Java copy parent object to child
Java copy parent object to child
Here's an explanation of how to copy a parent object to a child object using Java:
In Java, objects are instances of classes that inherit properties and behavior from their parent or superclass. When you want to create a new object that inherits the characteristics of another object, you can use inheritance to define a subclass (also known as a derived class) that extends its parent class.
To copy a parent object to a child object in Java, you need to understand the concept of deep and shallow copying. Shallow copying involves copying the reference of the original object to the new object, whereas deep copying involves creating an exact clone of the original object by recursively copying all its fields or properties.
Here's a simple example of how to copy a parent object to a child object using Java:
// Define a Parent class with private fields
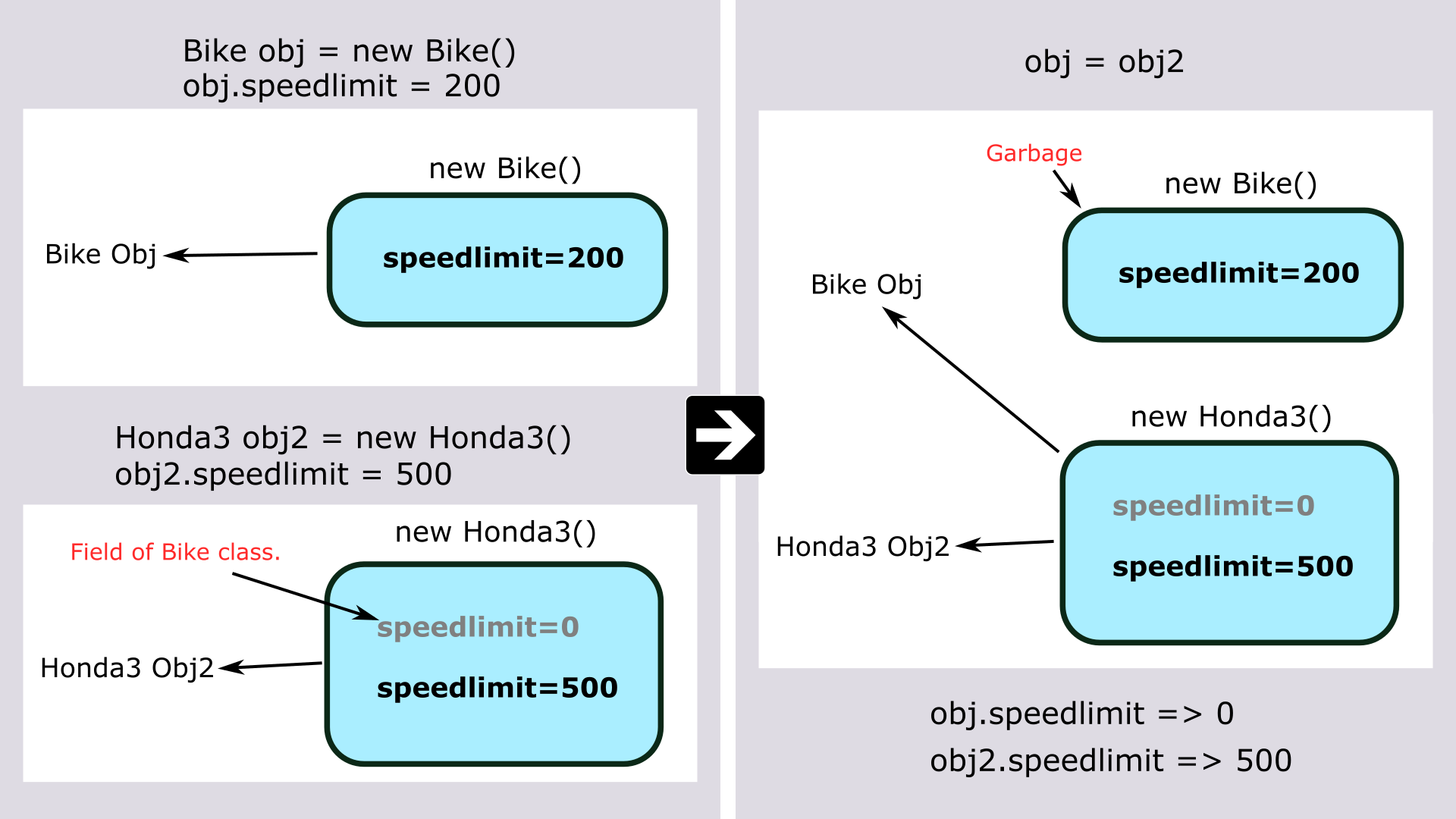
class Parent {
private int id;
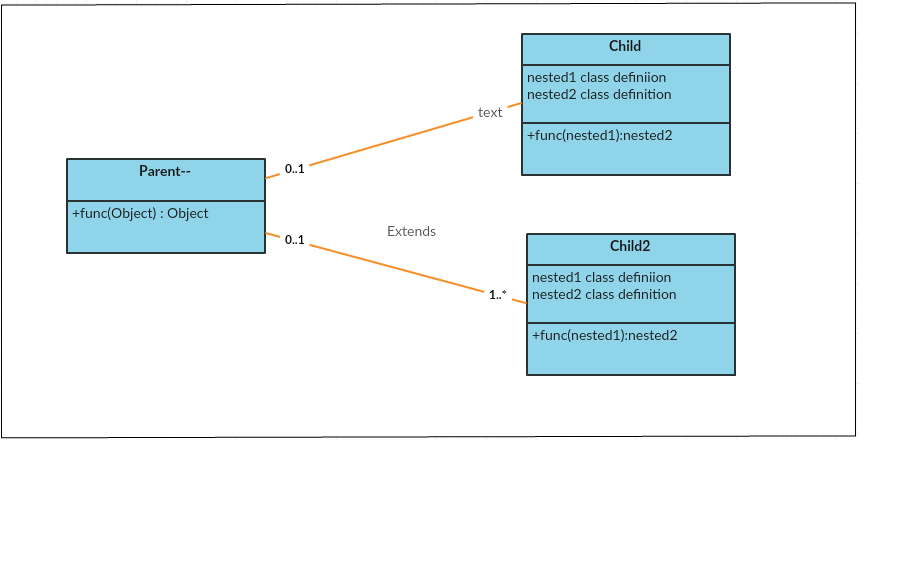
private String name;
public Parent(int id, String name) {
this.id = id;
this.name = name;
}
public int getId() {
return id;
}
public String getName() {
return name;
}
}
// Define a Child class that extends the Parent class
class Child extends Parent {
private int age;
public Child(int id, String name, int age) {
super(id, name);
this.age = age;
}
public int getAge() {
return age;
}
}
// Create a parent object and copy its values to the child object using deep copying
public class Main {
public static void main(String[] args) {
Parent parent = new Parent(1, "John");
Child child = new Child(parent.getId(), parent.getName(), 25);
System.out.println("Parent ID: " + parent.getId());
System.out.println("Parent Name: " + parent.getName());
System.out.println("Child ID: " + child.getId());
System.out.println("Child Name: " + child.getName());
System.out.println("Child Age: " + child.getAge());
}
}
In this example, the Parent
class has two private fields (id
and name
) that are accessed through public getter methods. The Child
class extends the Parent
class with an additional private field (age
). The constructor of the Child
class takes three arguments: the parent's id
, the parent's name
, and the child's age
.
To copy the values from the parent object to the child object, you can create a new Child
object and pass its constructor the same values as those used to create the parent object. This is known as shallow copying because you're only copying the references of the original fields, not their actual values.
