How to create XML using Java?
How to create XML using Java?
I'll respond in English for this question.
Creating an XML file using Java is a straightforward process that can be achieved through various APIs and libraries. Here, we will use the popular Apache Xerces library, which is part of the Apache XML Project.
Step 1: Install Apache Xerces
To get started, you need to download and install Apache Xerces from the official website:
https://xml.apache.org/xerces-j/
The installation process for Xerces is straightforward. Just follow the instructions provided on the website.
Step 2: Set up the Project
Create a new Java project in your preferred Integrated Development Environment (IDE) or text editor. Add the necessary JAR files to your classpath:
xercesImpl.jar
and xmlParserAPIs.jar
for Apache Xerces java.xml.jar
for built-in Java XML APIs
Step 3: Create an XML Document
Using Apache Xerces, you can create an XML document using the following code:
import org.apache.xerces.dom.DocumentImpl;
import org.w3c.dom.Element;
import org.w3c.dom.Text;
public class CreateXML {
public static void main(String[] args) {
// Create a new XML Document
DocumentImpl doc = new DocumentImpl();
// Create the root element
Element rootElement = doc.createElement("root");
// Add some child elements to the root element
Element child1 = doc.createElement("child1");
Element child2 = doc.createElement("child2");
Text textChild1 = doc.createTextNode("Hello, World!");
Text textChild2 = doc.createTextNode("This is a test XML file!");
child1.appendChild(textChild1);
child2.appendChild(textChild2);
rootElement.appendChild(child1);
rootElement.appendChild(child2);
// Write the XML document to a file
String xmlString = doc.getTextContent();
System.out.println(xmlString);
}
}
In this example, we create an xmlDocumentImpl
object and use its methods (createElement
, createTextNode
, appendChild
) to construct an XML document. Finally, we write the XML content to the console for display purposes.
Step 4: Write the XML Document to a File
To write the XML content to a file instead of printing it to the console, you can modify the code as follows:
import org.apache.xerces.dom.DocumentImpl;
import org.w3c.dom.Element;
import org.w3c.dom.Text;
public class CreateXML {
public static void main(String[] args) {
// Create a new XML Document
DocumentImpl doc = new DocumentImpl();
// ... (same as previous steps)
String xmlString = doc.getTextContent();
try (Writer writer = new FileWriter("example.xml")) {
writer.write(xmlString);
} catch (IOException e) {
System.err.println("Error writing to file: " + e.getMessage());
}
}
}
In this updated code, we use the FileWriter
class from the java.io
package to write the XML content to a file named example.xml
.
Conclusion
