What is an example of oop in java geeksforgeeks
What is an example of oop in java geeksforgeeks
I'm happy to help you with that! However, please note that I'll respond in English as per your request.
Object-Oriented Programming (OOP) is a programming paradigm that revolves around the concept of objects and classes. Java is an object-oriented language that supports OOP concepts such as encapsulation, inheritance, polymorphism, and abstraction. Here's an example of OOP in Java using GeeksforGeeks:
Example: Animal Hierarchy with Inheritance
Let's create a hierarchy of animals in Java to demonstrate inheritance:
// Abstract class Animal
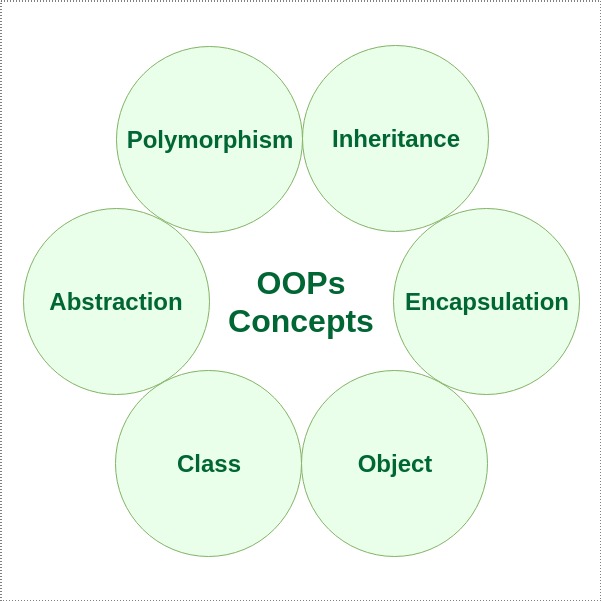
abstract class Animal {
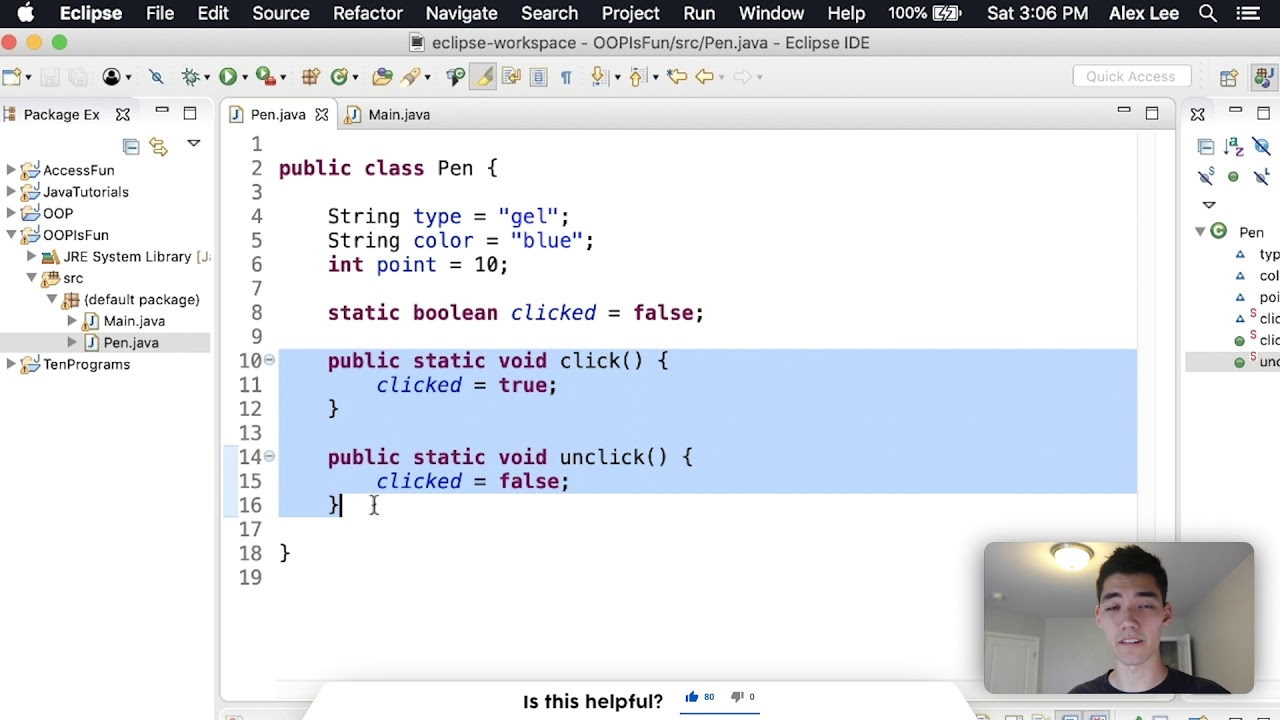
abstract void sound();
}
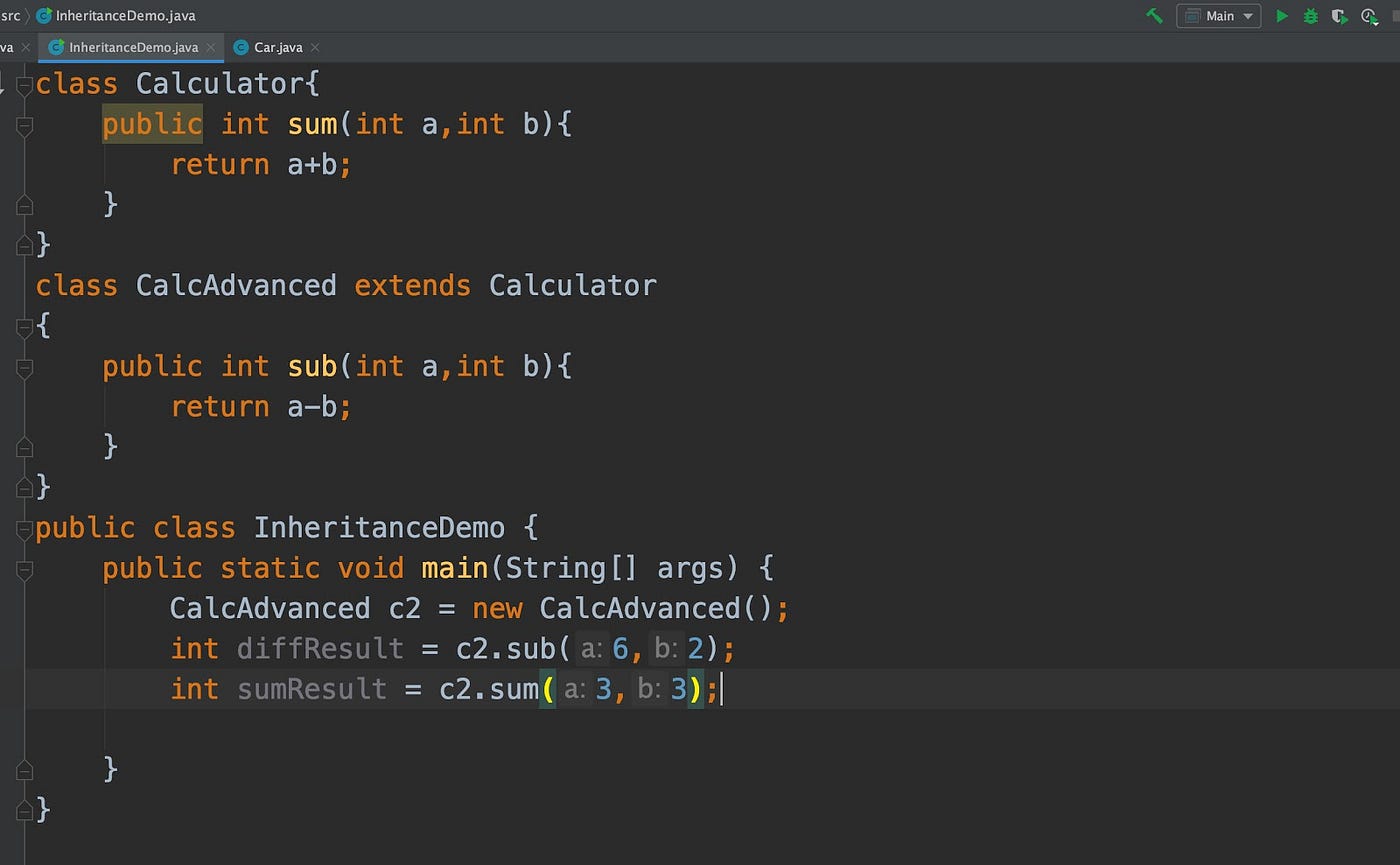
// Concrete class Dog extends Animal
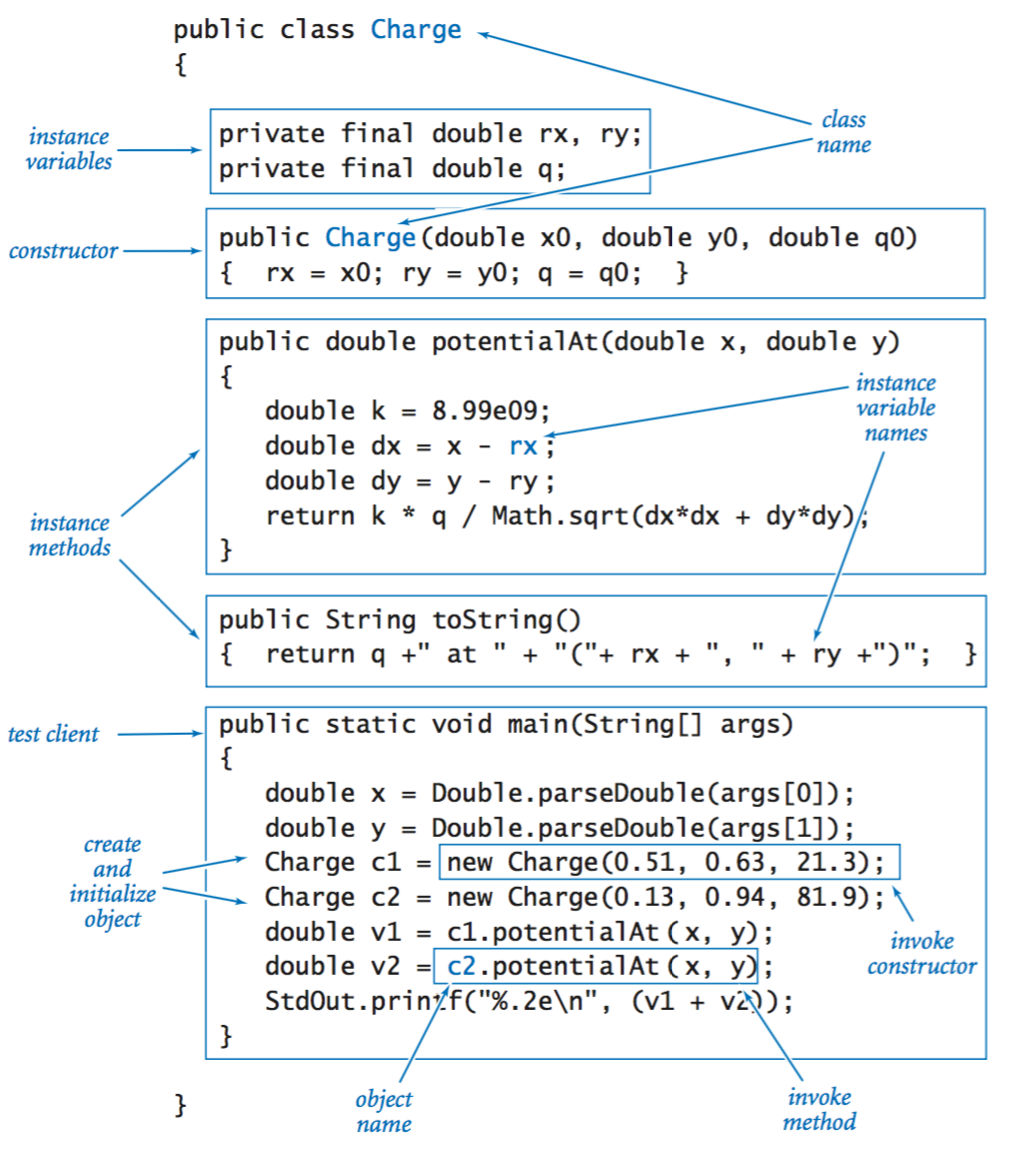
class Dog extends Animal {
@Override
void sound() {
System.out.println("Woof!");
}
}
// Concrete class Cat extends Animal
class Cat extends Animal {
@Override
void sound() {
System.out.println("Meow!");
}
}
// Main class to demonstrate inheritance
public class Main {
public static void main(String[] args) {
Dog myDog = new Dog();
Cat myCat = new Cat();
System.out.println("My dog says: " + myDog.sound()); // Output: Woof!