However, this approach won't work if you need to preserve the exact state of the parent object, including any nested objects or arrays that it may contain. For example, if the Parent
class had a field that was an array of strings, shallow copying would only copy the reference to the original array, not the actual values themselves.
To achieve deep copying in Java, you can implement the clone()
method on your parent class and override it in your child class. This allows you to create a new object that is an exact clone of the original object by recursively copying all its fields or properties.
Here's how you can modify the previous example to use deep copying:
// Define a Parent class with private fields and implement the Cloneable interface
class Parent implements Cloneable {
private int id;
private String name;
public Parent(int id, String name) {
this.id = id;
this.name = name;
}
public int getId() {
return id;
}
public String getName() {
return name;
}
@Override
public Object clone() throws CloneNotSupportedException {
return new Parent(id, name);
}
}
// Define a Child class that extends the Parent class and overrides the clone method
class Child extends Parent implements Cloneable {
private int age;
public Child(int id, String name, int age) {
super(id, name);
this.age = age;
}
public int getAge() {
return age;
}
@Override
public Object clone() throws CloneNotSupportedException {
return new Child(super.getId(), super.getName(), age);
}
}
// Create a parent object and copy its values to the child object using deep copying
public class Main {
public static void main(String[] args) {
Parent parent = new Parent(1, "John");
Child child = (Child) parent.clone();
System.out.println("Parent ID: " + parent.getId());
System.out.println("Parent Name: " + parent.getName());
System.out.println("Child ID: " + child.getId());
System.out.println("Child Name: " + child.getName());
System.out.println("Child Age: " + child.getAge());
}
}
In this updated example, the Parent
class implements the Cloneable
interface and provides a clone()
method that returns a new instance of itself with the same values. The Child
class also implements the Cloneable
interface and overrides the clone()
method to create a new child object with the same values as the original parent object.
By using deep copying, you can preserve the exact state of the parent object, including any nested objects or arrays that it may contain.
