Java catch Throwable
Java catch Throwable
I'd be happy to help!
The try
and catch
blocks are used to handle exceptions in Java. An exception is an event that occurs during the execution of a program, such as an arithmetic error or a file not found error.
In general, you should catch only those exceptions that your code can reasonably recover from. If your code cannot recover from an exception, it's best to let it propagate up the call stack and be caught by a higher-level try
block (or possibly terminate the program if no try
blocks are present).
Here's an example of using Java's catch
statement with Throwable
, which is the superclass of all exceptions:
public class CatchThrowableExample {
public static void main(String[] args) {
try {
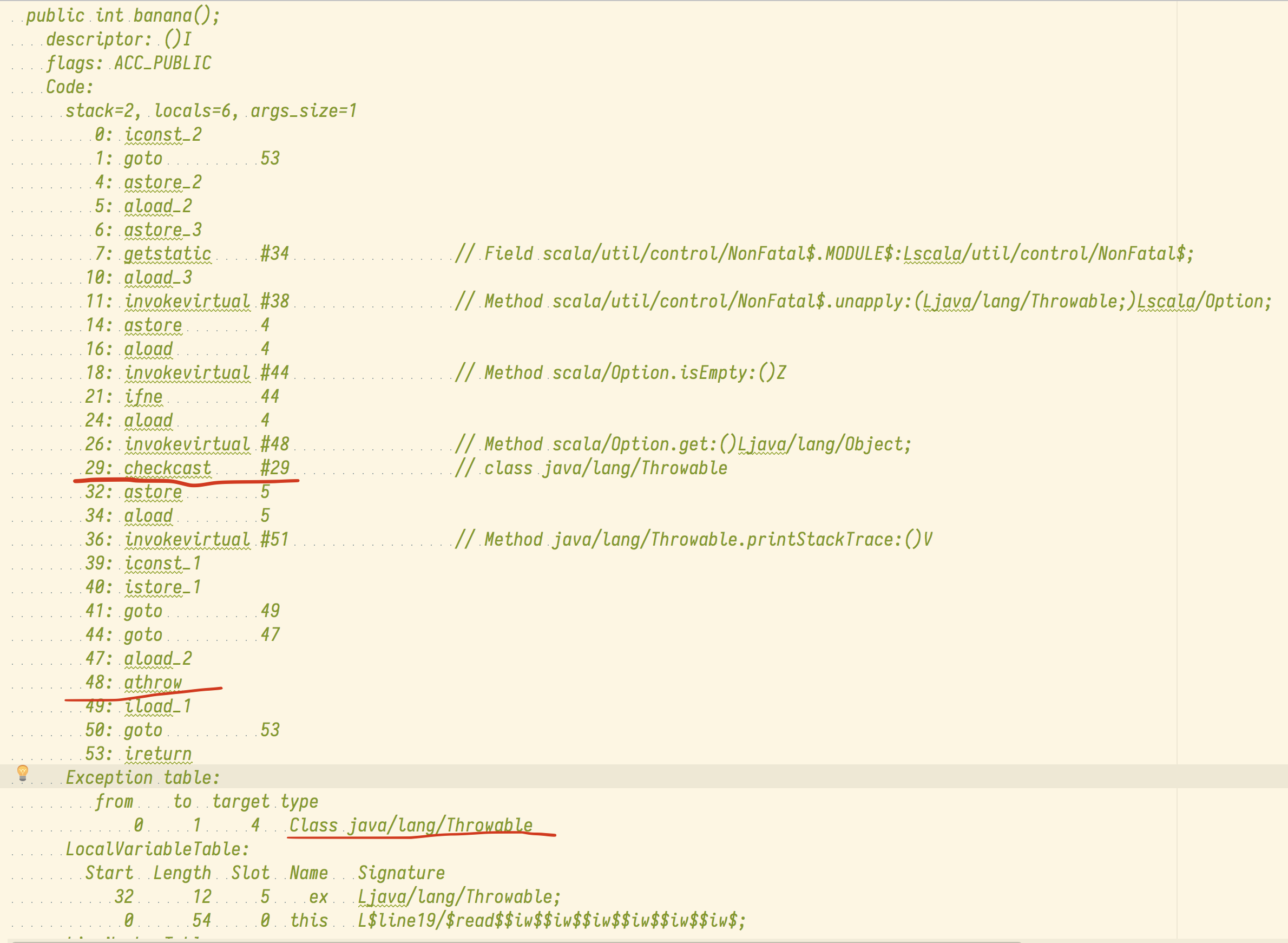
// Code that might throw an exception
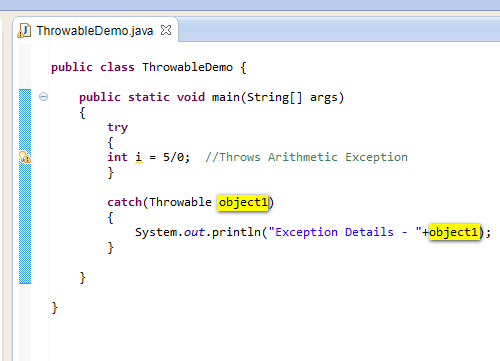
int i = 1 / 0; // This will throw an ArithmeticException
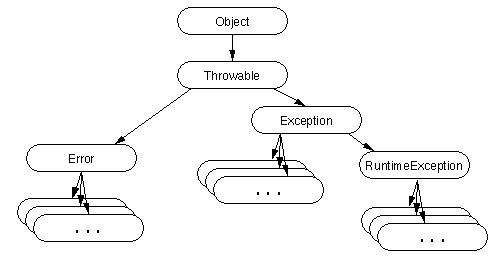
} catch (Throwable t) {
System.out.println("Caught a Throwable!");
t.printStackTrace();
}
}
}
In this example, the try
block contains code that may throw an exception. If it does, the catch
block is executed. In this case, the catch
block catches all types of exceptions (because it's catching Throwable
, which includes Exception
and its subclasses) and prints out a message indicating that it caught something, as well as printing out the stack trace for the exception.
Here are some best practices to keep in mind when using Java's catch
statement with Throwable
:
Exception
. If a more specific exception is caught by a higher-level block and you're trying to handle a more general one, that will not be caught. Don't catch Throwable
unless you have to: In general, catching Throwable
should be avoided as it can mask bugs in your code. It's better to catch specific exceptions or let them propagate up the call stack for debugging purposes. Log the exception if you can't recover: If an exception is caught and you can't reasonably recover from it (e.g., you're writing a utility function that doesn't need to be resilient), log the error message and stack trace so that the problem can be tracked down later.
