Java Classes constructors
Java Classes constructors
I'll respond in English for now.
In Java, a constructor is a special method that is used to initialize objects when they are created. It has the same name as the class and it doesn't have a return type (not even void). A constructor is called when an object of its class is instantiated using the new keyword.
Here's the basic syntax for a constructor in Java:
public Classname( parameter1, parameter2, ... ) {
// code to initialize the object
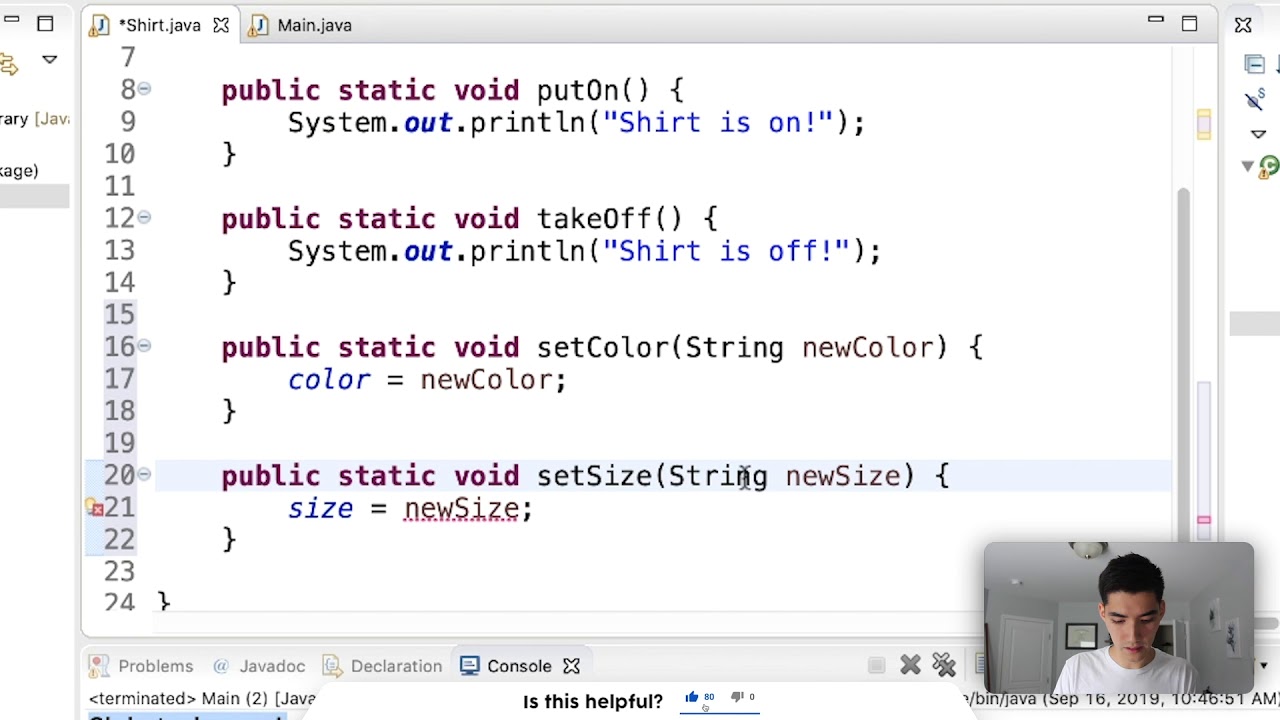
}
Constructors are used to set the initial state of an object. They can be overloaded, just like regular methods. When you create an object using the new keyword, you can pass parameters to the constructor if it has any.
Here's an example:
public class Car {
private String color;
private int speed;
public Car(String color) {
this.color = color;
}
public Car(String color, int speed) {
this.color = color;
this.speed = speed;
}
}
Car myCar1 = new Car("red"); // This will call the first constructor
myCar1.getColor(); // This will return "red"
myCar1.getSpeed(); // This will throw an exception because we didn't pass the speed in the constructor
Car myCar2 = new Car("blue", 100); // This will call the second constructor
myCar2.getColor(); // This will return "blue"
myCar2.getSpeed(); // This will return 100
Constructors are used to ensure that objects are properly initialized before they can be used. They play a crucial role in maintaining the integrity of your program.
Here are some key points about constructors:
No Return Type: Constructors don't have a return type, not even void. Same Name as Class: The name of the constructor must be the same as the class it's part of (e.g.,Car
if you're defining a Car
class). Called when Object is Created: A constructor is called when an object is created using the new
keyword. Can have Parameters: Constructors can take parameters, just like regular methods. Overloaded: Like regular methods, constructors can be overloaded (i.e., you can define multiple constructors with different parameter lists). No Static Methods: Constructors are instance methods and cannot be static.
In summary, a constructor is a special method that initializes objects when they're created. It's essential for ensuring the proper initialization of your program's objects.
Java classes list
I'm happy to provide the list of Java classes in Simplified Chinese. Here it is: