns to simplify your code and reduce the number of nesting levels. This can make your code easier to read and understand.
Here's an example:
if (condition1) {
if (condition2) {
if (condition3) {
return true; // Early return when all conditions are true
}
} else {
return false;
}
}
Use Loops Instead of Nesting
In some cases, you might be able to replace nested if statements with a loop. This can make your code more concise and easier to maintain.
Here's an example:
for (int i = 0; i < array.length; i++) {
if (array[i] > threshold) {
// Code to execute when the condition is true
} else {
break;
}
}
Use Method Extraction
If you find yourself repeatedly using nested if statements in different parts of your code, consider extracting a method that encapsulates this logic. This can make your code more modular and easier to maintain.
Here's an example:
public boolean checkConditions(int value1, int value2) {
if (value1 > threshold1 && value2 < threshold2) {
return true;
} else {
return false;
}
}
// Usage:
if (checkConditions(value1, value2)) {
// Code to execute when the conditions are met
} else {
// Code to execute when the conditions are not met
}
By following these best practices for nested if statements in Java, you can write more readable, maintainable code that's easier to understand.
How to write multiple if-else conditions in Java?
I'd be happy to explain how to write multiple if-else
conditions in Java!
In Java, you can use the traditional if-else
statement to check for multiple conditions and execute different blocks of code accordingly. Here's a step-by-step guide on how to do it:
if
statement:
int age = 25;
if (age >= 18) {
System.out.println("You are an adult.");
}
In this case, the code inside the if
block will only be executed if the condition age >= 18
is true.
if
, else if
, and else
statements to check for different conditions:
int score = 90;
if (score > 95) {
System.out.println("You got an A!");
} else if (score >= 85) {
System.out.println("You got a B.");
} else if (score >= 75) {
System.out.println("You got a C.");
} else {
System.out.println("You need to try harder.");
}
In this example, the code will check for the condition score > 95
first. If it's true, it will print "You got an A!". If not, it will move on to the next condition score >= 85
, and so on. The else
statement is executed only if none of the conditions are met.
int grade = 'A';
if (grade == 'A' || grade == 'B') {
if (student.isHonorsStudent()) {
System.out.println("You're an honors student!");
} else {
System.out.println("You're a regular student.");
}
} else if (grade == 'C' || grade == 'D' || grade == 'F') {
System.out.println("You need to retake the course.");
}
In this example, the code checks for the condition grade == 'A' || grade == 'B'
. If true, it then checks if the student is an honors student. If not, it prints a different message. The subsequent conditions are only checked if the previous one is false.
int temperature = 25;
if (temperature > 20) {
if (isRainy()) {
System.out.println("It's a rainy day!");
} else {
System.out.println("The weather is nice.");
}
} else {
System.out.println("The temperature is too low.");
}
In this example, the code first checks for the condition temperature > 20
. If true, it then evaluates whether it's a rainy day. If not, it prints a different message.
Remember to use proper indentation and formatting to make your code readable! In Java, you can also use switch
statements to handle multiple conditions, but that's a topic for another time.
That's it! I hope this helps you write more efficient and readable code in Java.