Conditional statements in java with example interview questions
Conditional statements in java with example interview questions
I'm happy to help! Here are some conditional statement examples in Java with interview questions:
If-else Statement
The if-else statement is used to execute a block of code if a condition is true or false.
Example:
int x = 10;
if (x > 5) {
System.out.println("x is greater than 5");
} else {
System.out.println("x is less than or equal to 5");
}
Interview Question: Write an if-else statement that checks if a person's age is greater than 18, and if so, prints "You are an adult." Otherwise, it prints "You are a minor."
Switch Statement
The switch statement is used to execute a block of code based on the value of a variable.
Example:
int dayOfWeek = 2;
switch (dayOfWeek) {
case 1:
System.out.println("Monday");
break;
case 2:
System.out.println("Tuesday");
break;
default:
System.out.println("Unknown day of the week");
break;
}
Interview Question: Write a switch statement that takes a grade as an input (A, B, C, D, or F) and prints out the corresponding letter grade.
If-else-if Statement
The if-else-if statement is used to execute multiple blocks of code based on multiple conditions.
Example:
int score = 80;
if (score >= 90) {
System.out.println("A grade");
} else if (score >= 80) {
System.out.println("B grade");
} else {
System.out.println("C grade or lower");
}
Interview Question: Write an if-else-if statement that checks if a person's score is greater than or equal to 90, and if so, prints "A grade". If not, it checks if the score is greater than or equal to 80, and if so, prints "B grade". Otherwise, it prints "C grade or lower".
Ternary Operator
The ternary operator is a shorthand way of writing an if-else statement.
Example:
int x = 10;
String result = (x > 5) ? "Greater than 5" : "Less than or equal to 5";
System.out.println(result);
Interview Question: Write a ternary operator that takes a person's age as an input and prints out "Adult" if the age is greater than 18, otherwise it prints "Minor".
Nested Conditions
The nested conditions are used when you have multiple conditions inside another condition.
Example:
int x = 10;
if (x > 5) {
if (x < 15) {
System.out.println("x is between 6 and 14");
} else {
System.out.println("x is greater than or equal to 15");
}
}
