What is the difference between collect and map in Java stream?
What is the difference between collect and map in Java stream?
In Java, Stream API provides two fundamental operations: collect()
and map()
. Both are used to process data streams, but they serve distinct purposes.
map()
:
The map()
function is used to transform or project each element of the stream into a new type. It's like applying a mathematical mapping function to each element. This operation does not change the overall number of elements in the stream, only their form or structure. Think of it as a data transformation.
Example:
Stream stream = Stream.of("Hello", "World");
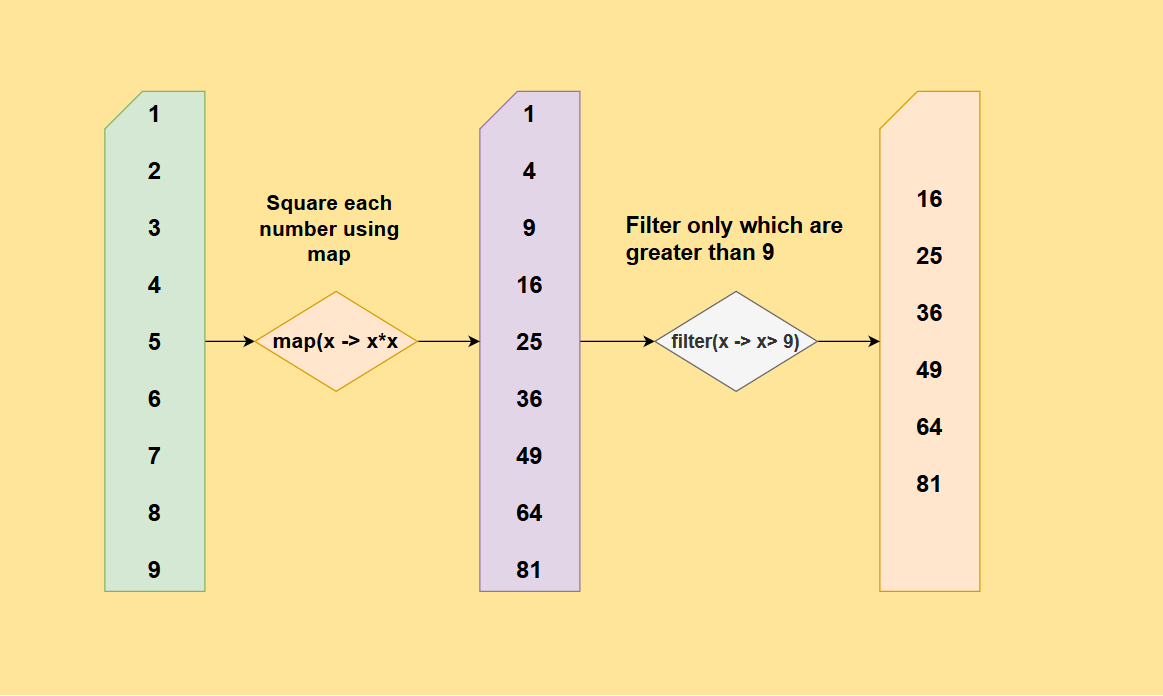
stream.map(s -> s.toUpperCase())
.forEach(System.out::println);
In this example, the map()
function is used to convert each string in the stream to uppercase. The resulting stream will have the same number of elements as the original, but with transformed values.
collect()
:
The collect()
function is used to accumulate or combine the elements of a stream into a summary form, such as a collection (e.g., List
, Set
, or Map
), an aggregate value, or a reduced representation. This operation can significantly change the number of elements in the stream, depending on how you implement the collector.
Example:
Stream stream = Stream.of("Hello", "World");
stream.collect(Collectors.toList())
.forEach(System.out::println);
In this example, the collect()
function is used to accumulate all the strings in the stream into a single List
object. The resulting collection will contain the same elements as the original stream.
Key differences:
Purpose:map()
transforms individual elements, while collect()
aggregates or accumulates multiple elements. Output: map()
produces a new stream with potentially transformed values, whereas collect()
returns a single value or collection. Stream size: map()
typically does not change the number of elements in the stream, while collect()
can reduce or combine elements into fewer representations.
When to use each:
Usemap()
when you need to transform individual data elements and retain the original stream size. Use collect()
when you want to aggregate or accumulate data values into a single representation or collection.
In summary, map()
is used for element-wise transformations, while collect()
is used for aggregation and accumulation. Understanding these differences will help you effectively utilize Java Stream API in your programming endeavors!
What is the difference between stream api and collection in java
I'm glad you asked!
The Stream
API and Collection
are two fundamental concepts in Java programming, serving distinct purposes in handling data structures. Understanding the differences between them will help you choose the right tool for your specific needs.
What is a Collection?
A Collection
is a foundational data structure in Java that allows you to store and manipulate groups of objects. A collection can be thought of as an ordered or unordered set of elements, where each element can be accessed by its index (for arrays) or via iteration (for lists).
The primary characteristics of collections are:
Mutable: You can add, remove, or modify elements in a collection. Ordered or Unordered: Collections can maintain the order in which elements were added, or they can be unordered, like sets. Homogeneous: A collection typically contains objects of the same class or type.Some examples of collections include ArrayList
, LinkedList
, HashSet
, and HashMap
.
What is a Stream?
A Stream
is a new data processing concept introduced in Java 8, which provides a pipeline for processing data structures. A stream represents a sequence of elements supporting parallel and pipelined operations.
The primary characteristics of streams are:
Immutability: Once created, a stream cannot be modified; it can only be consumed. Stateful: Streams maintain internal state between operations, allowing for efficient processing and pipelining. Lazy: Stream operations are executed on demand (when needed), which helps improve performance.Streams enable you to perform various operations on your data, such as:
Filtering: selecting specific elements Mapping: transforming elements Reducing: combining elements into a summary value Sorting: arranging elements in orderSome examples of stream-related classes include Stream
, IntStream
, and Map
.
Key differences between Stream API and Collection
Immutability vs. Mutability: Collections are generally mutable, while streams are immutable.In summary:
UseCollection
when you need to store and manipulate groups of objects with mutable behavior. Choose the Stream
API for processing data structures in a pipeline fashion, leveraging parallelism, and promoting functional programming techniques.
Now that you know the differences between these two fundamental concepts, choose wisely!