What are the four types of variables in java and their
What are the four types of variables in java and their
I'd be happy to help!
In Java, a variable is a named storage location that holds a value of a specific data type. There are four primary types of variables in Java:
Instance Variables: Also known as non-static instance variables or fields, these are variables that belong to an object and can be accessed using the dot operator (i.e.,object.instanceVariable
). Each object created from a class has its own copy of the instance variables, which means each object can have different values for the same variable. For example:
public class Person {
private String name;
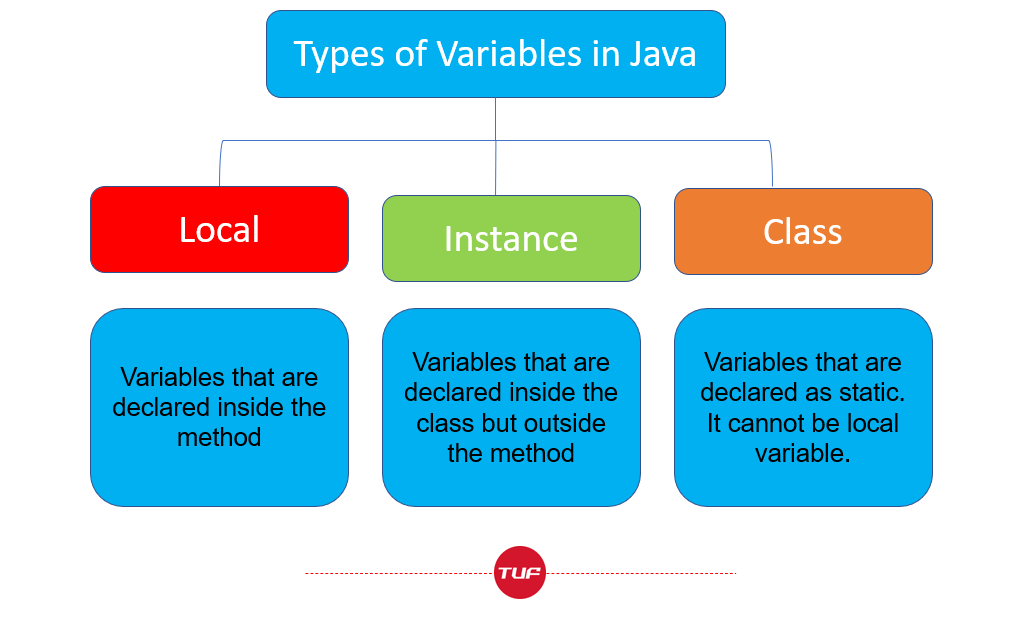
public int age;
// constructor and methods...
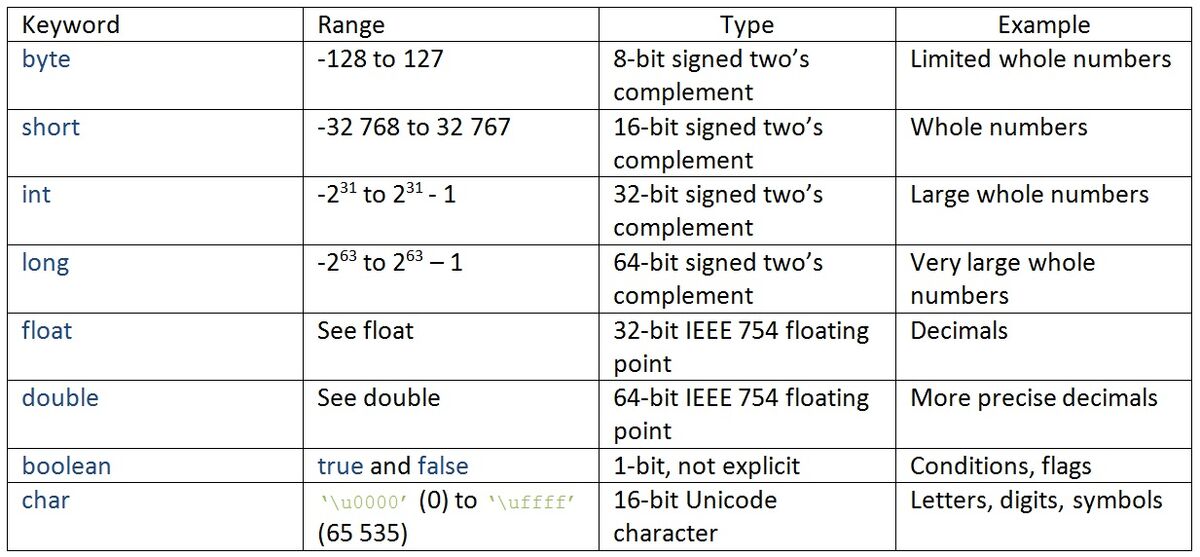
}
Static Variables: Also known as class variables or static fields, these are variables that belong to a class rather than an instance of the class. Static variables are shared by all objects created from the same class, meaning they have the same value for all instances. You access static variables using the class name and the dot operator (i.e., Person.staticVariable
). For example:
public class Person {
public static int population;
// constructor and methods...
}
Local Variables: These are variables declared within a method, block of code, or constructor. Local variables are created when the method or block is entered and destroyed when it's exited. They can be accessed only within the scope where they're declared:
public class Calculator {
public int calculate(int x, int y) {
int result = x + y; // local variable
return result;
}
}
Array Variables: These are variables that hold multiple values of the same data type, such as integers or strings. Array variables are created using the new
keyword and can be accessed using indexing (i.e., array[0]
, array[1]
, etc.). For example:
public class Student {
private String[] subjects = new String[5]; // array variable
public void addSubject(String subject) {
subjects[0] = subject; // accessing the first element of the array
}
}
In conclusion, Java has four primary types of variables: instance variables, static variables, local variables, and array variables. Understanding these differences is crucial for writing effective and efficient Java code!
