CompletableFuture Java 21
CompletableFuture Java 21
CompletableFuture in Java 21
In Java 8 and later versions, the CompletableFuture
class was introduced as part of the Java API to support asynchronous programming using lambda expressions and method references. It provides a powerful way to handle asynchronous computations by allowing you to compose together multiple tasks that can run concurrently or sequentially.
Key Features
Asynchronous Execution:CompletableFuture
allows you to execute tasks asynchronously, which means that your code can continue running without blocking until the task is completed. Handling Completion: You can handle the completion of a task by providing an onComplete()
method that gets called when the task is finished.
CompletableFuture
instances can be composed together to create complex workflows involving multiple tasks, which can run concurrently or sequentially. Error Handling: You can handle errors that occur during task execution using the whenComplete()
and exceptionally()
methods.
Example Usage
Here's a simple example of how you might use CompletableFuture
in a Java application:
import java.util.concurrent.*;
public class CompletableFutureExample {
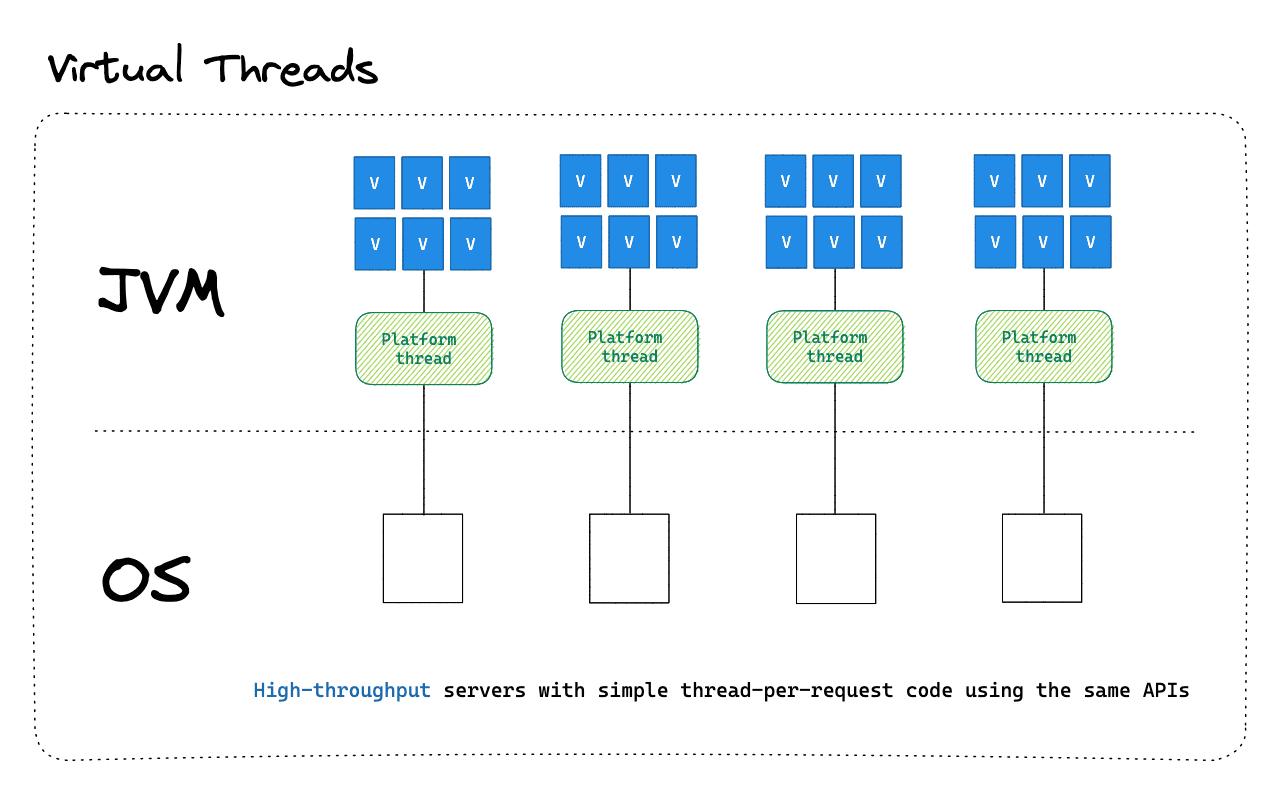
public static void main(String[] args) throws InterruptedException, ExecutionException {
// Create a CompletableFuture that runs after 2 seconds
CompletableFuture future = CompletableFuture.runAfterDelay(() -> {
try {
Thread.sleep(2000);
} catch (InterruptedException e) {
throw new RuntimeException(e);
}
return 42;
});
// Get the result of the future when it's complete
FutureResult result = future.whenComplete((r, ex) -> {
if (ex == null) {
System.out.println("The answer is: " + r.get());
} else {
System.out.println("An error occurred: " + ex.getMessage());
}
});
// Run the main thread for a few seconds to simulate some other work
Thread.sleep(3000);
}
}
Best Practices
When working with CompletableFuture
, it's essential to follow best practices to avoid common pitfalls:
.get()
: The get()
method can block your program if the task has not yet completed, leading to performance issues. Use whenComplete()
instead of .thenApply()
: The whenComplete()
method allows you to handle the completion of a task without blocking, whereas thenApply()
will block until the task is finished. Test for Errors: Always test for errors and handle them appropriately using the exceptionally()
or whenComplete()
methods.
Conclusion
CompletableFuture
provides a powerful way to handle asynchronous computations in Java, allowing you to create complex workflows that can run concurrently or sequentially. By following best practices and understanding its key features and usage, you can effectively use CompletableFuture
to improve the performance of your applications.
What is a CompletableFuture in Java?
I'm glad you asked!
In Java, a CompletableFuture
is a part of the Java Future API that allows you to write asynchronous code that's easier to read and maintain. Introduced in Java 8, it's designed to simplify working with asynchronous tasks and promises.
A CompletableFuture
represents an asynchronous computation whose result is either completed or will be completed when the computation finishes. This class provides a flexible way to compose and manipulate asynchronous computations.
Here are some key aspects of CompletableFuture
:
CompletableFuture
allows you to execute code asynchronously, which means your program can continue running without blocking on an operation's completion. Composability: You can combine multiple asynchronous operations using various methods like thenApply
, thenCompose
, or whenComplete
. This enables you to build complex asynchronous workflows from simpler building blocks. Result Handling: When the computation completes, its result becomes available. You can then handle this result using various methods like get()
, isCompleted()
, or join()
. Cancellation and Timeouts: CompletableFuture
provides APIs for cancelling an in-progress computation (e.g., with cancel()
) and setting timeouts to ensure the computation doesn't take too long.
Some common use cases for CompletableFuture
include:
To get started with CompletableFuture
, you can create a new instance using the static factory methods like supplyAsync()
or runAsync()
. Then, use various completion stages to compose and handle your asynchronous computations.
Here's a simple example:
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.ExecutionException;
public class Example {
public static void main(String[] args) throws ExecutionException, InterruptedException {
CompletableFuture future = CompletableFuture.supplyAsync(() -> {
// Perform an operation that takes some time
return "Result";
});
String result = future.get(); // Blocks until the computation completes
System.out.println("Final result: " + result);
}
}
In this example, we create a CompletableFuture
using supplyAsync()
and execute a simple operation (represented by an anonymous lambda). The get()
method blocks until the computation finishes, then returns the result.
Remember, when working with CompletableFuture
, it's essential to handle exceptions correctly. Java 8 provides the whenComplete()
method to achieve this.
I hope this explanation helps you understand the basics of CompletableFuture
in Java!