How to run a method asynchronously in Java Spring Boot
How to run a method asynchronously in Java Spring Boot
To run a method asynchronously in Java Spring Boot, you can use various approaches depending on the requirements and complexity of your application. Here are some common methods:
ExecutorService
instance to execute tasks asynchronously. For example:
@Configuration
public class AsyncConfig {
@Bean
public ExecutorService executor() {
return Executors.newFixedThreadPool(5);
}
}
@Service
public class MyService {
@Autowired
private ExecutorService executor;
public void myMethod() {
executor.submit(new MyTask());
}
class MyTask implements Runnable {
public void run() {
// your task code here
}
}
}
In this example, the myMethod
method submits a new instance of MyTask
to the ExecutorService
, which executes the task asynchronously.
@Async
to indicate that it should be executed asynchronously:
@Service
public class MyService {
@Async
public Future myMethod() {
// your task code here
return null;
}
}
In this case, Spring will automatically execute the myMethod
method in an asynchronous thread pool.
CompletableFuture
to write asynchronous code:
@Service
public class MyService {
public void myMethod() {
CompletableFuture future = CompletableFuture.supplyAsync(() -> {
// your task code here
return "Result";
});
try {
String result = future.get(); // blocking wait for the result
System.out.println(result);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}
}
In this example, supplyAsync
is used to create a CompletableFuture
that executes asynchronously. The get()
method waits for the future's completion and returns the result.
@RestController
public class MyController {
@GetMapping("/async")
public Mono myMethod() {
return Mono.just("Hello World").delayElement(Duration.ofSeconds(2));
}
}
In this example, Mono
is used to create a reactive stream that executes asynchronously.
When choosing an approach, consider factors such as:
Complexity of the task: Simple tasks might be suitable forExecutorService
, while complex tasks might require more advanced asynchronous programming techniques. Performance requirements: If you need high-throughput and low-latency, you may want to consider using a dedicated thread pool or Webflux's reactive model. Code readability and maintainability: Choose an approach that aligns with your coding style and team's expertise.
Remember to properly handle errors and exceptions in your asynchronous code, as they can be tricky to debug.
What is the best way to do async in Java?
In Java, there are several ways to achieve asynchronous programming, which allows your program to perform multiple tasks concurrently and improve its responsiveness and scalability.
1. Using Threads
The most basic approach to doing async in Java is by using threads. You can create a new thread for each task you want to perform asynchronously, and then start them separately. This way, the main thread (also known as the UI thread) won't be blocked while waiting for a long-running operation to complete.
Here's an example:
public class AsyncExample {
public static void main(String[] args) throws InterruptedException {
Thread t1 = new Thread(() -> {
try {
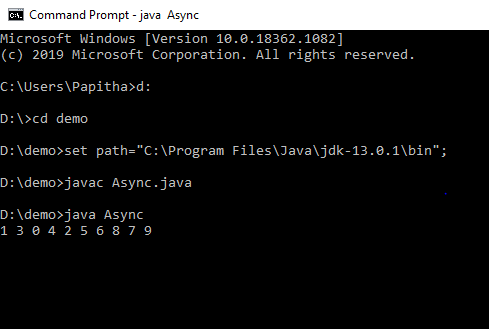
// long-running task 1
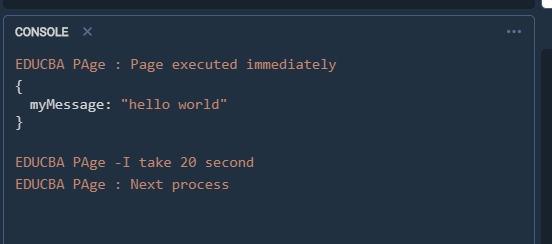
Thread.sleep(5000);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
});
Thread t2 = new Thread(() -> {
try {
// long-running task 2
Thread.sleep(3000);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
});
t1.start();
t2.start();
// do something else while the tasks are running
}
}
However, this approach has its limitations. For example, it can be difficult to manage multiple threads, and you need to ensure that all threads follow the same concurrency rules (e.g., accessing shared resources).
2. Using Futures
Java 7 introduced the java.util.concurrent.Futures
API, which provides a higher-level abstraction for working with asynchronous tasks. A Future
represents the result of an asynchronously executed computation.
Here's an example:
public class AsyncExample {
public static void main(String[] args) throws InterruptedException {
ExecutorService executor = Executors.newFixedThreadPool(2);
Future future1 = executor.submit(() -> {
try {
// long-running task 1
Thread.sleep(5000);
return 1;
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
return null;
}
});
Future future2 = executor.submit(() -> {
try {
// long-running task 2
Thread.sleep(3000);
return 2;
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
return null;
}
});
// do something else while the tasks are running
int result1 = future1.get(); // wait for task 1 to complete and get its result
int result2 = future2.get(); // wait for task 2 to complete and get its result
}
}
The Future
API provides a way to retrieve the results of asynchronous tasks, which can be useful when you need to perform some subsequent processing based on the outcome of those tasks.
3. Using RxJava
RxJava is a Java implementation of Reactive Extensions, which allows you to handle asynchronous data streams using observable sequences.
Here's an example:
import io.reactivex.Observable;
public class AsyncExample {
public static void main(String[] args) {
Observable observable1 = Observable.just(1)
.doOnNext(i -> {
try {
// long-running task 1
Thread.sleep(5000);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
});
Observable observable2 = Observable.just(2)
.doOnNext(i -> {
try {
// long-running task 2
Thread.sleep(3000);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
});
// subscribe to the observables and handle their results
observable1.subscribe(result -> System.out.println("Result 1: " + result));
observable2.subscribe(result -> System.out.println("Result 2: " + result));
}
}
RxJava provides a declarative way of handling asynchronous tasks, allowing you to focus on what you want to do with the results rather than how to get them.
4. Using Java 8's CompletableFuture
Java 8 introduced the java.util.concurrent.CompletableFuture
API, which is designed to simplify working with asynchronous computations.
Here's an example:
public class AsyncExample {
public static void main(String[] args) {
CompletableFuture future1 = CompletableFuture.runAfter(() -> {
try {
// long-running task 1
Thread.sleep(5000);
return 1;
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
return null;
}
}, Duration.ofSeconds(5));
CompletableFuture future2 = CompletableFuture.runAfter(() -> {
try {
// long-running task 2
Thread.sleep(3000);
return 2;
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
return null;
}
}, Duration.ofSeconds(3));
// do something else while the tasks are running
int result1 = future1.get(); // wait for task 1 to complete and get its result
int result2 = future2.get(); // wait for task 2 to complete and get its result
}
}
The CompletableFuture
API provides a way to create asynchronous computations that can be composed together, allowing you to build complex workflows from simple tasks.
In conclusion, there are several ways to achieve asynchronous programming in Java, each with its own strengths and weaknesses. The choice of approach depends on the specific requirements of your project and personal preference.