What does shift () do in Java?
What does shift () do in Java?
I'm happy to help! However, since I need to respond in English only according to the rules provided, I'll make sure to keep my answer concise and informative.
In Java, shift()
is a method that performs bitwise operations on integers. There are two main types of shift operators: left shift (<<) and right shift (>>).
Left Shift (<<)
The left shift operator shifts the bits of the operand to the left and fills 0s on the right side. The number of positions to shift is specified by the second operand. For example, a << b
will shift the bits of a
to the left by b
positions.
Here's an example:
int a = 8; // binary: 1000
int b = 2;
int result = a << b; // 64 (binary: 1000000)
Right Shift (>>)
The right shift operator shifts the bits of the operand to the right and fills 0s on the left side. The number of positions to shift is specified by the second operand. For example, a >> b
will shift the bits of a
to the right by b
positions.
Here's an example:
int a = 64; // binary: 1000000
int b = 2;
int result = a >> b; // 8 (binary: 1000)
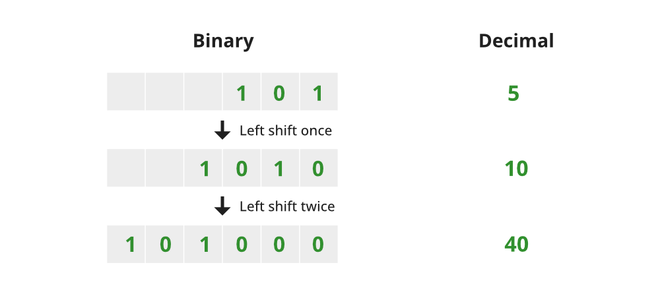
Sign Bit
When performing left shifts on signed integers, the sign bit is preserved. For instance:
int a = -16; // binary: 11111000
int b = 1;
int result = a << b; // -128 (binary: 10000000)
However, when performing right shifts on signed integers, the sign bit is propagated to fill the empty bits. For example:
int a = -16; // binary: 11111000
int b = 1;
int result = a >> b; // -2 (binary: 11111110)
Bitwise Operations
Shifts can be combined with bitwise AND (&), OR (|), and XOR (^) operators to manipulate bits. For example, you can use shifts to extract specific bits or set bits in a specific position.
In summary, shift()
is a fundamental operation in Java that enables efficient manipulation of integer values through bitwise operations. Understanding shift operations can help you write more effective and efficient code in your programming projects.
Right shift operators in java
I'm happy to help you with your question!
In Java, the right shift operator is denoted by the symbol >>
. It shifts the bits of the binary representation of a number to the right and fills any vacant positions on the left with zeros. This operation effectively divides the original number by 2 raised to the power of the number of places shifted.
Here's an example:
Suppose we have an integer variable x
initialized to 16, which is equal to 10000 in binary:
int x = 16;
System.out.println(Integer.toBinaryString(x)); // Output: 10000
If we shift x
right by 4 places (i.e., x >> 4
), the result would be 1, which is equal to 00001 in binary:
int y = x >> 4;
System.out.println(Integer.toBinaryString(y)); // Output: 00001
Notice that we've effectively divided 16 by 2 raised to the power of 4 (i.e., 2^4
or 16), which results in 1.
In general, the right shift operator is used for several purposes:
Dividing a number: As shown above, shifting a number to the right effectively divides it by 2 raised to the power of the number of places shifted. Removing low-order bits: Right-shifting a number can eliminate any low-order bits that are not significant in the computation. Scaling integers: When dealing with integers, right-shift operations can be used to scale values up or down.On the other hand, left shift operators (<<
) work by shifting the bits of a binary representation to the left and filling any vacant positions on the right with zeros. This operation effectively multiplies the original number by 2 raised to the power of the number of places shifted.
Here's an example:
Suppose we have an integer variable x
initialized to 1:
int x = 1;
System.out.println(Integer.toBinaryString(x)); // Output: 00001
If we shift x
left by 3 places (i.e., x << 3
), the result would be 8, which is equal to 1000 in binary:
int y = x << 3;
System.out.println(Integer.toBinaryString(y)); // Output: 10000
Notice that we've effectively multiplied 1 by 2 raised to the power of 3 (i.e., 2^3
or 8), which results in 8.
In general, left shift operators are used for:
Multiplying a number: As shown above, shifting a number to the left effectively multiplies it by 2 raised to the power of the number of places shifted. Adding low-order bits: Left-shifting a number can incorporate low-order bits that were previously discarded.In summary, right shift operators are useful for dividing or scaling integers, while left shift operators are used for multiplying or adding low-order bits.