Here's how you might implement these best practices:
public class CatchThrowableExample {
public static void main(String[] args) {
try {
int i = 1 / 0; // This will throw an ArithmeticException
} catch (ArithmeticException e) {
System.out.println("Caught an ArithmeticException!");
e.printStackTrace();
} catch (Exception e) {
System.out.println("Caught a general exception!");
logError(e);
// You can also re-throw the exception if you didn't recover from it
throw e;
}
}
private static void logError(Throwable t) {
// Your logging mechanism here
}
}
In summary, Java's catch
statement with Throwable
is used to catch all types of exceptions in a block. It's important to use this construct judiciously and follow best practices for exception handling: catch specific exceptions first, avoid catching Throwable
, log the error if you can't recover from it, and re-throw the exception if you didn't recover from it.
When to catch throwable in Java?
I'd be happy to help you with that!
In Java, a Throwable
is an object that represents an exceptional condition that has occurred. This can include things like runtime errors (e.g., NullPointerException
), checked exceptions (e.g., IOException
), and unchecked exceptions (e.g., ArithmeticException
).
So, when to catch a throwable in Java? Well, it's generally good practice to catch specific types of Throwable
s that you anticipate might occur in your code. This can help prevent propagation of the exception up the call stack, which can be useful for handling errors in a centralized manner.
Here are some general guidelines on when and how to catch throwables:
Catch specific exceptions: If you know that a particular method or block of code may throw a specific type ofThrowable
(e.g., FileNotFoundException
), you should catch it explicitly using the try-catch
construct. This is useful when you want to handle that specific error in a unique way.
try {
File file = new File("somefile.txt");
// ...