Interview Question: Write a nested condition that checks if a person's age is greater than 18, and if so, it checks if the age is less than 25. If both conditions are true, it prints "Young adult". Otherwise, it prints "Older".
I hope this helps!
What is a conditional statement in Java with an example?
I'm happy to help you with your question!
A conditional statement in Java, also known as an if-else statement or a decision-making statement, is used to make decisions based on conditions or criteria. It allows the program to execute different blocks of code depending on whether a condition is true or false.
The basic syntax for a conditional statement in Java is:
if (condition) {
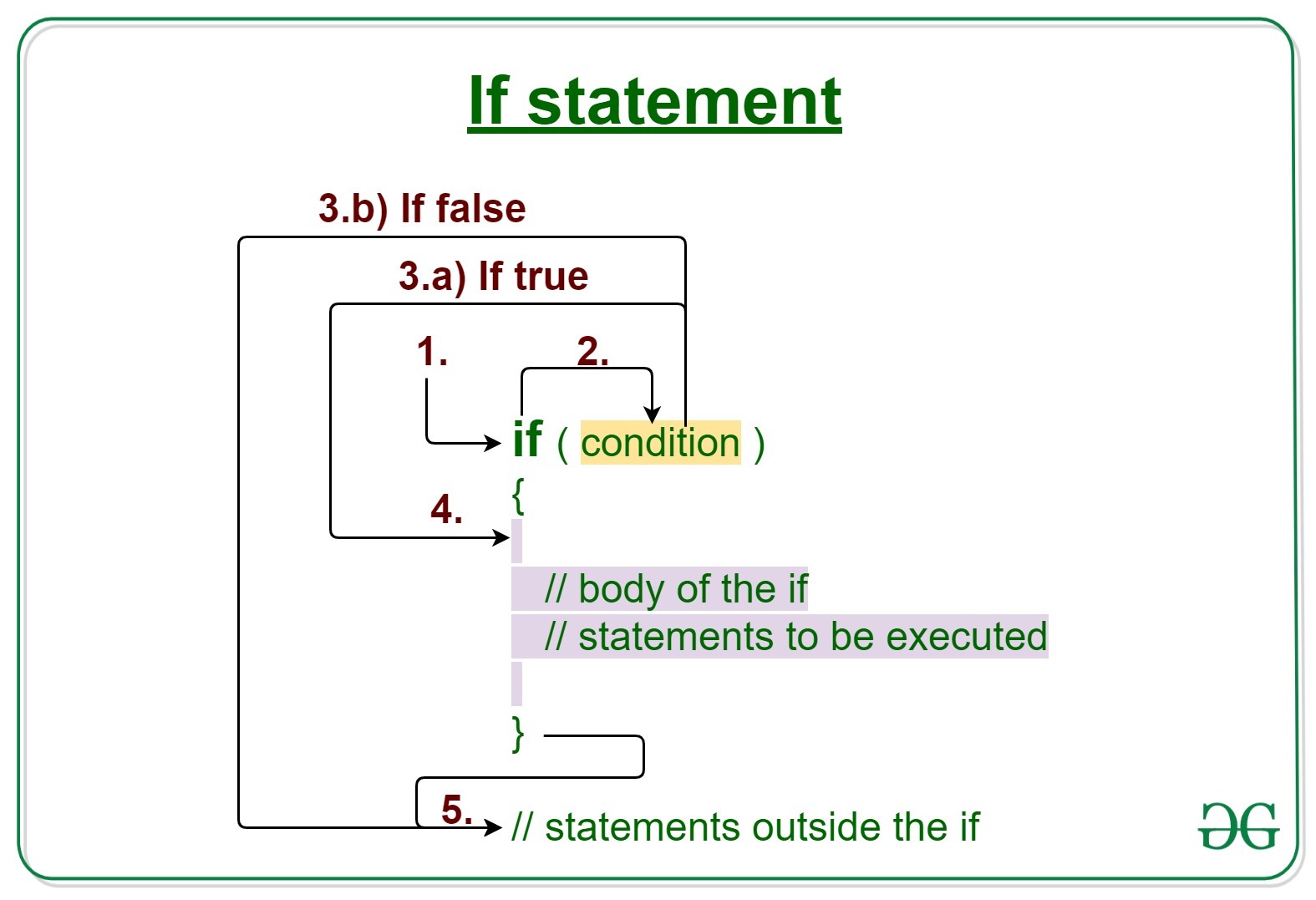
// code to be executed if condition is true
} else {
// code to be executed if condition is false
}
Here, condition
is a boolean expression that evaluates to either true
or false
. If the condition is true
, the code inside the if block will be executed. Otherwise, the code inside the else block will be executed.
Let's consider an example:
int age = 25;
if (age >= 18) {
System.out.println("You are eligible to vote.");
} else {
System.out.println("You are not yet eligible to vote.");
}
In this example, we have a variable age
with value 25. We then use an if-else statement to check whether the age is greater than or equal to 18. If it is, we print "You are eligible to vote." Otherwise, we print "You are not yet eligible to vote."
When we run this program, since the condition age >= 18
is true (25 is indeed greater than or equal to 18), the output will be:
You are eligible to vote.
Conditional statements can also have multiple conditions using the else if statement. For example:
int score = 80;
if (score >= 90) {
System.out.println("A");
} else if (score >= 80) {
System.out.println("B");
} else {
System.out.println("F");
}
In this case, we have a variable score
with value 80. We use an if-else statement to check the score and print out a grade accordingly. Since 80 is greater than or equal to 80 but less than 90, the output will be:
B
Conditional statements are a fundamental part of programming in Java (and many other languages). They allow you to make decisions based on conditions and execute different blocks of code depending on those conditions.
Hope this helps! Let me know if you have any further questions.