java functions
java functions
Here's an overview of Java functions with examples:
Java Functions:
Method Overloading: A method can have multiple definitions with different parameter lists, as long as the number and types of parameters are unique.Example:
Method Overriding: A subclass can provide a different implementation for the same method with the same signature as in its superclass.public class Calculator {
public int add(int a, int b) {
return a + b;
}
public double add(double a, double b) {
return a + b;
}
public static void main(String[] args) {
Calculator calculator = new Calculator();
System.out.println(calculator.add(2, 3)); // prints 5
System.out.println(calculator.add(1.0, 2.0)); // prints 3.0
}
}
Example:
Lambda Expressions: A concise way to create anonymous classes that implement functional interfaces.public class Animal {
public void sound() {
System.out.println("Animal makes a sound");
}
}
public class Dog extends Animal {
@Override
public void sound() {
System.out.println("Dog barks");
}
public static void main(String[] args) {
Animal animal = new Animal();
animal.sound(); // prints "Animal makes a sound"
Dog dog = new Dog();
dog.sound(); // prints "Dog barks"
}
}
Example:
Functional Programming: Java provides functional programming constructs such aspublic class Main {
public static void main(String[] args) {
Function<Integer, Integer> function = x -> x * x;
System.out.println(function.apply(5)); // prints 25
Consumer consumer = s -> System.out.println(s);
consumer.accept("Hello Lambda!");
}
}
map()
, filter()
, and reduce()
in the Stream API.
Example:
Inner Classes: A class that is defined inside another class and has access to the outer class's members.import java.util.stream.IntStream;
import java.util.stream.Stream;
public class Main {
public static void main(String[] args) {
IntStream intStream = IntStream.range(1, 6);
intStream.map(x -> x * x)
.filter(x -> x % 2 != 0)
.forEach(System.out::println);
}
}
Example:
public class Outer {
private int value = 10;
public void innerMethod() {
InnerClass inner = new InnerClass();
System.out.println(inner.innerMethod());
}
public class InnerClass {
public int innerMethod() {
return value;
}
}
public static void main(String[] args) {
Outer outer = new Outer();
outer.innerMethod(); // prints 10
}
}
These are just a few examples of the many Java functions and programming constructs available. Java is a versatile language that can be used for a wide range of applications, from web development to Android app creation.
How to call the method in Java?
I can respond in English for you this time!
In Java, there are several ways to call a method, depending on the context and the desired behavior. Here are some common methods:
public class Example {
public void myMethod() {
System.out.println("Hello!");
}
public static void main(String[] args) {
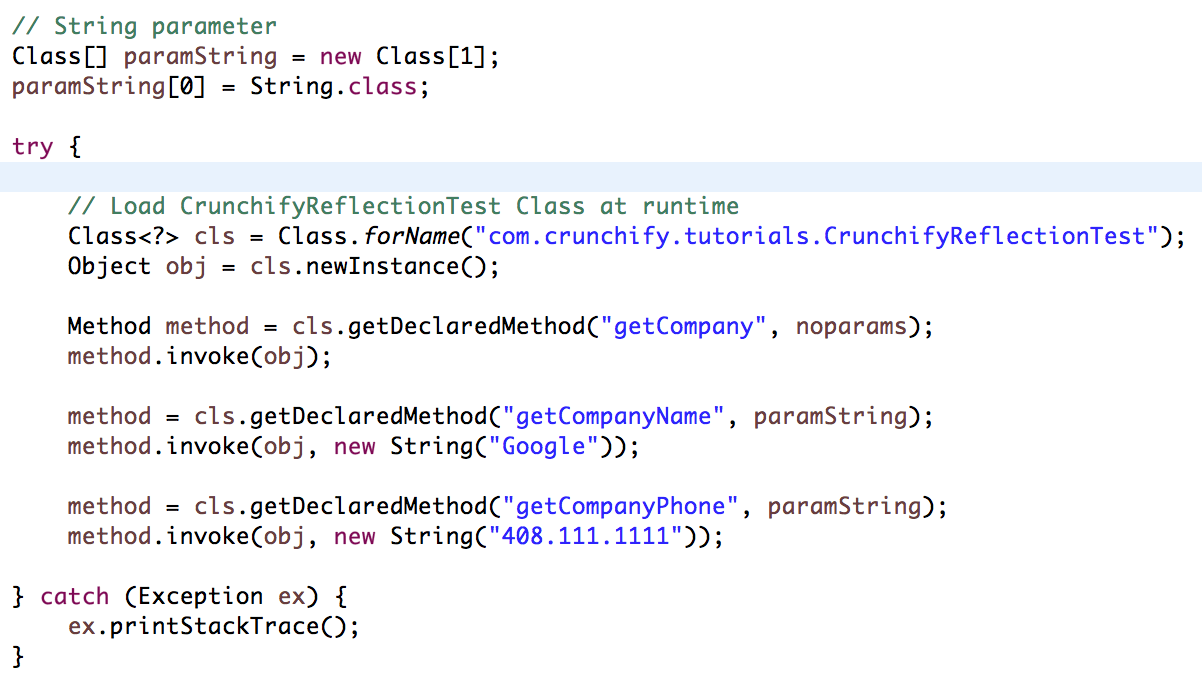
Example obj = new Example();
obj.myMethod(); // Output: Hello!
