Java http_proxy environment variable
Java http_proxy environment variable
I'll respond in English as requested.
The http_proxy
environment variable is used to specify a proxy server that the system should use when making HTTP or HTTPS requests. This is often needed when accessing resources behind a corporate firewall or when testing web applications that require a specific proxy setup.
In Java, you can set the http_proxy
environment variable in various ways depending on your development environment and needs. Here are some common approaches:
-D
flag followed by the name of the property and its value. For example:
java -Dhttp_proxy=http://myproxy:8080 MyJavaProgram
This sets the http_proxy
environment variable to http://myproxy:8080
for the duration of the Java program.
set http_proxy=http://myproxy:8080
java MyJavaProgram
In Unix-like systems (including Linux and macOS):
export http_proxy=http://myproxy:8080
java MyJavaProgram
Java Properties File: You can also configure the http_proxy
environment variable using a Java properties file. Create a props.properties
file with the following content:
http_proxy=http://myproxy:8080
Then, use the following code to read the property file and set the http_proxy
environment variable:
import java.io.FileInputStream;
import java.io.IOException;
import java.util.Properties;
public class MyJavaProgram {
public static void main(String[] args) throws IOException {
Properties props = new Properties();
props.load(new FileInputStream("props.properties"));
System.setProperty("http_proxy", props.getProperty("http_proxy"));
// Rest of your code here
}
}
HTTP Proxy URL: If you're using Java's built-in URL
class, you can set the proxy URL explicitly when creating a new URL
object:
import java.net.URL;
public class MyJavaProgram {
public static void main(String[] args) {
URL url = new URL("http://myurl.com");
URLConnection conn = url.openConnection();
conn.setProxy(new URL("http://myproxy:8080"));
// Rest of your code here
}
}
In summary, you can set the http_proxy
environment variable in Java using system properties, environment variables, a Java properties file, or by setting the proxy URL explicitly when creating a new URL
object. Each approach has its own strengths and weaknesses, and you should choose the one that best fits your specific use case.
How to pass JVM arguments in Java code?
Passing JVM arguments in Java code is a straightforward process. There are several ways to achieve this:
1. Using the main
method
In your main
method, you can access the command-line arguments using the String[] args
parameter. This method works well for small-scale applications or when you want to pass specific parameters.
Here's an example:
public class Main {
public static void main(String[] args) {
// Accessing the command-line arguments
for (int i = 0; i < args.length; i++) {
System.out.println("Argument " + i + ": " + args[i]);
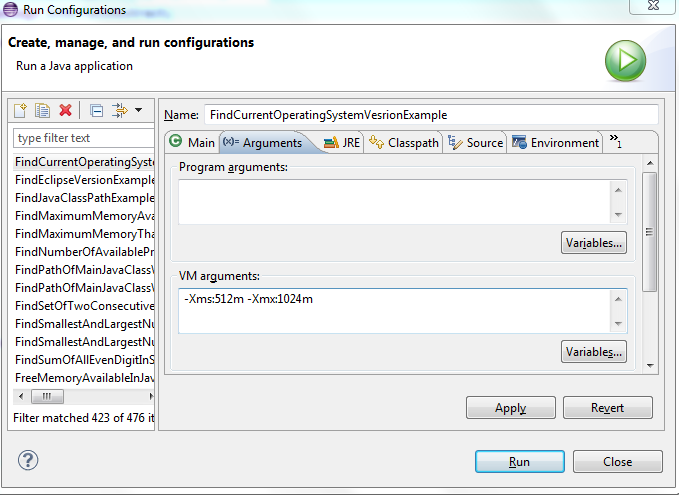
}
}
}
2. Using system properties
Java provides a way to access system properties using the System.getProperty()
method. This approach is useful when you want to pass configuration settings or environment variables.
Here's an example:
public class Main {
public static void main(String[] args) {
String propertyValue = System.getProperty("my.property");
// Use the property value as needed
}
}
3. Using a properties file
You can store JVM arguments in a properties file (e.g., config.properties
) and load them using the Properties
class.
Here's an example:
public class Main {
public static void main(String[] args) {
Properties props = new Properties();
try {
props.load(new FileInputStream("config.properties"));
String propertyValue = props.getProperty("my.property");
// Use the property value as needed
} catch (Exception e) {
e.printStackTrace();
}
}
}
4. Using an external configuration file
You can store JVM arguments in an external configuration file (e.g., a JSON or XML file) and load them using a library like Jackson or XStream.
Here's an example with Jackson:
public class Main {
public static void main(String[] args) {
ObjectMapper mapper = new ObjectMapper();
try {
JsonNode config = mapper.readTree(new File("config.json"));
String propertyValue = config.get("my.property").asText();
// Use the property value as needed
} catch (Exception e) {
e.printStackTrace();
}
}
}
When running your Java program, you can pass JVM arguments using the command line. For example:
java -Dmy.property=value Main
In this example, my.property
is a system property set to the value "value"
. You can access this property in your code using System.getProperty("my.property")
.
Remember to always follow best practices for storing sensitive information and configuration settings.