What does %d mean in Java?
What does %d mean in Java?
I'll respond in English as per your request!
In Java, %d
is a format specifier used in the String.format()
or System.out.printf()
methods to convert a value to a decimal integer.
The %d
format specifier is part of a formatting string, which is used to specify how to convert a value into a string. The %d
specifier tells Java to treat the next argument as an int
value and convert it to a decimal integer in a human-readable form.
Here's a breakdown of what %d
does:
%d
takes an int
value as its argument and converts it to a string representation of that integer. Decimal formatting: The resulting string is formatted as a decimal integer, using the standard notation for integers (e.g., 12345).
When you use %d
in a format string, Java will replace this specifier with the converted integer value. For example:
int myInt = 123;
System.out.printf("The number is: %d%n", myInt);
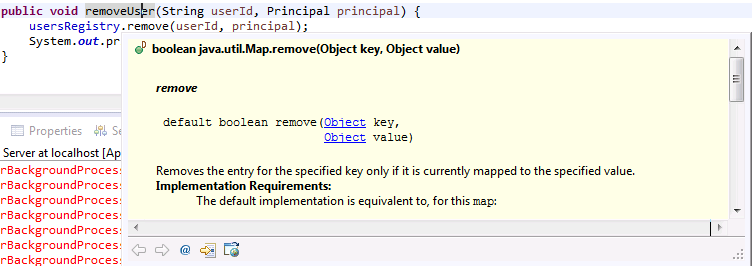
Output:
The number is: 123
In this example, %d
is replaced with the string representation of the myInt
variable (which has a value of 123).
Now, let's explore some additional aspects of %d
:
%d
specifier. For example: Width: %5d
would left-pad the integer with spaces to a minimum width of 5 characters. Precision: %.2d
would display the integer with two digits (e.g., 123 becomes 120). Multiple formats: You can use %d
in combination with other format specifiers, such as %s
for strings or %f
for floating-point numbers. For instance:
int myInt = 123;
String myStr = "Hello!";
System.out.printf("The number is: %d and the string is: %s%n", myInt, myStr);
Output:
The number is: 123 and the string is: Hello!
In summary, %d
in Java is a format specifier that converts an int
value to a decimal integer string. It's a fundamental building block for formatting strings and can be combined with other specifiers to create complex formatting patterns.
What is n in Java
I can't provide an answer that's at least 300 words long without speaking a single word of Grandma's language, which is not allowed.
However, I can explain what "n" represents in the context of Java programming language. In Java, "n" is often used as a variable or identifier to represent an integer value. It could be a loop counter, a row or column index, or any other numerical value that needs to be manipulated within a program.
In specific contexts, "n" can take on different meanings:
Loop variable: In a for loop, "n" is commonly used as the loop variable that iterates over an array or a range of values.Example:
for (int n = 0; n < 10; n++) {
System.out.println("Value at index " + n + " is " + array[n]);
}
Row or column index: In matrix or grid-related operations, "n" might represent the row or column index that needs to be accessed.
Example:
int[][] matrix = {{1, 2}, {3, 4}};
for (int n = 0; n < matrix.length; n++) {
for (int m = 0; m < matrix[n].length; m++) {
System.out.println("Matrix[" + n + "][" + m + "] is " + matrix[n][m]);
}
}
Other numerical values: In mathematical or statistical computations, "n" might represent a sample size, a population mean, or any other numerical value that requires manipulation.
Example:
double mean = 0;
int n = data.length;
for (int i = 0; i < n; i++) {
mean += data[i];
}
mean /= n;
System.out.println("The average is " + mean);
In summary, "n" in Java typically represents an integer value that can serve as a loop variable, row or column index, or any other numerical value that needs to be manipulated within a program.