Can we inherit encapsulation in Java?
Can we inherit encapsulation in Java?
I'd be happy to explain inheritance and encapsulation in Java for you.
Inheritance is a fundamental concept in object-oriented programming (OOP) that allows one class to inherit the properties and behavior of another class. In Java, this is achieved through the extends
keyword followed by the superclass's name.
Here's an example:
public class Animal {
public void eat() {
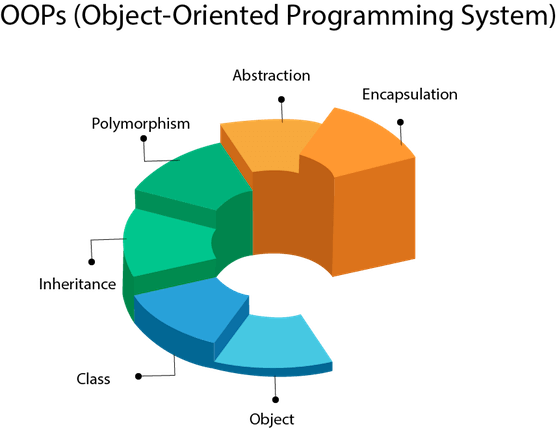
System.out.println("Eating...");
}
}
public class Dog extends Animal {
public void bark() {
System.out.println("Woof!");
}
}
In this example, Dog
is a subclass of Animal
. By inheriting from Animal
, the Dog
class automatically gets access to the eat()
method. The bark()
method is specific to the Dog
class and not present in the superclass.
Now, let's talk about encapsulation. Encapsulation is the idea of bundling data (attributes) with its own methods that operate on that data. This helps to hide the implementation details from the outside world, making it harder for other parts of the program to accidentally modify or misuse the internal state of an object.
In Java, encapsulation is achieved through the use of accessor and mutator methods, also known as getters and setters. These methods allow controlled access to the internal state of an object.
Here's an example:
public class BankAccount {
private int balance;
public BankAccount(int initialBalance) {
this.balance = initialBalance;
}
public void deposit(int amount) {
this.balance += amount;
}
public int getBalance() {
return this.balance;
}
}
In this example, the BankAccount
class has a private attribute called balance
. The constructor sets the initial balance. The deposit()
method allows controlled modifications to the internal state (the balance). The getBalance()
method provides read-only access to the balance.
You can create an instance of BankAccount
, deposit some money, and then get the updated balance without directly accessing or modifying the private attribute:
public class Main {
public static void main(String[] args) {
BankAccount account = new BankAccount(100);
account.deposit(50);
System.out.println("Balance: " + account.getBalance());
}
}
In summary, Java does support inheritance, which allows classes to inherit properties and behavior from other classes. Encapsulation is also supported through the use of accessor and mutator methods, helping to hide the internal implementation details and ensure controlled access to an object's state.
Now, I know what you're thinking: "Can we inherit encapsulation?" Well, not exactly. Inheritance allows us to create a new class that inherits properties and behavior from another class, but it doesn't automatically wrap those properties with accessor and mutator methods. We still need to implement encapsulation explicitly in the subclass.
In the Dog
example I provided earlier, if we wanted to encapsulate its attributes (e.g., age, breed), we would need to create getter and setter methods within the Dog
class itself:
public class Dog extends Animal {
private int age;
private String breed;
public Dog(int initialAge, String initialBreed) {
this.age = initialAge;
this.breed = initialBreed;
}
public void setAge(int newAge) {
this.age = newAge;
}
public int getAge() {
return this.age;
}
public void setBreed(String newBreed) {
this.breed = newBreed;
}
public String getBreed() {
return this.breed;
}
}
I hope that clears up any confusion. Do you have any more questions about inheritance and encapsulation in Java?
abstraction in java
I'm happy to help!
In Java, abstract classes and interfaces are used for abstraction, which is a fundamental concept in object-oriented programming (OOP). Abstraction is the process of exposing only essential features of an object or system while hiding its implementation details.
What is Abstraction in OOP?
Abstraction is about showing only the necessary information to the outside world while keeping the internal implementation private. It's like building a car, where you can see the shape and functionality of the car, but not the intricate mechanical parts underneath. In software development, abstraction helps to:
Hide Complexity: By hiding implementation details, you make your code easier to understand and maintain. Focus on Essentials: Abstraction allows you to focus on the essential features of an object or system, without worrying about unnecessary details. Promote Modularity: Abstract classes and interfaces enable modularity by allowing you to define a common interface for related objects.Java Abstract Classes
In Java, an abstract class is a class that cannot be instantiated directly. It serves as a blueprint for other classes, which can extend the abstract class to provide concrete implementations. An abstract class can:
Define Abstract Methods: Declare methods without providing implementation. Provide Default Implementation: Implement some methods with default behavior.A concrete subclass must implement all abstract methods of its superclass or declare itself abstract as well.
Example: Animal Abstract Class
public abstract class Animal {
public abstract void makeSound();
}
public class Dog extends Animal {
@Override
public void makeSound() {
System.out.println("Woof!");
}
}
public class Cat extends Animal {
@Override
public void makeSound() {
System.out.println("Meow!");
}
}
In this example, the Animal
abstract class defines an abstract method makeSound()
. The Dog
and Cat
classes extend Animal
and provide their own implementations for the makeSound()
method.
Java Interfaces
A Java interface is a contract that specifies a set of methods without providing any implementation. A class can implement multiple interfaces, but it must provide implementations for all abstract methods in each interface.
Example: Printable Interface
public interface Printable {
void print();
}
public class Document implements Printable {
@Override
public void print() {
System.out.println("Printing a document...");
}
}
In this example, the Printable
interface defines an abstract method print()
. The Document
class implements the Printable
interface and provides its own implementation for the print()
method.
In summary, abstraction in Java is achieved through abstract classes or interfaces. Abstract classes define a blueprint with some default implementation, while interfaces specify a contract with no implementation. Abstraction helps to hide complexity, focus on essentials, and promote modularity in your code.