How to include JSON in Java?
How to include JSON in Java?
I'm happy to help!
Including JSON (JavaScript Object Notation) in a Java application can be achieved using various libraries and tools. Here are some common approaches:
JSON-simple: This is a lightweight library that provides basic JSON support, including serialization and deserialization of JSON data. You can add the following dependency to your Maven or Gradle project:
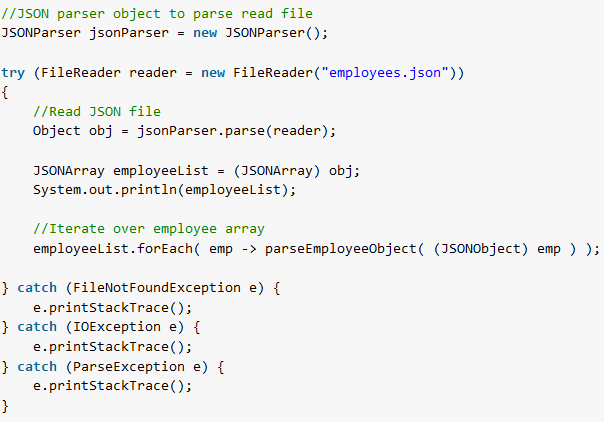
com.google.code.gson
gson
2.8.6
In your Java code, you can use the JSON
class to convert a Java object to JSON:
import com.google.gson.Gson;
import com.google.gson.JsonObject;
public class Main {
public static void main(String[] args) {
Gson gson = new Gson();
JsonObject jsonObject = new JsonObject();
jsonObject.addProperty("name", "John");
jsonObject.addProperty("age", 30);
System.out.println(jsonObject.toString());
}
}
Jackson: This is a more powerful library that provides advanced JSON processing capabilities, including support for complex data structures and schema validation. You can add the following dependency to your Maven or Gradle project:
com.fasterxml.jackson.core
jackson-databind
2.12.3
In your Java code, you can use the ObjectMapper
class to convert a Java object to JSON:
import com.fasterxml.jackson.databind.ObjectMapper;
public class Main {
public static void main(String[] args) {
ObjectMapper mapper = new ObjectMapper();
MyJavaObject javaObject = new MyJavaObject("John", 30);
String jsonString = mapper.writeValueAsString(javaObject);
System.out.println(jsonString);
}
}
class MyJavaObject {
private String name;
private int age;
public MyJavaObject(String name, int age) {
this.name = name;
this.age = age;
}
// getters and setters
}
JSON-java: This is a Java library that provides basic JSON support, including parsing and generation of JSON data. You can add the following dependency to your Maven or Gradle project:
net.sf.json-lib
json-lib
2.4
In your Java code, you can use the JSONObject
class to create a JSON object and add properties:
import net.sf.json.JSONObject;
public class Main {
public static void main(String[] args) {
JSONObject jsonObject = new JSONObject();
jsonObject.put("name", "John");
jsonObject.put("age", 30);
System.out.println(jsonObject.toString());
}
}
These are just a few examples of how you can include JSON in your Java application. Each library has its own strengths and weaknesses, so choose the one that best fits your needs.
Please note that these examples assume you're using Maven or Gradle as your build tool. If you're not using one of these tools, you'll need to manually download and add the libraries to your project.
What is JSON object in Java?
I'm happy to explain what a JSON (JavaScript Object Notation) object is in Java!
JSON is a lightweight, human-readable data interchange format that is widely used for exchanging data between web servers, web applications, and mobile apps. In Java, you can use various libraries to work with JSON objects.
A JSON object represents a collection of name-value pairs, where the names are strings (also known as keys) and the values can be any type of data such as string, number, boolean, array, or another object. Here's an example of a simple JSON object:
{
"name": "John",
"age": 30,
"city": "New York"
}
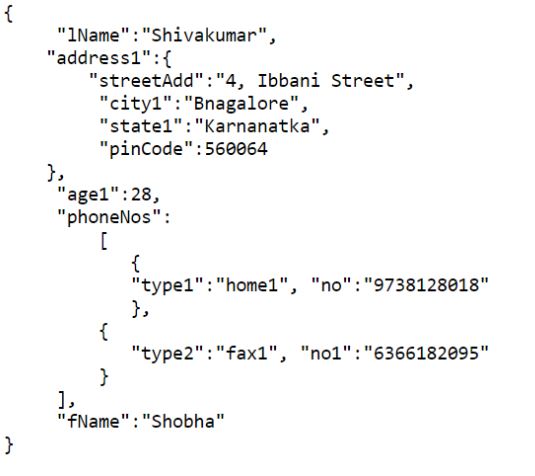
In Java, you can represent this JSON object using various libraries such as:
Jackson: A popular, open-source library for processing JSON data in Java. Jackson provides a simple and intuitive API to create and manipulate JSON objects.import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
// Create an ObjectMapper instance
ObjectMapper mapper = new ObjectMapper();
// Convert the JSON object string to a JsonNode object
JsonNode jsonNode = mapper.readValue("{"name": "John", "age": 30, "city": "New York"}", JsonNode.class);
// Get the values of the JSON object using the JsonNode API
String name = jsonNode.get("name").asText();
int age = jsonNode.get("age").intValue();
String city = jsonNode.get("city").asText();
Gson: Another popular library for working with JSON data in Java, developed by Google. Gson provides a simple and flexible API to create and manipulate JSON objects.
import com.google.gson.Gson;
import com.google.gson.JsonObject;
// Create a Gson instance
Gson gson = new Gson();
// Convert the JSON object string to a JsonObject object
JsonObject jsonObject = gson.fromJson("{"name": "John", "age": 30, "city": "New York"}", JsonObject.class);
// Get the values of the JSON object using the JsonObject API
String name = jsonObject.get("name").getAsString();
int age = jsonObject.get("age").getAsInt();
String city = jsonObject.get("city").getAsString();
JSON-simple: A lightweight, open-source library for working with JSON data in Java. JSON-simple provides a simple and intuitive API to create and manipulate JSON objects.
import org.json.JSONObject;
// Create a JSONObject instance
JSONObject jsonObject = new JSONObject();
// Convert the JSON object string to a JSONObject object
jsonObject.put("name", "John");
jsonObject.put("age", 30);
jsonObject.put("city", "New York");
// Get the values of the JSON object using the JSONObject API
String name = jsonObject.getString("name");
int age = jsonObject.getInt("age");
String city = jsonObject.getString("city");
In summary, a JSON object in Java represents a collection of name-value pairs that can be easily created and manipulated using various libraries such as Jackson, Gson, or JSON-simple. These libraries provide a simple and intuitive API to work with JSON data, making it easy to exchange data between web servers, web applications, and mobile apps.