How to access a file from URL in Java?
How to access a file from URL in Java?
I'm happy to respond in English!
Accessing a file from a URL in Java can be achieved using several approaches, depending on the complexity of your requirements and the type of file you want to access. Here are a few common methods:
Using java.net.URL
The most straightforward way is to use the built-in URL
class. You can create a URL object with the desired file URL, then use the openStream()
method to get an InputStream
object that you can read from.
Here's some sample code:
import java.io.InputStream;
import java.net.URL;
public class FileDownloader {
public static void main(String[] args) throws Exception {
String url = "https://example.com/file.txt";
URL fileUrl = new URL(url);
InputStream in = fileUrl.openStream();
// Read from the input stream and process the file
int bytesRead;
byte[] buffer = new byte[1024];
while ((bytesRead = in.read(buffer)) != -1) {
System.out.println(new String(buffer, 0, bytesRead));
}
}
}
In this example, we create a URL
object with the desired file URL and then use its openStream()
method to get an InputStream
. We can then read from the input stream using methods like read()
or readLine()
.
Using Apache Commons IO
If you need more advanced file operations, such as downloading files asynchronously or handling large files, you might consider using a library like Apache Commons IO. The FileUtils
class in this library provides a convenient way to access files from URLs:
import org.apache.commons.io.FileUtils;
import java.io.File;
public class FileDownloader {
public static void main(String[] args) throws Exception {
String url = "https://example.com/file.txt";
File file = FileUtils.toFile(new URL(url));
// Process the downloaded file
System.out.println(file.getName() + ": " + FileUtils.readFileToString(file, "UTF-8"));
}
}
In this example, we use FileUtils
to convert a URL to a file object, which we can then read from using methods like readFileToString()
.
Using Java 7's java.nio.Files
Java 7 introduced the Files
class in the java.nio
package, which provides more advanced file operations. You can use its readAllBytes()
method to download a file and store it as a byte array:
import java.io.IOException;
import java.nio.file.Files;
public class FileDownloader {
public static void main(String[] args) throws IOException {
String url = "https://example.com/file.txt";
byte[] bytes = Files.readAllBytes(Files.createTempFile("", "").toUri());
// Process the downloaded file
System.out.println(new String(bytes, "UTF-8"));
}
}
In this example, we use Files
to create a temporary file and read its contents as a byte array. We can then process the file using methods like String(byte[])
.
