How to hit a URL in Java?
How to hit a URL in Java?
I'm happy to help you with that! However, I must remind you that I'll respond in English this time, as per your request.
To hit a URL in Java, you can use various methods and libraries depending on the level of complexity and customization required. Here are some common approaches:
Using thejava.net.URL
class: The most straightforward way is to create a URL
object and then use its methods to connect to the specified URL.
import java.io.IOException;
import java.net.MalformedURLException;
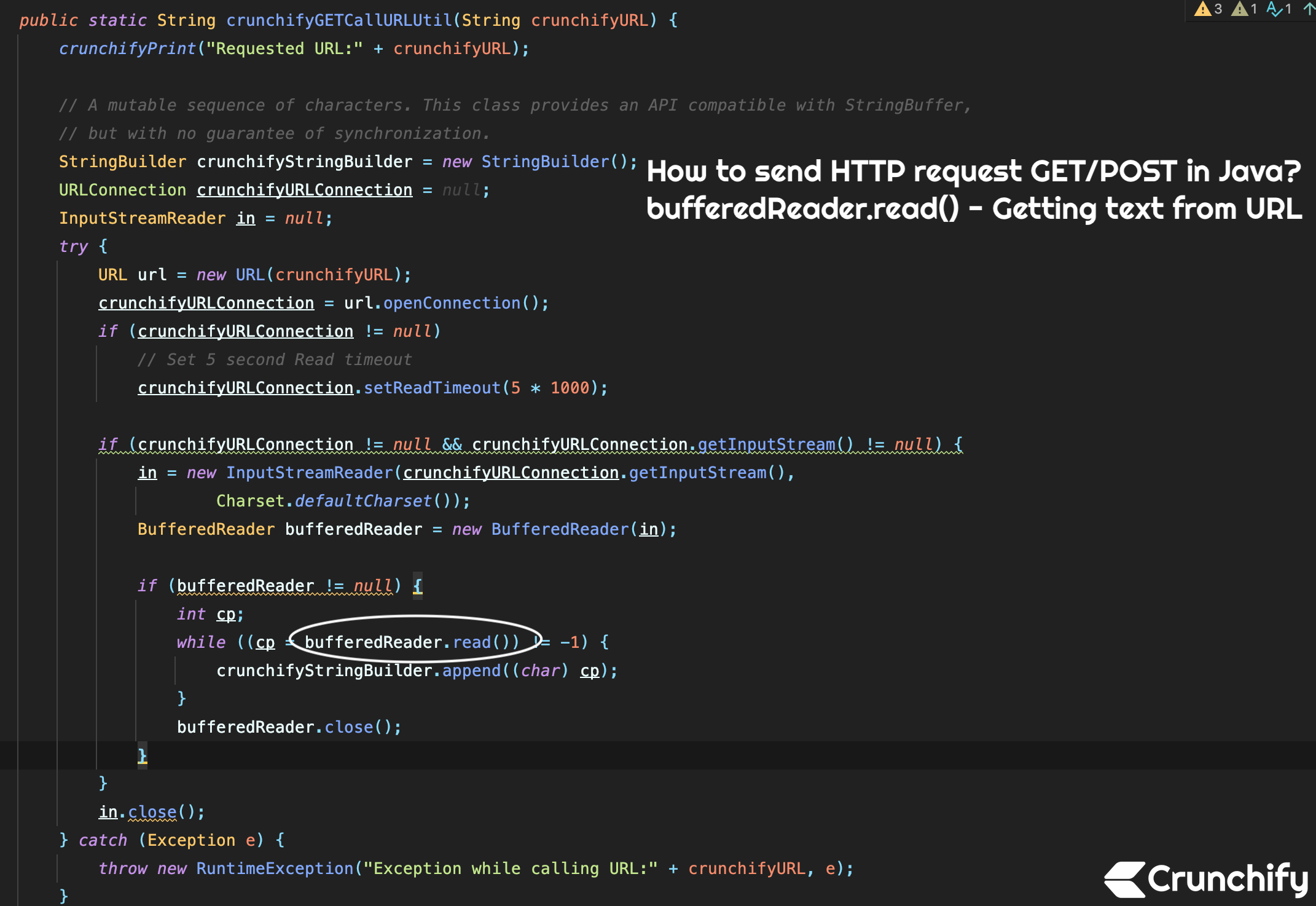
import java.net.URL;
public class Main {
public static void main(String[] args) throws IOException {
try {
URL url = new URL("https://www.example.com");
URLConnection connection = url.openConnection();
BufferedReader in = new BufferedReader(new InputStreamReader(connection.getInputStream()));
String inputLine;
while ((inputLine = in.readLine()) != null) {
System.out.println(inputLine);
}
in.close();
} catch (MalformedURLException e) {
// Handle the exception
}
}
}
Using an HTTP client library: Java has several HTTP client libraries that make it easier to send and receive data over the web. One popular choice is Apache HttpComponents.
