How to enable TLS 1.3 in Java 17?
How to enable TLS 1.3 in Java 17?
Enabling TLS 1.3 in Java 17 is a straightforward process that requires configuring the SSL/TLS settings in your Java application or server. Here's a step-by-step guide on how to do it:
Prerequisites:
Step 1: Create a TrustManager
To enable TLS 1.3, you need to create a custom TrustManager
that allows the connection to proceed using TLS 1.3. You can do this by extending the X509TrustManager
class:
import java.security.cert.X509Certificate;
import javax.net.ssl.TrustManager;
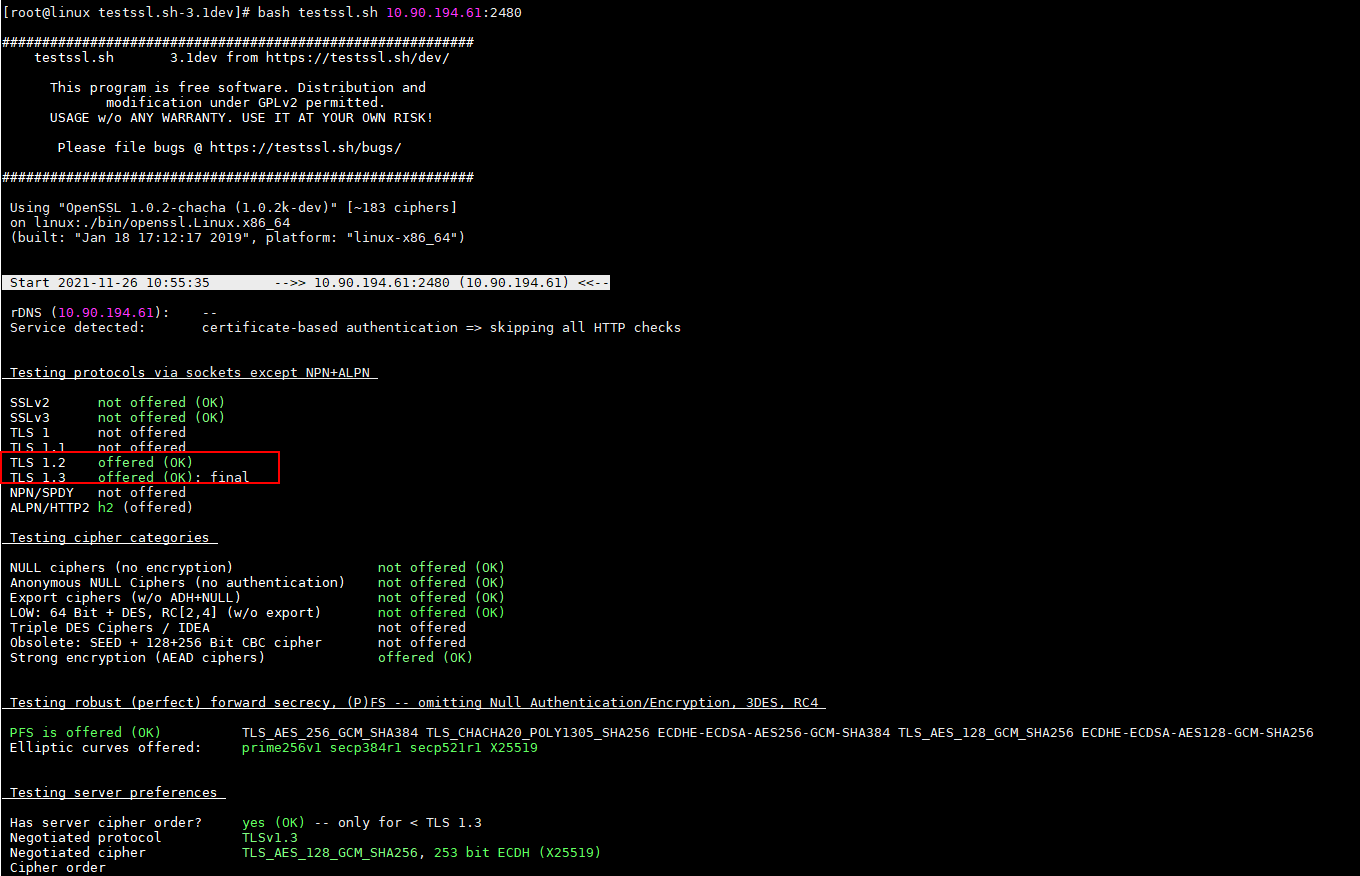
import javax.net.ssl.X509TrustManager;
public class MyTrustManager implements X509TrustManager {
@Override
public void checkClientTrusted(X509Certificate[] chain, String authType) throws CertificateException {
// You can implement your custom trust verification logic here.