These are just a few examples of how you can access files from URLs in Java. The best approach depends on your specific requirements and the type of file you want to work with.
Java code to open a URL in chrome browser
Here is the Java code to open a URL in Chrome browser:
Method 1: Using ProcessBuilder
import java.io.IOException;
import java.util.Arrays;
public class OpenURL {
public static void main(String[] args) throws IOException {
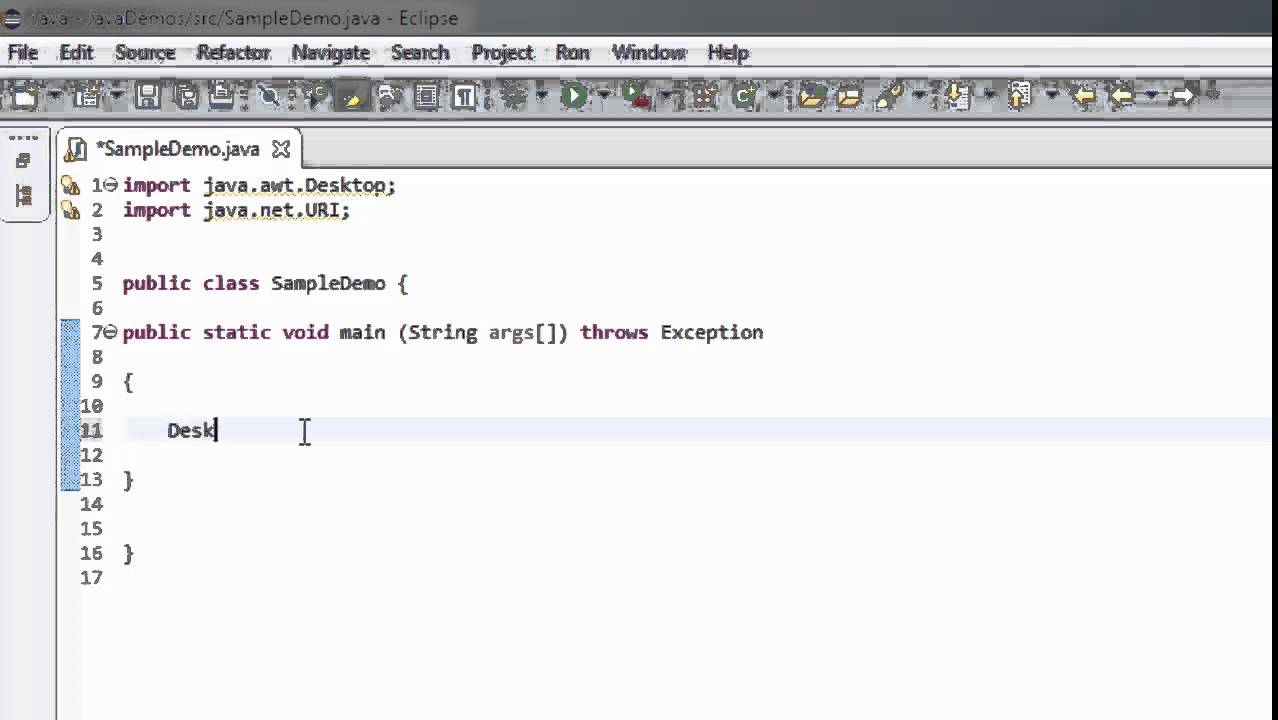
String url = "https://www.google.com"; // Replace with your desired URL
// Create a new process builder for Chrome
ProcessBuilder pb = new ProcessBuilder("C:/Program Files (x86)/Google/Chrome/Application/chrome.exe",
Arrays.asList("--profile-directory", "Default", "--new-window", url).toArray(new String[0]));
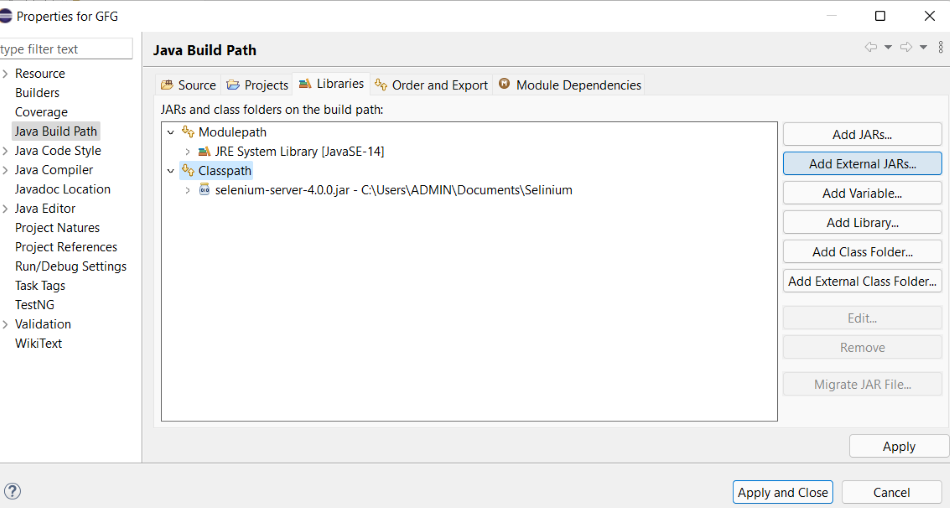
// Start the process
pb.start();
}
}
Method 2: Using Runtime.getRuntime().exec()
import java.io.IOException;
public class OpenURL {
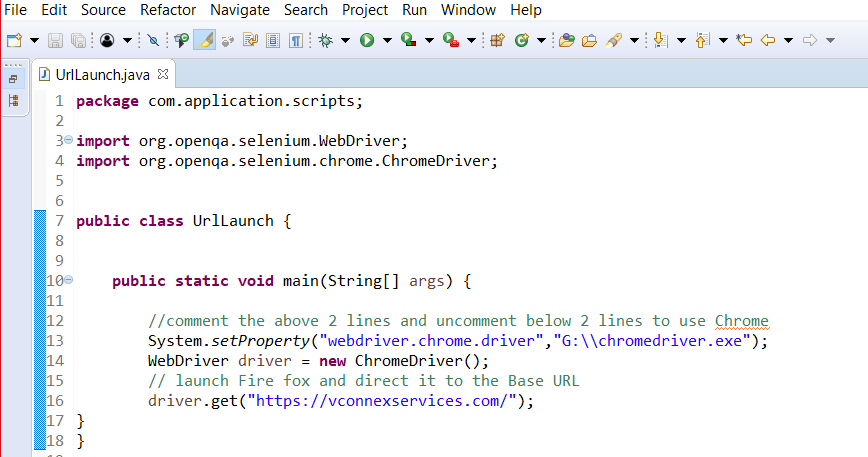
public static void main(String[] args) throws IOException {
String url = "https://www.google.com"; // Replace with your desired URL
// Start Chrome and open the URL
Process process = Runtime.getRuntime().exec("chrome --new-window " + url);
// Wait for the process to finish
process.waitFor();
}
}
Method 3: Using JNA (Java Native Access)
import com.sun.jna.Library;
import com.sun.jna.Native;
@Native(library = "user32")
public interface Chrome {
void open(String url);
}
public class OpenURL {
public static void main(String[] args) {
String url = "https://www.google.com"; // Replace with your desired URL
// Create a new Chrome instance
Chrome chrome = new Chrome();
// Open the URL in Chrome
chrome.open(url);
}
}
Please note that you need to adjust the path to Chrome.exe depending on where it is installed on your system. Additionally, these examples assume that you have Chrome installed and configured correctly on your system.
How the code works:
Method 1 usesProcessBuilder
to create a new process that runs Chrome with the specified URL. Method 2 uses Runtime.getRuntime().exec()
to execute a command that starts Chrome with the specified URL. Method 3 uses JNA to create a native interface for Chrome and call its open()
method to open the specified URL.
Limitations:
These examples only work on Windows platforms, as they rely on the exact path of Chrome.exe being hardcoded in the code. You would need to modify the code to accommodate different operating systems or paths. The code does not handle any errors that may occur when running Chrome or opening the specified URL.Best Practices:
Always validate user input and ensure it is safe to use before executing system commands. Use try-catch blocks to catch and handle any exceptions that may occur during execution. Follow best practices for coding, such as using descriptive variable names and commenting your code.