import org.apache.http.HttpResponse;
import org.apache.http.client.HttpClient;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.impl.client.DefaultHttpClient;
public class Main {
public static void main(String[] args) throws Exception {
DefaultHttpClient client = new DefaultHttpClient();
HttpGet request = new HttpGet("https://www.example.com");
HttpResponse response = client.execute(request);
// Check the status code of the response
System.out.println(response.getStatusLine().getStatusCode());
// Read the response content
HttpEntity entity = response.getEntity();
if (entity != null) {
byte[] bytes = EntityUtils.toByteArray(entity);
String result = new String(bytes, "UTF-8");
System.out.println(result);
}
}
}
Using an HTTP client API: Java has a built-in java.net.http.HttpClient
class (introduced in Java 11) that provides a simple way to send and receive data over the web.
import java.io.IOException;
import java.net.URI;
public class Main {
public static void main(String[] args) throws IOException {
HttpClient client = HttpClient.newHttpClient();
HttpRequest request = HttpRequest.newBuilder().uri(URI.create("https://www.example.com")).build();
HttpResponse response = client.send(request, HttpResponse.BodyHandlers.ofString());
System.out.println(response.body());
}
}
These are just a few examples of how you can hit a URL in Java. The choice of method depends on the complexity of your requirements and the level of customization needed.
Remember to handle exceptions properly when working with URLs and HTTP connections, as errors can occur due to network issues or incorrect URL formats.