Parent and child class in Java example
I'm happy to help you with that! Here's an example of a parent-child class relationship in Java:
Let's say we want to create a program that can calculate the area and perimeter of different shapes (such as rectangles, circles, triangles). We can do this by defining a parent class called "Shape" that contains common methods for calculating these attributes. Then, we'll create child classes for each specific shape that will inherit the common methods from the parent class.
Here's the code:
// Parent class: Shape
public class Shape {
private double width;
private double height;
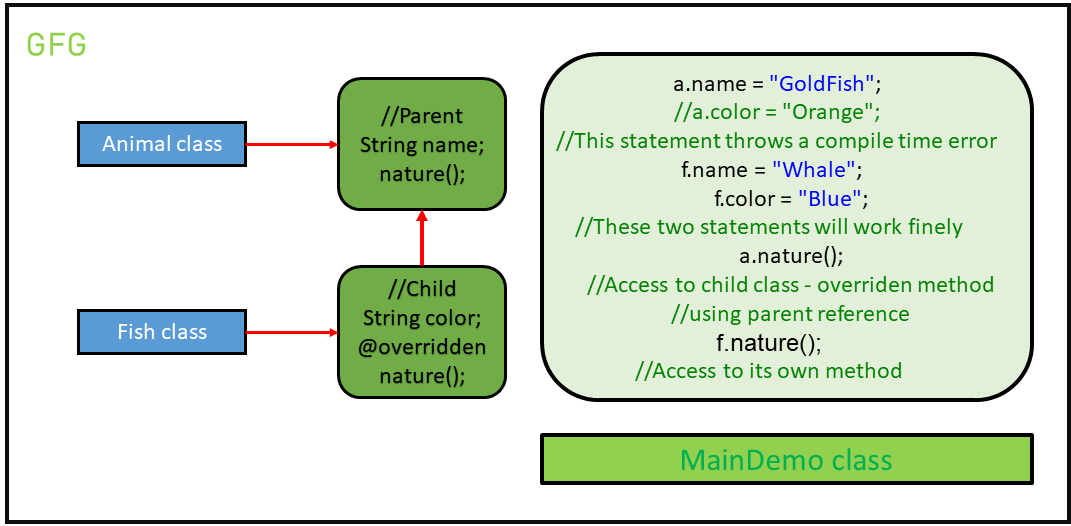
public Shape(double width, double height) {
this.width = width;
this.height = height;
}
public double getWidth() {
return width;
}
public double getHeight() {
return height;
}
// Abstract method that will be implemented by child classes
public abstract double calculateArea();
public abstract double calculatePerimeter();
}
// Child class: Rectangle
public class Rectangle extends Shape {
public Rectangle(double width, double height) {
super(width, height);
}
@Override
public double calculateArea() {
return getWidth() * getHeight();
}
@Override
public double calculatePerimeter() {
return 2 * (getWidth() + getHeight());
}
}
// Child class: Circle
public class Circle extends Shape {
private double radius;
public Circle(double radius) {
super(radius, 0); // Set height to zero for a circle
this.radius = radius;
}
@Override
public double calculateArea() {
return Math.PI * radius * radius;
}
@Override
public double calculatePerimeter() {
return 2 * Math.PI * radius;
}
}
// Child class: Triangle
public class Triangle extends Shape {
private double base;
private double height;
public Triangle(double base, double height) {
super(base, height);
}
@Override
public double calculateArea() {
return 0.5 * getWidth() * getHeight();
}
@Override
public double calculatePerimeter() {
// Since perimeter is not directly applicable to triangles,
// let's just return the sum of the base and two sides (hypotenuse)
return getWidth() + getHeight() + Math.sqrt(getWidth() * getWidth() + getHeight() * getHeight());
}
}
In this example, we have a parent class "Shape" that defines common attributes like width and height, as well as abstract methods for calculating the area and perimeter. The child classes "Rectangle", "Circle", and "Triangle" inherit from the Shape class and implement the abstract methods using specific calculations for each shape.
We can then create objects of these shapes and call the calculateArea() and calculatePerimeter() methods to get the desired results:
public static void main(String[] args) {
Rectangle rect = new Rectangle(4, 5);
Circle circle = new Circle(3);
Triangle tri = new Triangle(3, 4);
System.out.println("Rectangle Area: " + rect.calculateArea());
System.out.println("Circle Perimeter: " + circle.calculatePerimeter());
System.out.println("Triangle Area: " + tri.calculateArea());
System.out.println("Triangle Perimeter: " + tri.calculatePerimeter());
}
This demonstrates the benefits of using inheritance and polymorphism in Java, where we can create a hierarchy of classes that share common attributes and methods, making our code more reusable and maintainable!