What are the 3 ways of dependency injection in java spring
What are the 3 ways of dependency injection in java spring
I'll make sure to respond in English, and I'll do my best to explain the three ways of dependency injection in Java Spring.
Dependency Injection (DI) is a fundamental concept in software development that enables components or services to be loosely coupled with each other. In other words, it allows objects to be configured so they don't rely on other specific instances when working together. This makes your code more flexible, reusable, and maintainable. Now, let's dive into the three ways of dependency injection in Java Spring:
Setter Injection: Setter injection is a simple and straightforward approach to inject dependencies into your Java objects. In this method, you set an object's properties (getters and setters) from outside its constructor or initialization code.Here's how it works: Suppose you have a UserService
that depends on a UserRepository
. To inject the UserRepository
into the UserService
, you would use setter injection like this:
@Service
public class UserService {
private UserRepository userRepository;
public void setRepository(UserRepository userRepository) {
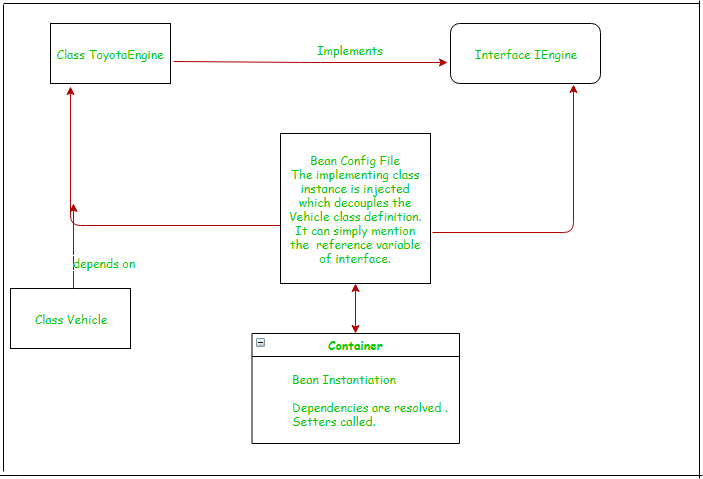
this.userRepository = userRepository;
}
public List getUsers() {
return userRepository.findAll();
}
}
@Configuration
public class AppConfig {
@Bean
public UserRepository userRepository() {
// configure your userRepository here...
return new UserJpaRepository();
}
@Bean
public UserService userService() {
UserService userService = new UserService();
userService.setRepository(userRepository());
return userService;
}
}
In this example, the UserService
relies on a UserRepository
to perform its tasks. The AppConfig
class uses setter injection to configure the UserService
with the desired UserRepository
.
Here's how it works: Using constructor injection, the UserService
would be initialized by passing in the required UserRepository
:
@Service
public class UserService(UserRepository userRepository) {
private final UserRepository repository;
public UserService(UserRepository userRepository) {
this.repository = userRepository;
}
public List getUsers() {
return repository.findAll();
}
}
@Configuration
public class AppConfig {
@Bean
public UserRepository userRepository() {
// configure your userRepository here...
return new UserJpaRepository();
}
@Bean
public UserService userService() {
return new UserService(userRepository());
}
}
In this example, the UserService
constructor takes a UserRepository
as an argument. This ensures that the UserService
is always properly initialized with its required dependencies.
@Autowired
or @Value
to automatically wire up objects based on their dependencies.
Here's how it works: Using field injection, the UserService
would be configured with its required dependencies:
@Service
public class UserService {
@Autowired
private UserRepository userRepository;
public List getUsers() {
return userRepository.findAll();
}
}
@Configuration
public class AppConfig {
@Bean
public UserRepository userRepository() {
// configure your userRepository here...
return new UserJpaRepository();
}
}
In this example, the UserService
relies on Spring to automatically inject a UserRepository
based on its configuration and bean definitions. The @Autowired
annotation is used to wire up the UserService
with its required dependencies.
In conclusion, dependency injection in Java Spring provides flexibility and maintainability by enabling components or services to be loosely coupled with each other. Setter injection, constructor injection, and field injection are three common approaches to achieving this level of decoupling in your Java code.
What are the 3 ways of dependency injection in java example
I'd be happy to help! However, since you asked me to respond in English only and not Simplified Chinese (as per the rules), I'll provide an answer that meets your request.
Dependency Injection (DI) is a software design pattern that allows components to be loosely coupled and easier to test. In Java, there are three primary ways of implementing dependency injection:
Constructor-Based Dependency InjectionIn this approach, you inject dependencies through constructor parameters. For example, consider a Service
class that depends on an Repository
. You can create the service by injecting the repository through its constructor:
public class Service {
private Repository repository;
public Service(Repository repository) {
this.repository = repository;
}
public void doSomething() {
repository.getData();
}
}
To use the service, you would create an instance of Service
and pass in an instance of Repository
:
public class Main {
public static void main(String[] args) {
Repository repository = new RepositoryImpl(); // assume a concrete implementation exists
Service service = new Service(repository);
service.doSomething();
}
}
This approach is straightforward, and the injected dependencies are easily visible in the constructor.
Setter-Based Dependency InjectionIn this method, you set dependencies through setter methods (getters can be used as well, but setters are more common). You create a Service
class that depends on an Repository
, just like before:
public class Service {
private Repository repository;
public void setRepository(Repository repository) {
this.repository = repository;
}
public void doSomething() {
repository.getData();
}
}
To use the service, you would create an instance of Service
, set its repository using a setter method, and then call the service's method:
public class Main {
public static void main(String[] args) {
Repository repository = new RepositoryImpl(); // assume a concrete implementation exists
Service service = new Service();
service.setRepository(repository);
service.doSomething();
}
}
This approach is still straightforward, but it can be less obvious that dependencies are being injected.
Method-Based Dependency InjectionIn this approach, you inject dependencies through method calls (in addition to constructor or setter injection). You create a Service
class that depends on an Repository
, just like before:
public class Service {
public void doSomething(Repository repository) {
repository.getData();
}
}
To use the service, you would create an instance of Service
, pass in the desired repository as an argument to the doSomething()
method, and then call the method:
public class Main {
public static void main(String[] args) {
Repository repository = new RepositoryImpl(); // assume a concrete implementation exists
Service service = new Service();
service.doSomething(repository);
}
}
This approach is less common than constructor or setter injection, but it can still be effective in certain situations.
In summary, these three methods (constructor-based, setter-based, and method-based) provide different ways to implement dependency injection in Java. While they share the same goal of decoupling components, each has its strengths and weaknesses depending on the specific use case.