In this tutorial, we've covered the basics of creating an XML document using Apache Xerces in Java. You can modify this example to create more complex XML structures or integrate it with other applications for data exchange purposes.
Please let me know if you have any further questions or require more detailed information on a specific topic!
How to create XML using Java?
I'd be happy to help!
Creating an XML (Extensible Markup Language) file using Java can be achieved through various methods, including:
Using DOM (Document Object Model): This approach involves creating a Document object and then appending the necessary elements and attributes using the NodeList interface. Using StAX (Streaming API for XML): This method is more efficient than the DOM approach as it allows you to process XML documents in a streaming fashion, reducing memory usage. Using XOM (XML Object Model): This library provides a high-level abstraction over the underlying XML document and can simplify the task of creating an XML file.Here's an example of how to create an XML file using the DOM approach:
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import org.w3c.dom.Text;
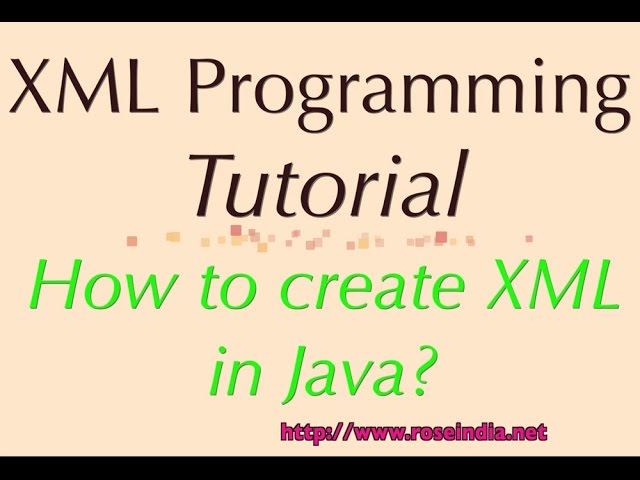
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import java.io.File;
import java.io.FileWriter;
import java.io.IOException;
public class XmlGenerator {
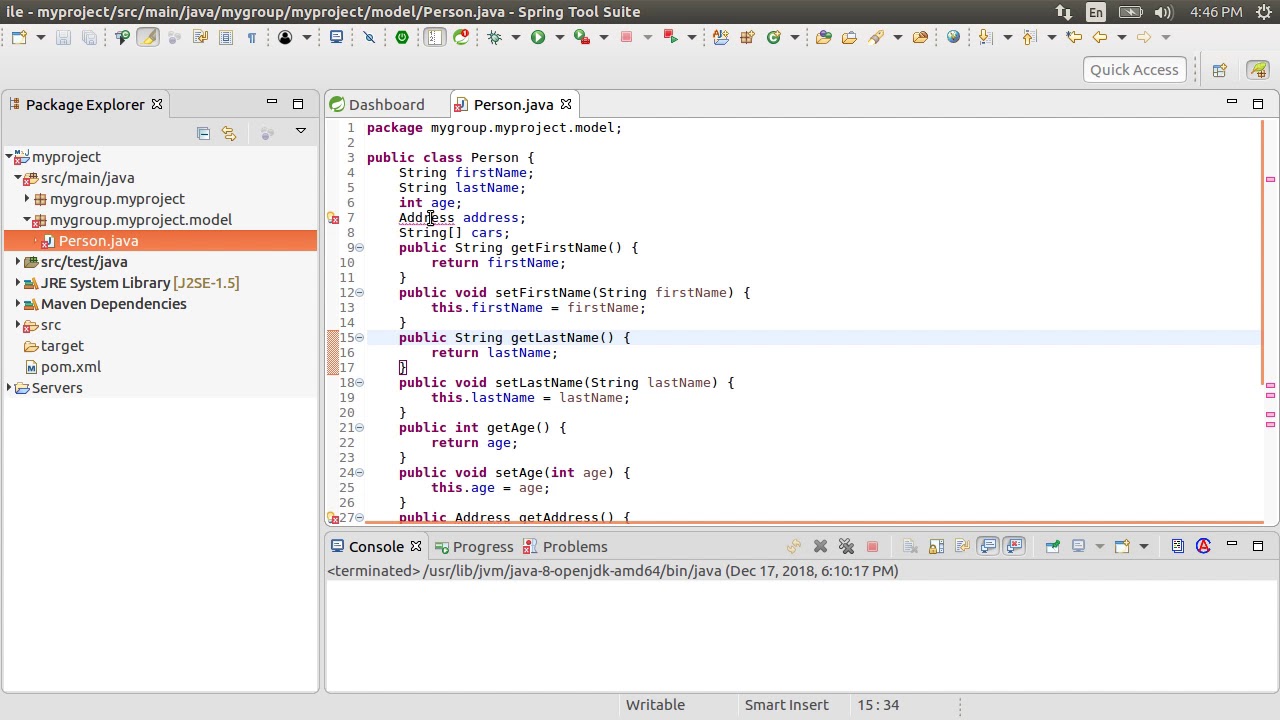
public static void main(String[] args) throws IOException {
// Create a DocumentBuilder and DocumentFactory
DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance();
DocumentBuilder builder = factory.newDocumentBuilder();
// Create the root element
Document document = builder.newDocument();
Element rootElement = document.createElement("root");
document.appendChild(rootElement);
// Append child elements to the root element
Element child1 = document.createElement("child1");
Element child2 = document.createElement("child2");
// Add attributes and text content to the child elements
child1.setAttribute("attr1", "value1");
child1.setAttribute("attr2", "value2");
Text textContent = document.createTextNode("Text Content 1");
child1.appendChild(textContent);
rootElement.appendChild(child1);
rootElement.appendChild(child2);
// Write the XML file to disk
FileWriter writer = new FileWriter(new File("example.xml"));
document.writeTo(writer);
writer.close();
}
}
This example creates a basic XML file with a single root element and two child elements. You can customize this code to fit your specific requirements.
For more complex XML structures, consider using an existing library such as XOM or DOM4J, which provide higher-level APIs for manipulating XML documents.
Remember that when creating an XML file, you should ensure that the resulting file adheres to the relevant XML syntax and naming conventions.