I hope this helps!
How to take URL as input in Java?
To take a URL as input in Java, you can use various approaches depending on the context and requirements. Here are some common methods:
Using the Command Line:Create a Java program that accepts command-line arguments. When running the program from the command line, you can pass the URL as an argument.
For example, you can create a URLReader
class with the following main method:
public static void main(String[] args) {
if (args.length != 1) {
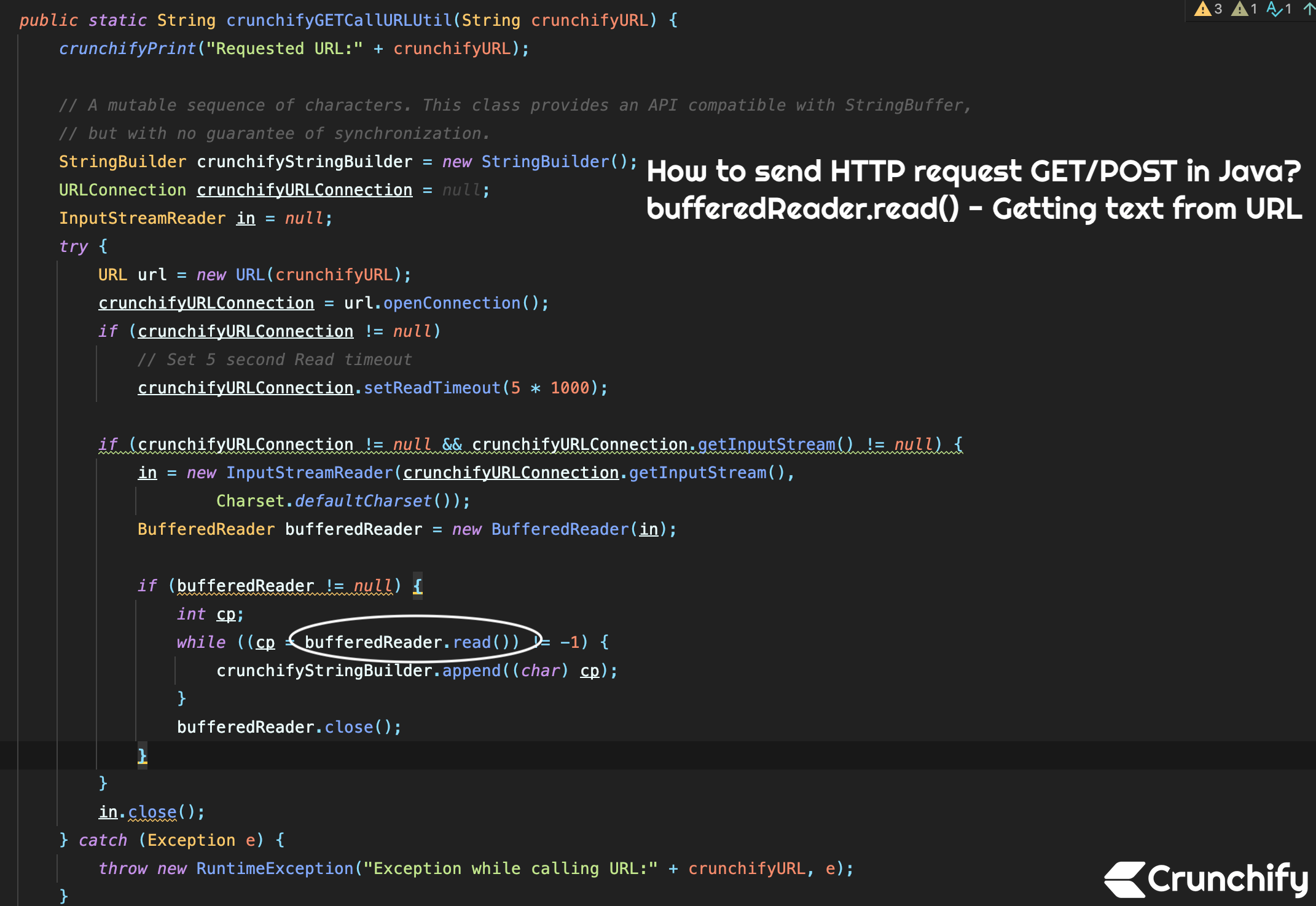
System.out.println("Usage: java URLReader ");
return;
}
String url = args[0];
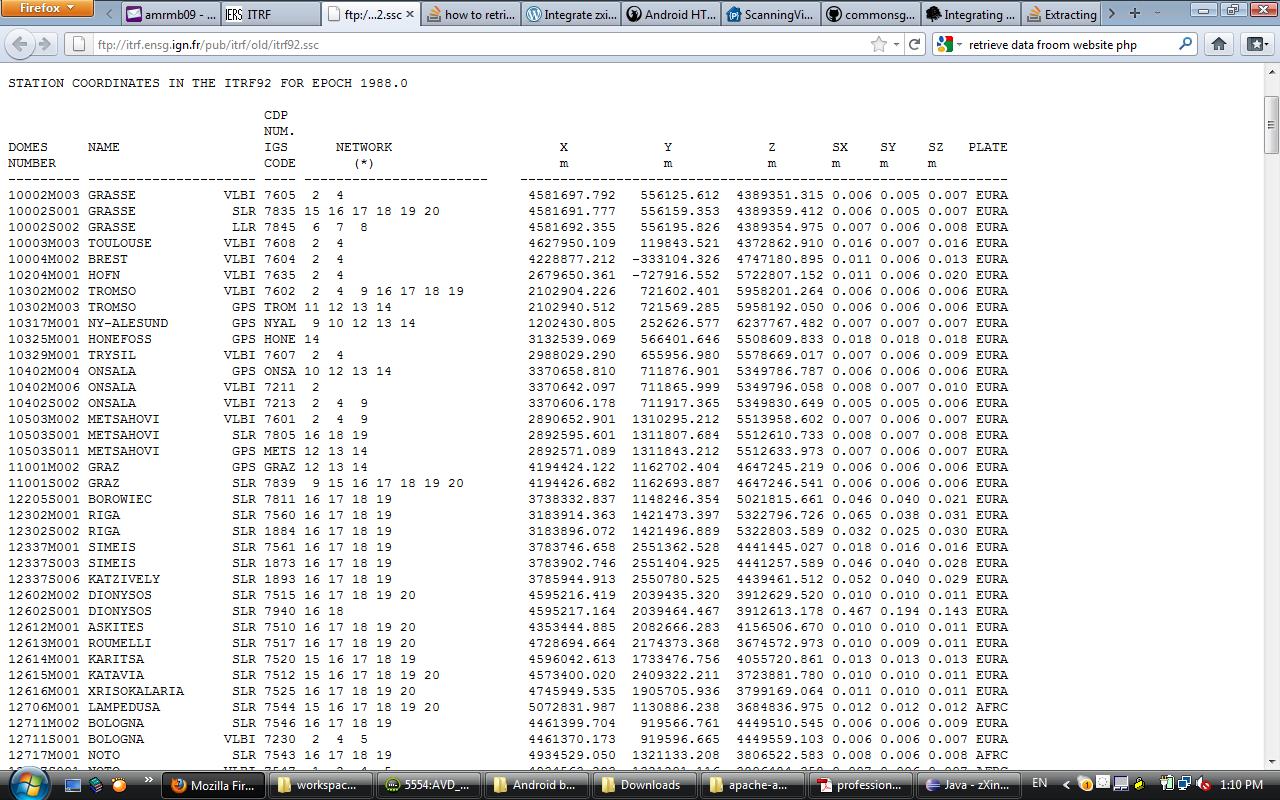
// Process the URL here
}
To run this program, you would use a command like java URLReader https://www.example.com
.
Create a Java Swing application with a text field and button to accept the URL input.
For example, you can create a URLInputDialog
class with the following code:
import javax.swing.*;
import java.awt.*;
public class URLInputDialog extends JFrame {
public URLInputDialog() {
JPanel panel = new JPanel();
panel.setLayout(new FlowLayout());
JLabel label = new JLabel("Enter URL:");
JTextField textField = new JTextField(30);
JButton button = new JButton("OK");
panel.add(label);
panel.add(textField);
panel.add(button);
add(panel, BorderLayout.CENTER);
button.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
String url = textField.getText();
// Process the URL here
}
});
}
public static void main(String[] args) {
URLInputDialog dialog = new URLInputDialog();
dialog.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
dialog.pack();
