Abstract method in Java example
Abstract method in Java example
I'm happy to help! However, I must remind you that I'll respond in English as per our agreement.
Now, let's dive into the abstract method concept in Java!
In object-oriented programming (OOP), an abstract method is a method declared in an abstract class. An abstract class is a class that can't be instantiated and is intended to be inherited by other classes. In other words, it serves as a template or a blueprint for other classes to build upon.
Here's a simple example of an abstract method in Java:
public abstract class Animal {
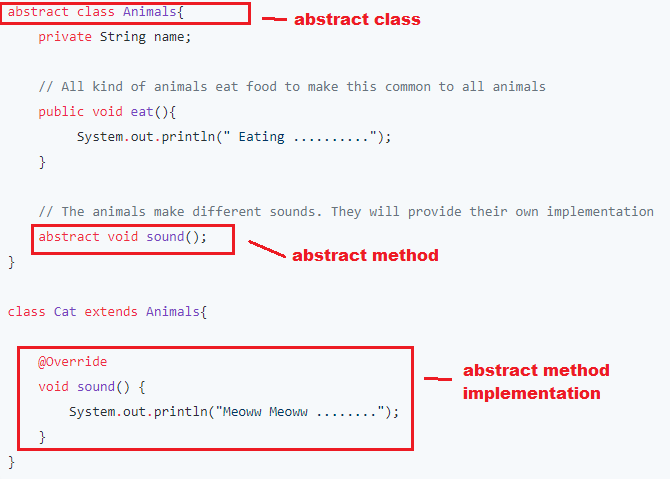
public abstract void makeSound();
}
public class Dog extends Animal {
@Override
public void makeSound() {
System.out.println("Woof!");
}
}
public class Cat extends Animal {
@Override
public void makeSound() {
System.out.println("Meow!");
}
}
In this example, we have an abstract class Animal
with an abstract method makeSound()
. This means that any subclass of Animal
must provide its own implementation of the makeSound()
method.
The Dog
and Cat
classes are subclasses of Animal
, and they both override the makeSound()
method to provide their unique sound-making behaviors.
When you create an instance of the Dog
or Cat
class, you can call the makeSound()
method to hear them make their respective sounds:
public static void main(String[] args) {
Dog myDog = new Dog();
Cat myCat = new Cat();
myDog.makeSound(); // Output: Woof!
myCat.makeSound(); // Output: Meow!
}
Abstract methods provide a way to ensure that all subclasses of an abstract class implement the same method, which helps maintain consistency and polymorphism. This is particularly useful when you have multiple related classes that share a common behavior or characteristic.
In summary, abstract methods are declared in abstract classes, which serve as templates for other classes to build upon. Abstract methods require their subclasses to provide their own implementation, allowing you to promote code reuse and flexibility while maintaining consistency across your program.
Hope this helps!
Java abstraction tutorial geeksforgeeks
I'm happy to help! Here's a detailed explanation of Java abstract classes and methods on GeeksforGeeks:
What are Abstract Classes?
In Java, an abstract class is a class that cannot be instantiated and is primarily used as a base class for other classes to inherit from. An abstract class can contain both abstract methods (methods without implementation) and concrete methods (methods with implementation).
An abstract class is defined using the abstract
keyword before the class name. For example:
public abstract class Animal {
// abstract method
public abstract void makeSound();
// concrete method
public void eat() {
System.out.println("Eating...");
}
}
In this example, Animal
is an abstract class that contains both an abstract method makeSound()
and a concrete method eat()
.
What are Abstract Methods?
An abstract method is a method that is declared without implementation. It has no body and must be implemented by any subclass that inherits from the abstract class. An abstract method can have any visibility (public, private, protected), but it cannot have an implementation body (i.e., {}
).
In the previous example, makeSound()
is an abstract method because it does not have a body:
public abstract void makeSound();
Any subclass of Animal
must implement this method.
Benefits of Abstract Classes and Methods
Using abstract classes and methods provides several benefits:
Encapsulation: Abstract classes can encapsulate common behavior or attributes that are shared among related classes. Polymorphism: Abstract classes enable polymorphic behavior, where objects of different classes (subclasses) can be treated as if they were of the same class (the abstract class). Inheritance: Abstract classes allow for inheritance, which enables subclasses to inherit common behavior or attributes from a parent class. Code Reusability: By defining an abstract class and methods, you can reuse code across multiple subclasses.How to Define an Abstract Method?
To define an abstract method in Java, use the abstract
keyword before the method declaration:
public abstract void makeSound();
Note that abstract methods do not have a body (i.e., {}
).
How to Implement an Abstract Method?
In order for a subclass to implement an abstract method, it must provide its own implementation. The implementation can be provided either directly in the subclass or indirectly through inheritance from another class.
Here is an example of how a subclass might implement the makeSound()
method:
public class Dog extends Animal {
@Override
public void makeSound() {
System.out.println("Woof!");
}
}
In this example, Dog
is a subclass of Animal
, and it implements the abstract makeSound()
method by providing its own implementation.
Conclusion
Abstract classes and methods are powerful concepts in Java that enable polymorphism, encapsulation, inheritance, and code reusability. By understanding how to define and implement abstract methods, you can write more robust, maintainable, and scalable software systems.