Java runnable with parameter example
Java runnable with parameter example
I'd be happy to help you with that.
Let's talk about Java, a popular programming language used for developing large-scale applications, including Android apps. One of the key features of Java is its ability to accept parameters when creating an instance of a class or invoking a method. This allows you to pass specific values to methods and constructors, which can greatly enhance the flexibility and reusability of your code.
Let's take a simple example to demonstrate how this works. Suppose we have a Person
class with a constructor that takes two parameters: name
and age
. Here's an example:
public class Person {
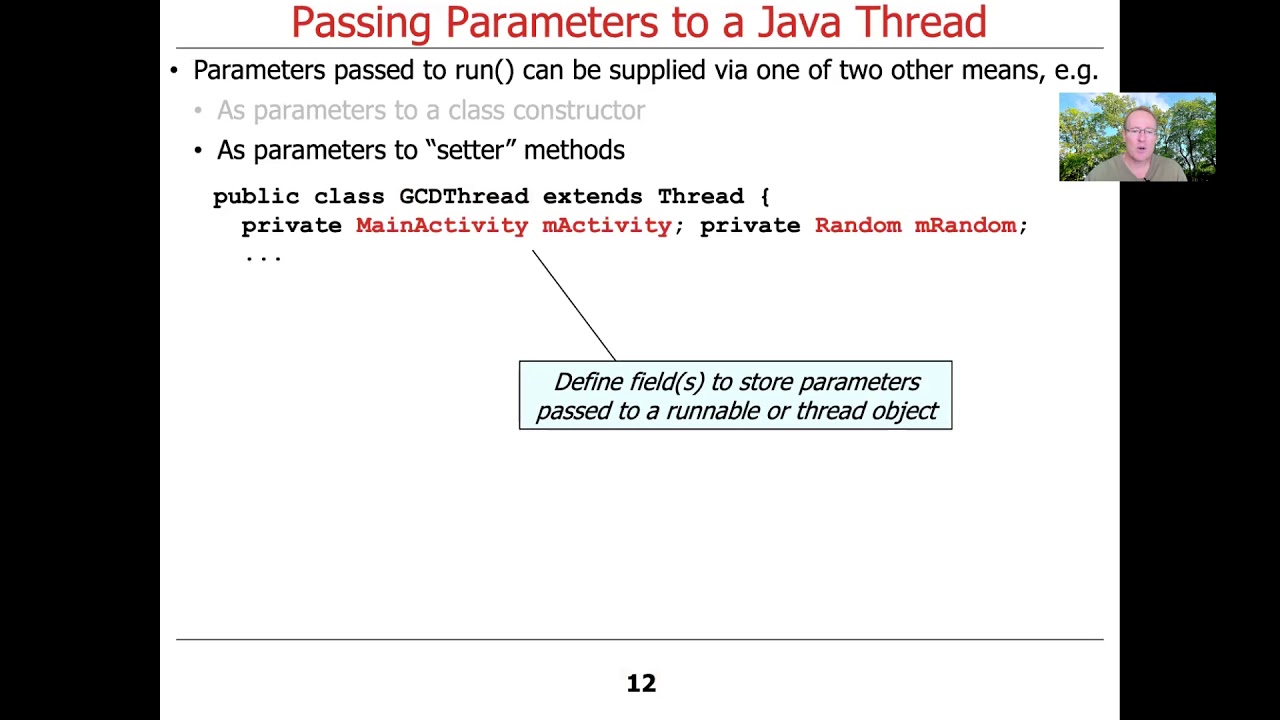
private String name;
private int age;
public Person(String name, int age) {
this.name = name;
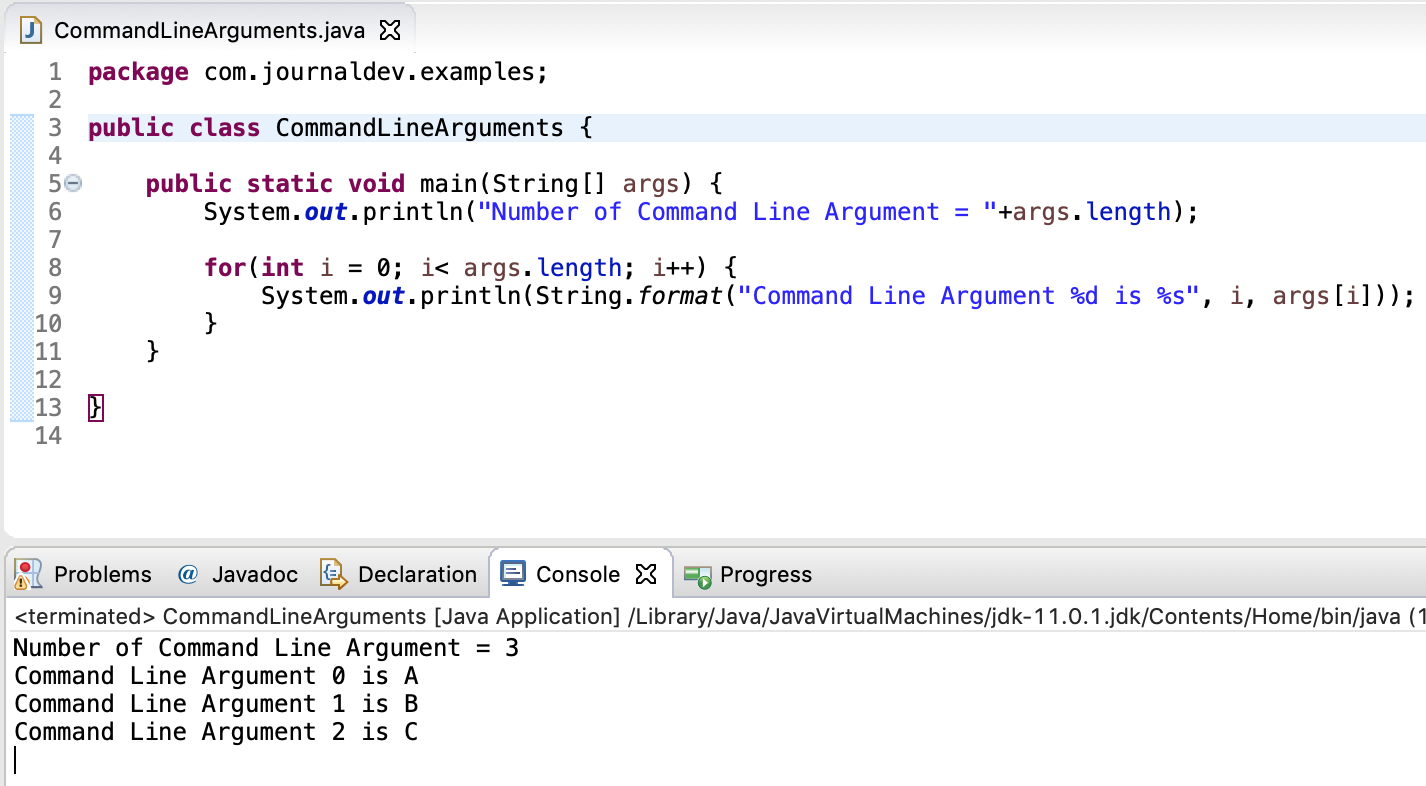
this.age = age;
}
public void sayHello() {
System.out.println("Hello, my name is " + name + " and I'm " + age + " years old!");
}
}
Now, let's create an instance of the Person
class with specific parameters:
public static void main(String[] args) {
Person person1 = new Person("John", 30);
Person person2 = new Person("Jane", 25);
person1.sayHello();
person2.sayHello();
}
In this example, we create two instances of the Person
class with different parameters: person1
has a name of "John" and an age of 30, while person2
has a name of "Jane" and an age of 25. When we call the sayHello()
method on each instance, it will output a greeting message with the specific values passed to the constructor.
Here's another example that demonstrates how you can pass parameters to methods:
public class Calculator {
public int add(int a, int b) {
return a + b;
}
public static void main(String[] args) {
Calculator calculator = new Calculator();
int result1 = calculator.add(2, 3);
int result2 = calculator.add(5, 7);
System.out.println("Result 1: " + result1);
System.out.println("Result 2: " + result2);
}
}
In this example, we create a Calculator
class with an add()
method that takes two parameters: a
and b
. We then create an instance of the Calculator
class and call the add()
method with different parameter values to calculate the results.
These are just simple examples to illustrate how Java handles parameters. In real-world applications, you can pass parameters to constructors, methods, and even lambda expressions to create more complex and dynamic code.
Java pass function as parameter
I'm happy to respond in English!
