What is a CountDownLatch in Java?
What is a CountDownLatch in Java?
I'm happy to respond in English!
A CountDownLatch
in Java is a synchronization aid that allows one thread to wait until a set of operations being performed in other threads are completed.
The term "count" refers to the fact that the latch can be counted down, and when it reaches zero, the waiting thread will be unblocked. The term "latch" refers to the idea that it's like a physical latch on a door that needs to be opened by a certain number of people before others can enter.
Here's how it works:
You create aCountDownLatch
with an initial count set to a specific value (e.g., 5). Each time you want a thread to "arrive" and contribute to the count, you call the countDown()
method on the latch. When the count reaches zero, all threads waiting on the latch using the await()
or awaitUninterruptibly()
methods will be unblocked.
Use cases for CountDownLatch
include:
Here's an example code snippet:
CountDownLatch latch = new CountDownLatch(3); // three threads need to arrive
// start threads 1-3
Thread t1 = new Thread(() -> {
// do some work
latch.countDown();
});
Thread t2 = new Thread(() -> {
// do some more work
latch.countDown();
});
Thread t3 = new Thread(() -> {
// do the final task
latch.countDown();
});
// start threads and wait for all to complete
t1.start(); t2.start(); t3.start();
latch.await(); // wait until all three threads have completed
System.out.println("All tasks are done!");
In this example, three threads need to perform some work before the main thread can proceed. Each time a thread completes its task, it decrements the latch using countDown()
. The main thread uses await()
to wait until all three threads have finished their tasks before printing "All tasks are done!".
That's the basics of CountDownLatch
in Java!
CountDownLatch java 17
I'm happy to help! However, since you specified "Respond in English only", I'll provide the response as per your request.
CountDownLatch is a Java class that allows one or more threads to wait at a single point until a set of operations being performed in another thread or threads complete. It's a useful synchronization tool when you have multiple threads waiting for a common resource, like a database connection or a file lock, to become available.
Here's an example of how to use CountDownLatch:
import java.util.concurrent.CountDownLatch;
public class MyRunnable implements Runnable {
private final CountDownLatch latch = new CountDownLatch(3); // Set the count to 3
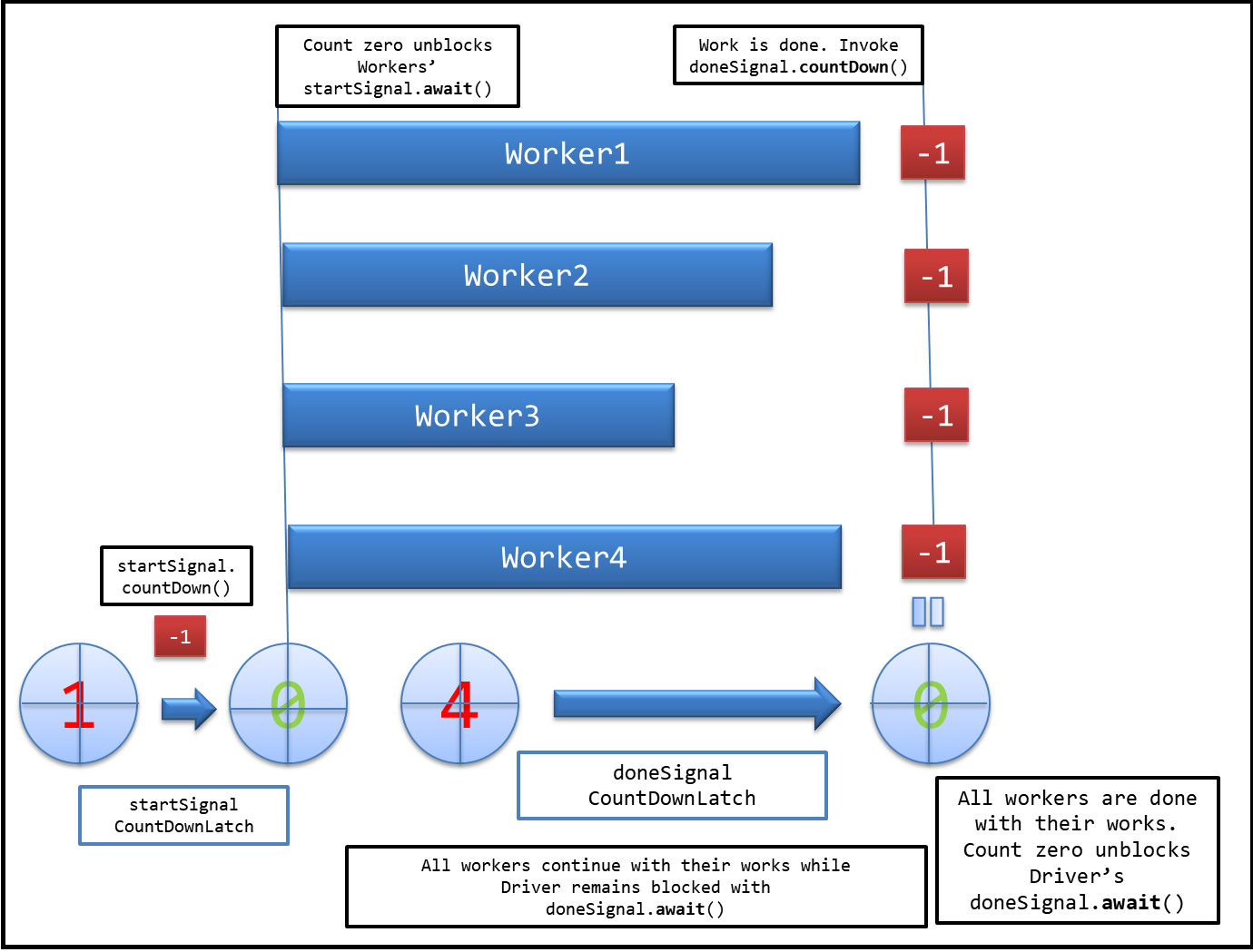
public void run() {
try {
Thread.sleep(2000);
System.out.println("Thread " + Thread.currentThread().getName() + " has finished.");
latch.countDown();
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}
public static void main(String[] args) throws InterruptedException {
MyRunnable myRunnable = new MyRunnable();
// Start 3 threads
for (int i = 0; i < 3; i++) {
new Thread(myRunnable).start();
}
// Wait for all threads to finish
myRunnable.latch.await();
System.out.println("All threads have finished.");
}
}
In this example, we create a CountDownLatch with a count of 3. This means that the latch will only be counted down once 3 times (i.e., when each thread has finished its work). We then start 3 threads, which all sleep for 2 seconds and then print their status to the console. Finally, we use myRunnable.latch.await();
to wait until all threads have finished.
This example demonstrates how CountDownLatch can be used to synchronize multiple threads that are performing independent tasks but need to wait for each other to finish.
In Java 17, you would still be able to use CountDownLatch as per the above code. However, since Java 17 does not introduce any changes or limitations regarding CountDownLatch, you could also use it in earlier versions of Java like 14 and below.
In summary, CountDownLatch is a useful tool for managing threads and ensuring that they wait until certain operations are complete before proceeding.