What is \\\\\\\\\\\\\\\\ w in Java?
What is w in Java?
I'd be happy to explain what w
is in Java!
In Java, w
is a regular expression (regex) character class that matches any word character. A "word character" is defined as an alphanumeric character (letters or digits) and underscores (_
). This means that w
will match any string of characters that consists only of letters, digits, or underscores.
Here are some examples to illustrate what w
matches:
hello
: matches because it's a sequence of alphanumeric characters 123abc
: matches because it's a sequence of alphanumeric characters and underscore _my_var
: matches because it's a sequence of alphanumeric characters and underscore
On the other hand, w
will not match strings that contain special characters (like .
, (
, )
, [
, ]
, {
, }
, etc.) or whitespace characters.
Here are some examples to illustrate what w
does NOT match:
hello world
: does not match because it contains a space
abc@def
: does not match because it contains an @
(abc)
: does not match because it contains parentheses
In Java, you can use the w
character class in regular expressions (regex) to search for patterns in strings. For example:
String input = "hello_world";
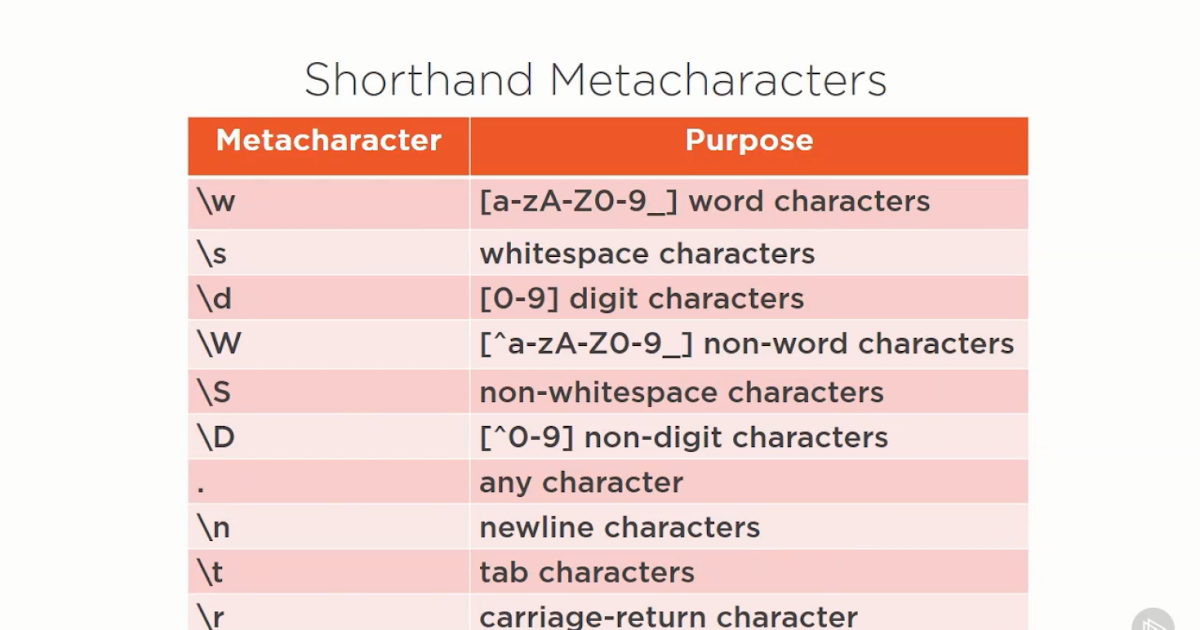
Pattern pattern = Pattern.compile("w+");
Matcher matcher = pattern.matcher(input);
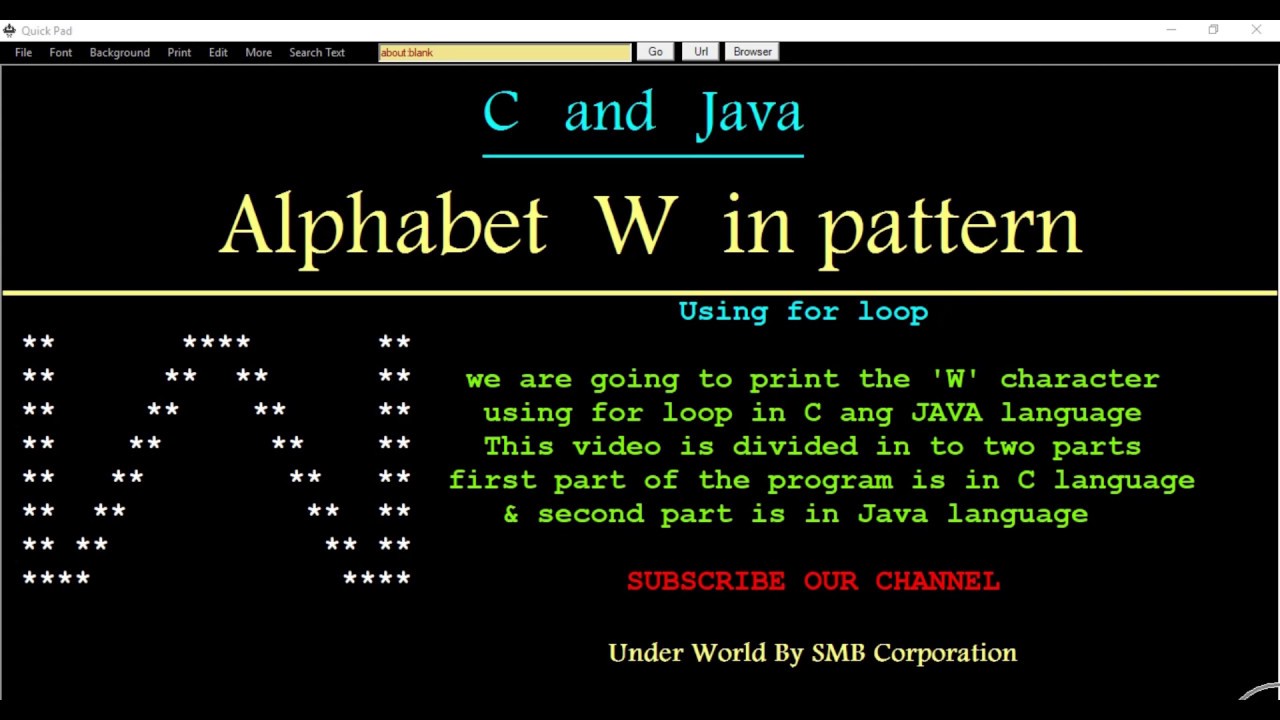
if(matcher.matches()) {
System.out.println("Matched!");
}
In this example, the regex w+
will match one or more word characters (hello_world
) in the input string.
So, to summarize, w
is a regex character class that matches any word character (alphanumeric characters and underscore) in Java.
How to generate regex expressions in Java?
I'll respond in English since that's what you requested!
In Java, regular expressions (regex) are used to match patterns in strings. You can use the java.util.regex
package to create and work with regex expressions.
Here's a step-by-step guide on how to generate regex expressions in Java:
Method 1: Using String Patterns
You can create simple regex expressions by using string patterns. For example, if you want to match all strings that start with "hello", you can use the following code:
String regex = "^hello";
In this case, ^
matches the start of a line, and hello
is the literal pattern.
Method 2: Using Pattern Class
The Pattern
class provides more flexibility when creating regex expressions. You can create patterns using the Pattern.compile()
method:
Pattern pattern = Pattern.compile("hello");
This will match all strings that contain the literal "hello".
Method 3: Using Character Classes
You can use character classes (such as [abc]
to match any of the characters within the square brackets) and anchors (such as ^
or $
) to create more complex regex expressions.
For example, if you want to match all strings that contain only letters and spaces, you can use:
String regex = "[a-zA-Z s]*";
Here, [a-zA-Z s]*
matches any character (letter or space) zero or more times (*
).
Method 4: Using Quantifiers
Quantifiers (such as +
, ?
, and {}
) allow you to specify the number of times a pattern should match.
For example, if you want to match all strings that contain at least one "o" followed by zero or more letters, you can use:
String regex = "o[abc]+";
Here, o
is the literal character, [abc]+
matches any letter (one or more times), and the whole expression is wrapped in parentheses (()
).
Method 5: Using Groups
Groups (using parentheses) allow you to group patterns together and treat them as a single unit.
For example, if you want to match all strings that contain either "hello" or "world", you can use:
String regex = "(hello|world)";
Here, (hello|world)
is the pattern that matches either "hello" or "world".
Working with Regex Expressions
Once you've created a regex expression using one of the above methods, you can use the Matcher
class to search for matches in strings. For example:
String str = "Hello World!";
Pattern pattern = Pattern.compile("hello");
Matcher matcher = pattern.matcher(str);
if (matcher.find()) {
System.out.println("Match found!");
} else {
System.out.println("No match found.");
}
This code will search for the pattern "hello" in the string "Hello World!" and print a message if a match is found.
Additional Tips
Use thePattern
class's methods, such as compile()
or quote()
, to create regex expressions safely. When using character classes, be aware that some characters (such as .
, [
, and -
) have special meanings in regex expressions. You may need to escape them using backslashes (
). When creating complex patterns, use whitespace and parentheses to make your code more readable.
In conclusion, generating regex expressions in Java can be done through various methods, including string patterns, the Pattern
class, character classes, quantifiers, and groups. By understanding how to create and work with regex expressions, you can effectively search for patterns in strings using Java!