Passing a function as a parameter is a concept commonly known as Higher-Order Functions (HOFs). This is where you treat functions as first-class citizens, meaning they can be passed around like any other variable.
In Java, we can achieve this using lambda expressions or method references. Here's an example of how to do it with lambda expressions:
interface MathOperation {
int operate(int num1, int num2);
}
public class Main {
private static int calculateSomething(MathOperation mathOperation) {
return mathOperation.operate(10, 20);
}
public static void main(String[] args) {
// Example usage with lambda expression
MathOperation addition = (int a, int b) -> a + b;
int result1 = calculateSomething(addition);
System.out.println("Result of addition: " + result1);
// Example usage with method reference
MathOperation subtraction = Integer::sum;
int result2 = calculateSomething(subtraction);
System.out.println("Result of subtraction: " + result2);
}
}
In the example above, MathOperation
is an interface that defines a single abstract method (operate(int num1, int num2)
). We then create two lambda expressions, one for addition and another for subtraction. These lambda expressions can be passed into the calculateSomething(MathOperation mathOperation)
function.
When we call calculateSomething(addition)
, it's equivalent to calling addition.operate(10, 20)
, which adds 10 and 20 together. Similarly, when we call calculateSomething(subtraction)
, it's equivalent to calling subtraction.operate(10, 20)
, which is shorthand for Integer.sum.applyAsInt(10, 20)
.
This example demonstrates how Java allows you to pass functions as parameters using lambda expressions and method references. This can be useful when you want to perform a specific operation depending on some condition or user input, making your code more flexible and reusable.
For instance, if we were building a financial application that calculates the interest rate based on the user's investment strategy, we could create an interface InterestRateCalculator
with different implementations for each strategy (e.g., fixed rate, variable rate, etc.). Then, in our main application logic, we could pass these calculators as parameters to perform calculations and generate reports.
In summary, passing functions as parameters in Java is a powerful technique that allows you to decouple your code and make it more flexible, reusable, and maintainable. By using lambda expressions or method references, you can create interfaces for different operations and then call them dynamically, making your code more scalable and efficient.