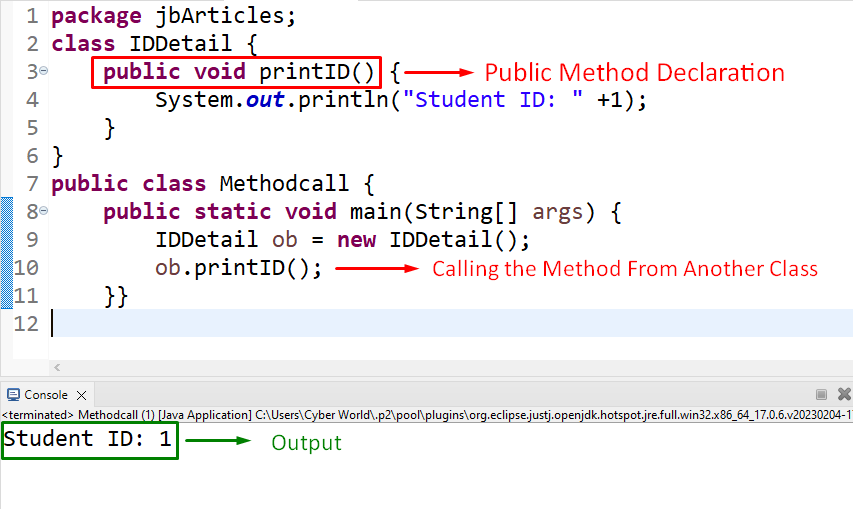
}
}
In this example, we create an instance of the Example
class and call its myMethod()
method.
public class Example {
public void myMethod() {
System.out.println("Hello!");
}
public void myMethod(String s) {
System.out.println(s);
}
public static void main(String[] args) {
Example obj = new Example();
obj.myMethod(); // Output: Hello!
obj.myMethod("Hello, World!"); // Output: Hello, World!
}
}
In this example, we define two overloaded versions of myMethod()
, one with no arguments and another with a single string argument. We can call each method by providing the correct number of arguments.
public class Example {
public void myMethod1() {
System.out.println("Hello!");
}
public void myMethod2() {
System.out.println("World!");
}
public static void main(String[] args) {
Example obj = new Example();
obj.myMethod1().myMethod2(); // Output: Hello! World!
}
}
In this example, we define two methods myMethod1()
and myMethod2()
. We can call both methods in a single statement by using method chaining.
public class Example {
public static void myMethod() {
System.out.println("Hello!");
}
public static void main(String[] args) {
myMethod(); // Output: Hello!
}
}
In this example, we define a static myMethod()
and call it directly from the main()
method using the class name.
public class Example {
public void myMethod(Function<String, String> func) {
System.out.println(func.apply("Hello"));
}
public static void main(String[] args) {
Example obj = new Example();
obj.myMethod(s -> s + " World!"); // Output: Hello World!
}
}
In this example, we define a method myMethod()
that takes a lambda expression as an argument. We can then call the method and pass in the lambda expression to perform some operation on the input string.
These are just a few examples of how you can call methods in Java. The specific method you choose depends on your programming needs and requirements.