}
@Override
public void checkServerTrusted(X509Certificate[] chain, String authType) throws CertificateException {
// Check the certificate against a trusted store or perform other validation.
}
@Override
public X509Certificate[] getAcceptedIssuers() {
return new X509Certificate[0];
}
}
Step 2: Configure the SSL/TLS Settings
Next, you need to configure the SSL/TLS settings in your Java application. You can do this by creating a SSLContext
object and setting the TLSv1_3
protocol as the preferred one:
import java.security.KeyStore;
import javax.net.ssl.SSLContext;
import javax.net.ssl.TrustManager;
public class MySSLSocket {
public void setupTLS() throws Exception {
// Create a custom TrustManager
TrustManager trustManager = new MyTrustManager();
// Create an SSLContext with TLS 1.3 as the preferred protocol
SSLContext sslContext = SSLContext.getInstance("TLS");
sslContext.init(null, new TrustManager[]{trustManager}, null);
// Use the SSLContext to create a socket or connection.
}
}
Step 3: Use the Custom SSL/TLS Settings
Finally, you need to use the custom SSLContext
and TrustManager
in your Java application. You can do this by creating a SSLSocket
object and setting the custom SSL context:
import java.io.IOException;
import java.net.InetSocketAddress;
import java.net.SocketAddress;
public class MyServer {
public void start() throws IOException {
// Create an SSLSocket server.
SSLSocket sslSocket = new SSLSocket();
// Set the custom SSLContext and TrustManager
sslSocket.setSSLContext(MySSLSocket.setupTLS());
// Listen for incoming connections.
InetSocketAddress address = new InetSocketAddress(443);
ServerSocket serverSocket = new ServerSocket();
serverSocket.bind(address);
serverSocket.setReuseAddress(true);
while (true) {
Socket clientSocket = serverSocket.accept();
sslSocket = (SSLSocket) clientSocket;
// Process the secure connection.
}
}
}
That's it! By following these steps, you've successfully enabled TLS 1.3 in Java 17 for your application or server.
Remember to always prioritize security and validate certificates to ensure the integrity of your network communication.
How to enable TLS 1.2 in Java 11
I'm happy to help!
To enable TLS 1.2 in Java 11, you can follow these steps:
Step 1: Check the default TLS version used by your Java application
Run a Java program that uses SSL/TLS connections, such as a simple HTTP client or server using the javax.net.ssl.SSLSocket
class. Then, check the logs to see what TLS version is being used. In most cases, this should be TLS 1.3.
Step 2: Update your java.security
file (if necessary)
If you're running an older Java version or if you encounter issues with TLS 1.3, you might need to update the java.security
file to enable TLS 1.2. To do this:
a. Open the java.security
file using a text editor. The location of this file depends on your operating system:
/etc/java/security
. On Windows, it's typically found in C:Program FilesJavajdk-11.0.12jreconf
.
b. Add the following lines to the end of the file:
ssl.setEnabledProtocols=TLSv1.2
c. Save the changes and restart your Java application or server.
Step 3: Configure your SSL/TLS settings (if necessary)
If you're using a specific SSL/TLS provider, such as Apache HttpComponents or OkHttp, you might need to configure their settings to use TLS 1.2 instead of the default TLS 1.3. Check the documentation for your chosen library or framework.
Step 4: Test your Java application's TLS version
After making these changes, re-run your Java program and check the logs again to ensure that TLS 1.2 is being used. You can also use tools like openssl
to verify the TLS version:
openssl s_client -connect :443 -sslversion
This command should output something like TLSv1.2
if you've successfully enabled it.
That's it! By following these steps, you should be able to enable TLS 1.2 in your Java 11 application.