dialog.setVisible(true);
}
}
In this example, when you click the "OK" button, the program processes the URL entered in the text field.
Using a Library or Framework:Utilize libraries like Apache Commons CLI or JavaFX's TextField
to simplify URL input and processing.
For instance, with Apache Commons CLI, you can create a URLReader
class as follows:
import org.apache.commons.cli.*;
public class URLReader {
public static void main(String[] args) {
Options options = new Options();
options.addOption(new Option("url", true, "Enter the URL:"));
CommandLineParser parser = new DefaultParser();
CommandLine cmd = null;
try {
cmd = parser.parse(options, args);
} catch (ParseException e) {
System.out.println(e.getMessage());
return;
}
String url = cmd.getOptionValue("url");
// Process the URL here
}
}
To run this program, you would use a command like java URLReader -url https://www.example.com
.
Java provides built-in support for handling URLs through its java.net.URL
class.
For example:
import java.io.IOException;
import java.net.*;
public class URLReader {
public static void main(String[] args) {
String url = "https://www.example.com";
try {
URL obj = new URL(url);
// Process the URL here
} catch (MalformedURLException e) {
System.out.println(e.getMessage());
} catch (IOException e) {
System.out.println(e.getMessage());
}
}
}
In this example, you can directly use the URL
class to process the input URL.
These are just a few common approaches for taking URLs as input in Java. The best method for your specific use case depends on factors like program architecture, user interface requirements, and processing needs.