How to set proxy in Java code
How to set proxy in Java code
Here is the information about how to set a proxy in your Java program:
Why use a proxy?
Using a proxy server can help you bypass restrictions, hide your IP address, or speed up certain network operations. For example, if you're behind a corporate firewall that blocks direct access to certain websites, setting a proxy can allow you to access those websites indirectly through the proxy server.
How to set a proxy in Java
You can set a proxy in your Java code using the Proxy
class provided by the Java API. Here are some steps to follow:
Proxy
object: Use the Proxy
constructor to create a new Proxy
object, specifying the hostname and port number of the proxy server.
import java.net.Proxy;
import java.net.URL;
Proxy proxy = new Proxy(new InetSocketAddress("proxy.example.com", 8080), true);
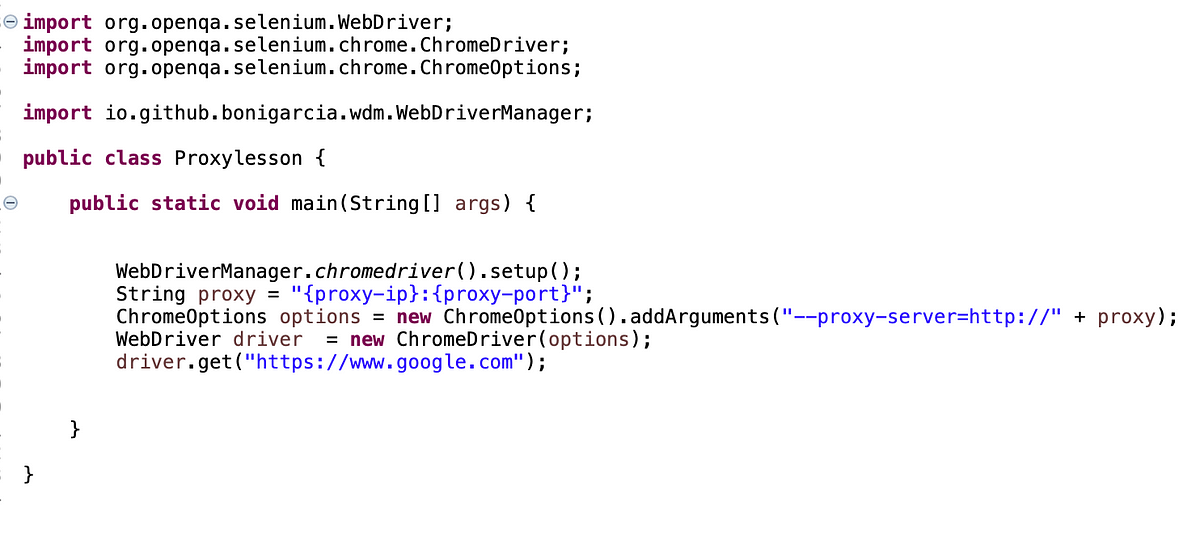
In this example, we're creating a Proxy
object that connects to the proxy server at proxy.example.com
on port 8080
.
URL
object: Use the URL
class to create a new URL
object pointing to the resource you want to access (e.g., a website).
URL url = new URL("https://www.example.com");
Use the Proxy
object with the URL
object: Use the Proxy
object with the URL
object to establish a connection through the proxy server.
URLConnection conn = (HttpURLConnection) url.openConnection(proxy);
conn.setRequestMethod("GET");
int responseCode = conn.getResponseCode();
In this example, we're opening an HTTP connection using the openConnection()
method and specifying the Proxy
object as the proxy to use. We then set the request method to "GET" and get the response code from the server.
System.setProperty()
method to set system properties related to proxies, such as http.proxyHost
and http.proxyPort
. For example:
System.setProperty("http.proxyHost", "proxy.example.com");
System.setProperty("http.proxyPort", "8080");
This sets the HTTP proxy host and port for your Java program.
Use a library or framework: If you're using a Java library or framework (e.g., Spring, Apache HttpClient), it may provide its own mechanisms for setting proxies. Consult the documentation for the specific library or framework you're using to learn more about how to set a proxy.Additional considerations
When setting up a proxy in your Java program, keep the following points in mind:
Make sure the proxy server is configured correctly and allows connections from your Java program. Be aware of any restrictions or limitations imposed by the proxy server, such as rate limits or blocked ports. Consider using a reliable and fast proxy server to minimize latency and improve performance.I hope this helps! Let me know if you have further questions.
Java HTTP proxy server
A Java-based HTTP proxy server!
An HTTP proxy server is a type of application that acts as an intermediary between clients and servers, forwarding requests from the client to the target server and returning the response back to the client. A Java-based HTTP proxy server can be built using various libraries and frameworks.
Here's an overview of how such a system can be implemented in Java:
Key Components
Java Servlet Container: The foundation of any Java-based web application is a servlet container, which handles incoming requests and dispatches them to the corresponding servlet or filter. Popular choices include Apache Tomcat, Jetty, and Eclipse Equinox. Java HTTP Proxy Servlet: This is the core component that handles the actual proxying functionality. It extends theHttpServlet
class in Java and overrides the doGet()
and/or doPost()
methods to process incoming requests. HTTP Client Library: A library like Apache HttpClient or OkHttp can be used to send requests to the target server and retrieve responses.
Implementing a Simple HTTP Proxy Server
Here's a basic outline of how you could implement a simple HTTP proxy server in Java:
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
public class HttpProxyServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse res) throws ServletException, IOException {
// Get the URL from the request
String url = req.getRequestURI();
// Create a new HTTP connection to the target server
HttpURLConnection conn = (HttpURLConnection) new URL(url).openConnection();
conn.setRequestMethod(req.getMethod());
// Set headers and body as needed
// Send the request
int responseCode = conn.getResponseCode();
// Get the response
BufferedReader reader = new BufferedReader(new InputStreamReader(conn.getInputStream()));
String line;
StringBuilder response = new StringBuilder();
while ((line = reader.readLine()) != null) {
response.append(line);
}
reader.close();
// Send the response back to the client
res.setContentLength(response.length());
res.getOutputStream().write(response.toString().getBytes());
}
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse res) throws ServletException, IOException {
doGet(req, res);
}
}
Adding Features and Improvements
Of course, this is a very basic implementation, and you'll likely want to add features like:
Authentication and authorization mechanisms Caching for frequently accessed resources Support for HTTPS (SSL/TLS) connections Handling of HTTP/2 and other modern protocols Error handling and loggingThese are just some of the considerations when building a Java-based HTTP proxy server. Depending on your specific requirements, you may also need to add additional functionality or tweak performance optimization techniques.
Conclusion
Building an HTTP proxy server in Java can be a fun and rewarding project! With the right libraries and frameworks, it's possible to create a robust and highly customizable solution for managing web traffic.