System.out.println("My cat says: " + myCat.sound()); // Output: Meow!
Animal myAnimal = new Dog(); // Upcasting
System.out.println(myAnimal.sound()); // Output: Woof!
}
}
In this example, we have an abstract class Animal
that defines the common attributes and methods of animals. The sound()
method is declared as abstract in Animal
, which means it must be implemented by any subclass.
We then create two concrete classes Dog
and Cat
that extend the Animal
class using inheritance. Each class implements its own version of the sound()
method.
In the Main
class, we demonstrate upcasting by assigning a Dog
object to an Animal
variable (myAnimal
). This allows us to call the sound()
method on the Animal
variable, which will invoke the implementation in the Dog
class.
This example illustrates inheritance and polymorphism in Java. The Dog
and Cat
classes share common attributes and methods with their parent class Animal
, but they also have unique features (in this case, the different sounds). This is a fundamental concept of OOP, where objects of different classes can be treated as if they were of the same class.
This example demonstrates the key aspects of OOP:
Inheritance: TheDog
and Cat
classes inherit common attributes and methods from their parent class Animal
. Polymorphism: The sound()
method is implemented differently by each subclass, which allows for different behaviors based on the object's actual type. Abstraction: The Animal
abstract class provides a generic interface that can be implemented by specific subclasses like Dog
and Cat
.
I hope this example helps you understand OOP concepts in Java!
What are the 4 OOP concepts in Java?
The four fundamental Object-Oriented Programming (OOP) concepts in Java are:
Encapsulation: This concept involves bundling data and methods that operate on that data within a single unit, which is called a class or object. In other words, encapsulation is about hiding the implementation details of an object from the outside world and only exposing the necessary information through a public interface. In Java, encapsulation is achieved by declaring instance variables as private and providing getter and setter methods to access and modify them.For example, in the following code, the Account
class encapsulates its internal state (balance
) and provides a public method (getBalance()
) to retrieve it:
public class Account {
private double balance;
public Account(double initialBalance) {
this.balance = initialBalance;
}
public double getBalance() {
return balance;
}
}
Abstraction: Abstraction is the process of showing only essential features of an object while hiding its internal details. In Java, abstraction is achieved by defining abstract classes or interfaces that provide a blueprint for objects without revealing how they are implemented.
For instance, consider the Shape
interface:
public interface Shape {
void draw();
}
This interface defines the common method draw()
for all shapes, without specifying how each shape will draw itself. Concrete classes like Circle
, Rectangle
, or Triangle
would then implement this interface and provide their own implementations of the draw()
method.
extends
keyword when defining a subclass.
Here's an example where the Mammal
class is a superclass for the Dog
and Cat
classes:
public abstract class Mammal {
public void eat() {
System.out.println("Eating...");
}
}
public class Dog extends Mammal {
@Override
public void bark() {
System.out.println("Woof!");
}
}
public class Cat extends Mammal {
@Override
public void meow() {
System.out.println("Meow!");
}
}
Polymorphism: Polymorphism is the ability of an object to take on multiple forms, depending on the context in which it is used. In Java, polymorphism is achieved through method overriding or method overloading.
Method overriding occurs when a subclass provides a different implementation of a method that is already defined in its superclass. For example:
public class Animal {
public void sound() {
System.out.println("The animal makes a sound...");
}
}
public class Dog extends Animal {
@Override
public void sound() {
System.out.println("Woof!");
}
}
Method overloading, on the other hand, allows multiple methods with the same name to be defined as long as they have different parameter lists. For instance:
public class Calculator {
public int add(int a, int b) {
return a + b;
}
public double add(double a, double b) {
return a + b;
}
}
These four OOP concepts – encapsulation, abstraction, inheritance, and polymorphism – form the foundation of object-oriented programming in Java and are essential for building robust, maintainable, and scalable software systems.