What are the method variables in Java?
I'm happy to respond in English for you!
In Java, a variable is a name given to a storage location that holds a value of a specific data type. Method variables, also known as local variables or parameters, are the variables defined within a method.
There are three types of method variables:
Method Parameters: These are the variables passed into a method when it's called. They are declared in the method's parameter list and are used to pass information into the method. For example:public void myMethod(int x, String y) {
// Method code here
}
In this example, x
and y
are method parameters.
public void myMethod() {
int z = 10; // Local variable declaration
System.out.println(z); // Accessing local variable
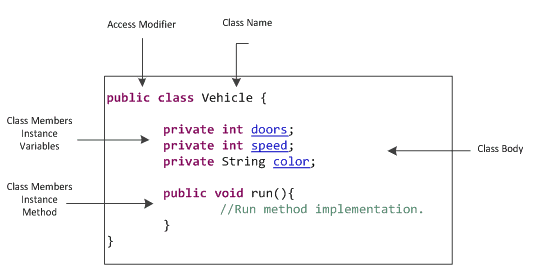
}
In this example, z
is a local variable.
Here's an example:
public void myMethod() {
final int z = 10; // Final variable declaration
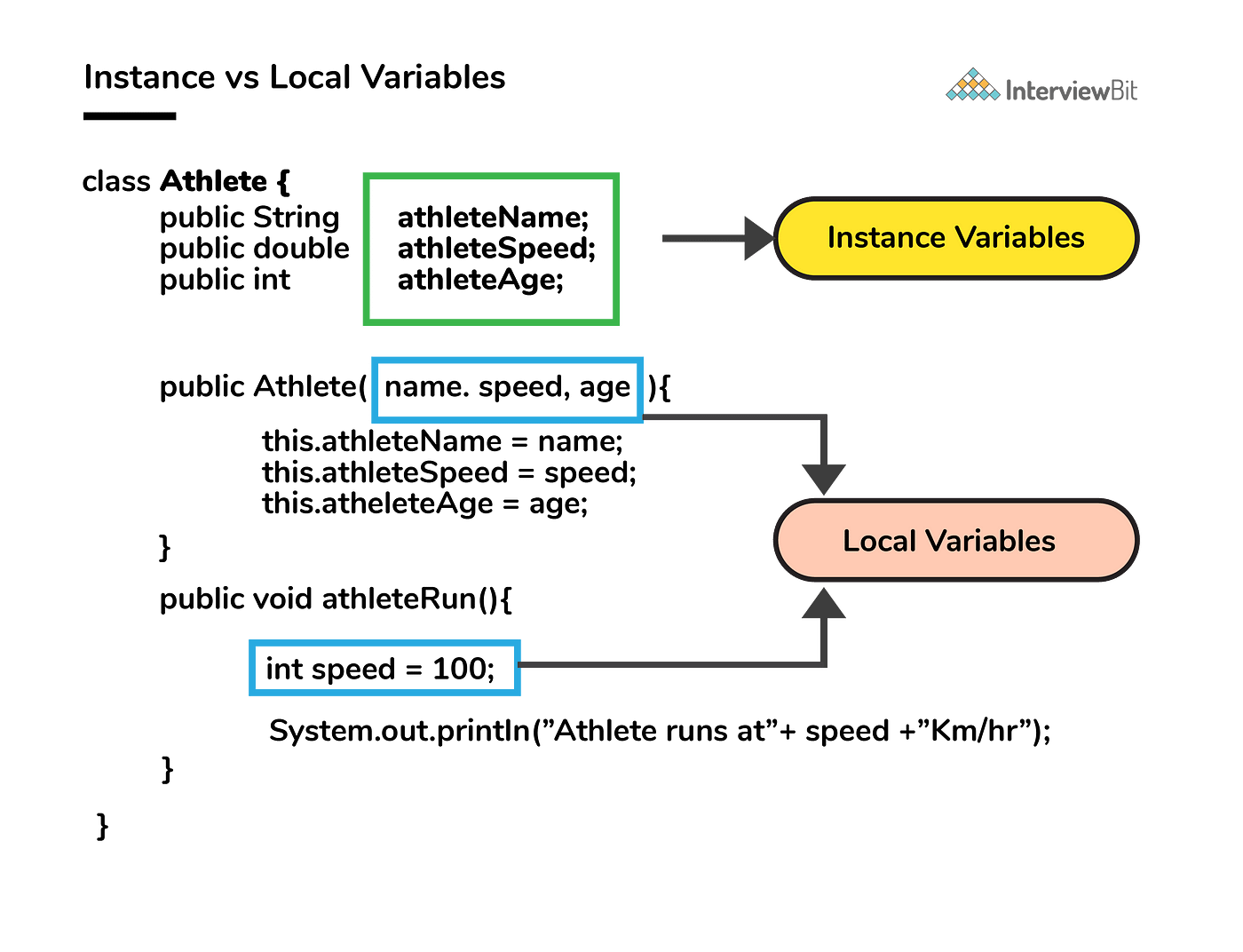
System.out.println(z); // Accessing final variable
}
In this case, z
is a final variable.
Method variables are essential in Java programming as they allow you to manipulate data within a method and control the flow of your program. Understanding how to declare, use, and manipulate these variables is crucial for writing effective and efficient code.
Remember, method variables can be used to pass information into a method, store temporary values within a method, or maintain constant values throughout your program. By mastering the different types of method variables, you'll become more proficient in Java programming and be able to create robust and reliable programs.