} catch (FileNotFoundException e) {
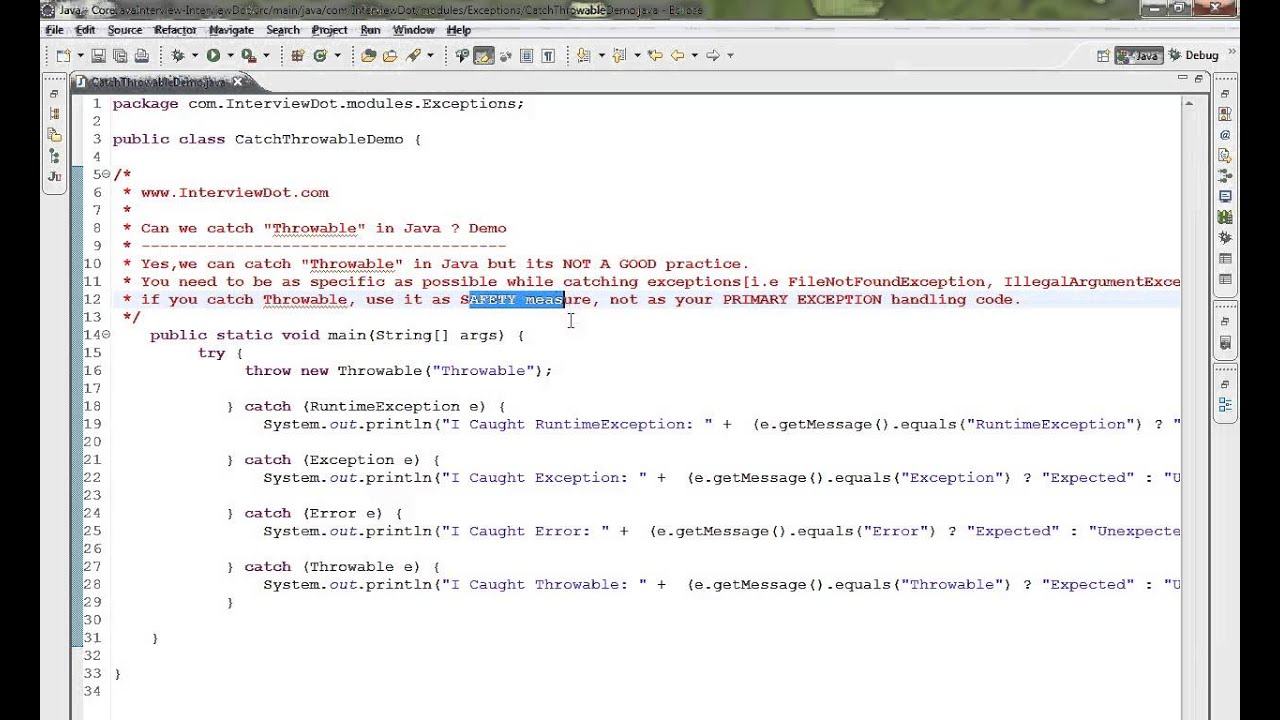
System.out.println("File not found!");
}
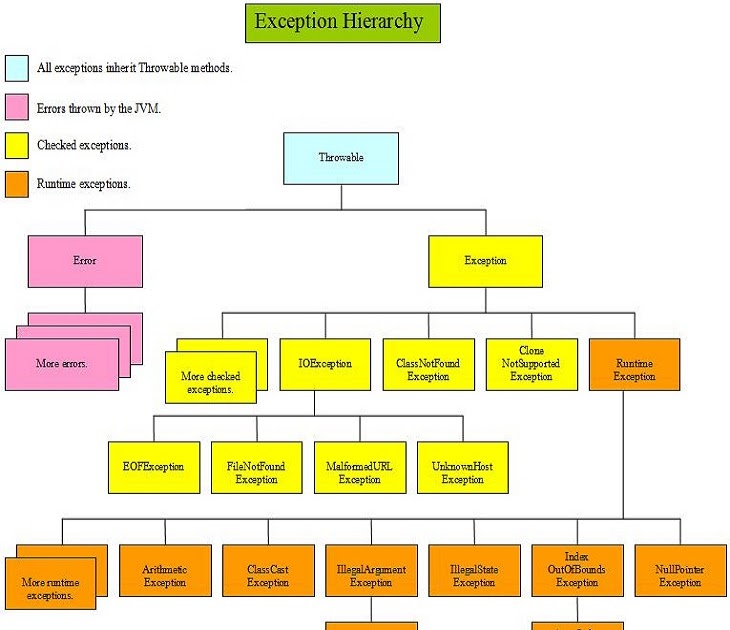
Throwable
that might occur, you can catch a more general exception like Exception
or RuntimeException
. This is useful when you want to provide a default error handling mechanism.
try {
// ...
} catch (Exception e) {
System.out.println("Something went wrong!");
}
Don't catch checked exceptions: In Java, it's generally considered bad practice to catch checked
exceptions like IOException
or SQLException
at the highest level of your program. Instead, you should rethrow them or handle them in a way that provides meaningful feedback to the user.
try {
// ...
} catch (IOException e) {
throw e; // Re-throw the exception
}
Use finally block: Regardless of whether an exception is thrown or not, you should always include a finally
block in your code to ensure that resources are properly released.
try {
// ...
} catch (Exception e) {
// Handle error
} finally {
// Release resources
}
Remember: the goal of exception handling is to provide meaningful feedback to users, prevent propagation of errors, and keep your program running smoothly. So, when in doubt, it's always better to err on the side of caution and catch specific types of Throwable
s that you anticipate might occur!